Question
Need help in c++, not sure what is wrong please help and thank you, the code is below and so is the question. Write a
Need help in c++, not sure what is wrong please help and thank you, the code is below and so is the question.
Write a complete program that performs encryption/decryption of a text file. Encryption means you need to scramble the words of a file to become secure. Decryption means you need to apply some rules to an encrypted file in order to find the original file with its original content. An example of encryption is to replace each letter in a file with its consecutive letter in the alphabet. Therefore, a is replaced by b, b is replaced by c and so on and finally z is replaced by a. Thus, if the file contains the following message:
I am a student at csusm
The encrypted message becomes:
J bn b tuvefou bu dtvtn
Then if you get this encrypted message and the key is 1, it means you need to shift the alphabets in the decrypted message by 1 to the left to get the original message back.
This method is called rot1 indicating that every alphabet rotates by 1 during the encryption. Similarly, rot10 means every alphabet should be rotated by 10 during encryption.
Another method of encryption is to create cryptogram. A cryptogram is a message or word where each letter is replaced with another letter. For example, the string:
The birds name was squawk
might be scrambled to form
xms kbypo zhqs fho obrhfu
Note that space or any punctuation is not scrambled. In this particular case, T was replaced with x, each a was replaced with h. etc. We assume there is no difference between lower case or uppercase. Therefore, you must turn all of the letters of a word into lower case before encrypt it. For example, if your work is McMaster, the function (word = tolower(McMaster)) makes word to be equal to mcmaster.
Your job is to write a driver program(look at the program guide to see how to create a driver program)that performs different types of encryption or description. First, you need to ask the user to see if the user wants to do encryption or decryption. If the user wants to do encryption, you should find out if the user wants to use rot method or cryptogram method. If the user selects the rot method, you need to ask for the encryption key. For example, entering 15, means that the user wants to use rot15 method to do encryption. If the user selects the cryptogram method, you should open the cryptogram text file called CryptoKey where the cryptogram string is stored. The cryptogram string should contain a string of 26 letters where the first letter corresponds to letter a, the second letter corresponds to letter b and so on. For example, your cryptogram file may contain the following message.
uhtxvdepqlzfonkcrwyijsgabm
which means u corresponds to a, h corresponds to b and and finally m corresponds to z.
The same procedure should be followed for decryption. You need to ask the user which method the user wants to use (rot or cryptogram) for encryption. Then write the routines that takes an encrypted file and does the decryption to get the original file back.
You are responsible to use string and vector class in this program. For encryption, you need to read the file to be encrypted into a vector of string and place the words (one word per element) into a vector. Then read the elements of your vector one by one and do the encryption and place the encrypted word into another vector. Finally write the content of encrypted vector to an output file.
For decryption, you need to do the same process which is read the file that contains the encrypted information and place the encrypted words one by one into a vector. Then create another vector so that as you decrypt the information you can place the decrypted words into another vector. Finally, the vector that contains the decrypted words (original message) should be written to an output file.
Here is a message you can do your test:
Psychology is the study of mindand behavior[1][2]. It is an academic disciplineand an applied sciencewhich seeks to understand individuals and groups by establishing general principles and researching specific cases [3][4]. In this field, a professional practitioneror researcher is called a psychologistand can be classified as a social, behavioral, or cognitive scientist. Psychologists attempt to understand the role of mental functionsin individual and social behavior, while also exploring the physiologicaland biologicalprocesses that underlie cognitive functions and behaviors. Psychologists explore concepts such as perception, cognition, attention, emotion, intelligence, phenomenology, motivation, brain functioning, personality, behavior, and interpersonal relationships, including psychological resilience, family resilience, and other areas. Psychologists of diverse orientations also consider the unconscious mind. [5]Psychologists employ empirical methodsto infer causaland correlationalrelationships between psychosocial variables. In addition, or in opposition, to employing empiricaland deductivemethods, some especially clinicaland counselingpsychologists at times rely upon symbolic interpretationand other inductivetechniques. Psychology has been described as a "hub science", [6]with psychological findings linking to research and perspectives from the social sciences, natural sciences, medicine, humanities, and philosophy
Place the above message in a text file called Original.txt and test your program based on the following:
Execute your program to run the driver routine. Then do the following:
Encrypt the original message using rot21 (shifting every alphabet to the right 21) and call the file EncryptRot.txt
Encrypt the original message using the following cryptogram and call the file EncryptCrypto.txt
yihtqkcdplzuvwmxsgajbrfeon
Decrypt EncryptRot.txt file using rot21 (shifting every alphabet to the left 21) places and call it DecryptRot.txt
Decrypt EncryptCypto.txt file using the above cryptogram and call it DecryptCrypto.txt
Your DecryptRot.txt and DecryptCrypto.txt should be similar (not exactly identical) to your Original.txt file you used for encryption. For example, in the decrypted files all words are in lower case but it is not necessarily the case in the Original.txt file.
The code is below
#include
#include
#include
#include
using namespace std;
class EncDec //Class for encryption and decryption
{
private:
string keyFile; //Data member to store cryptogram key.
vector
public:
EncDec(){};
string filetoString(string filename); //Method to open a file and copy into a string.
vector
void vectortoFile(const vector
void displayVector(const vector
void EncryptROT(); //Encrypts data using the ROT method.
void DecryptROT(); //Decrypts data using the ROT method.
void EncryptCrypto(); //Encrypts data using the cryptogram method.
void DecryptCrypto(); //Decrypts data using the cryptogram method.
};
//Method implementation.
string EncDec::filetoString(string filename)
{
ifstream fin;
fin.open(filename.data()); //Open a file and save it to a string.
string word;
if(!fin)
{
cout << " The file could not be opened."
<< " Please retry."
<< endl;
exit(1);
}
else
{
while(!fin.eof()) //Data is saved to string keyFile until the end of file.
keyFile.push_back(fin.get());
fin.close();
}
return keyFile;
}
vector
{
ifstream fin;
fin.open(filename.data()); //Open a file and save it to a vector.
string word;
if(!fin)
{
cout << " The file could not be opened."
<< " Please retry."
<< endl;
exit(1);
}
else
{
while(fin>>word) //Data is saved to the vector until the end of file.
originalFile.push_back(word);
fin.close();
}
return originalFile;
}
void EncDec::vectortoFile(const vector
{
ofstream fout;
fout.open(filename.data()); //Read a vector and save it to a file.
string word;
if(!fout)
{
cout << " The file could not be opened."
<< " Please retry."
<< endl;
exit(1);
}
else
{
for(int i=0; i { word = V[i]; fout << word << " "; } fout.close(); } return; } void EncDec::displayVector(const vector { for(int i=0; i cout << V[i] <<" "; cout << endl; } void EncDec::EncryptROT() //Encrypt using the ROT method. { //Declare and initialize variables. int rot = 0; string filename = "Original.txt"; string save = "EncryptRot.txt"; cout << "\tEnter ROT number: "; cin >> rot; cout << endl; vector vector //Loop to read a single element and store it into string word. for(int i=0; i { string word = V[i]; for(int j=0; j { int value = word[j]; if(value>96 && value<123) //Encrypt lowercase. { value += rot; if(value>122) word[j] = value - 26; else word[j] = value; } else if(value>64 && value<91) //Encrypt uppercase. { value += rot; if(value>90) word[j] = value - 26; else word[j] = value; } else //If value is not a letter, then skip. { word[j] = value; } } string encryptWord = word; //Store encrypted word in string encryptWord. encVec.push_back(encryptWord); //Store string encryptWord in vector encVec. } vectortoFile(encVec, save); //Save encVec to file "encrypt01.txt". displayVector(encVec); //Output vector decVec for confirmation. return; } void EncDec::DecryptROT() //Decrypt using the ROT method. { //Declare and initialize variables. int rot = 0; string filename = "EncryptRot.txt"; string save = "DecryptRot.txt"; cout << "\tEnter ROT number: "; //Ask the user for input. cin >> rot; cout << endl; vector vector //Loop to read a single element and store it into string word. for(int i=0; i { string word = V[i]; for(int j=0; j { int value = word[j]; if(value>96 && value<123) //Decrypt lowercase. { value -= rot; if(value<97) word[j] = value + 26; else word[j] = value; } else if(value>64 && value<91) //Decrypt uppercase. { value -= rot; if(value<65) word[j] = value + 26; else word[j] = value; } else //If value is not a letter, then skip. { word[j] = value; } } string decryptWord = word; //Store decrypted word in string decryptWord. decVec.push_back(decryptWord); //Store string decryptWord in vector decVec. } vectortoFile(decVec, save); //Save decVec to file "decrypt01.txt". displayVector(decVec); //Output vector decVec for confirmation. return; } void EncDec::EncryptCrypto() //Encrypt using the cryptogram method. { cout << endl; //Declare and initialize variables. string filename = "Original.txt"; string key = "key.txt"; string save = "EncryptCrypto.txt"; string key2 = filetoString(key); //Load the cryptogram into string key2. vector vector //Loop to read a single element and store it into string word. for(int i=0; i { string word = V[i]; for(int j=0; j { int value = word[j]; if(value>96 && value<123) //Encrypt lowercase. { char value2 = key2.at(value - 97); word[j] = value2; } else if(value>64 && value<91) //Encrypt uppercase. { char value2 = key2.at(value - 65); word[j] = value2 - 32; } else //If value is not a letter, then skip. { word[j] = value; } } string encryptWord = word; //Store encrypted word in string encryptWord. encVec.push_back(encryptWord); //Store string encryptWord in vector encVec. } vectortoFile(encVec, save);//Save encVec to file "encrypt02.txt". displayVector(encVec); //Output vector decVec for confirmation. return; } void EncDec::DecryptCrypto() //Decrypt using the cryptogram method. { cout << endl; //Declare and initialize variables. string filename = "EncryptCrypto.txt"; string key = "key.txt"; string save = "DecryptCrypto.txt"; string key2 = filetoString(key); //Load the cryptogram into string key2. //Load a file into vector V and save it to vector decVec. vector vector //Loop to read a single element and store it into string word. for(int i=0; i { string word = V[i]; for(int j=0; j { int value = word[j]; if(value>96 && value<123) //Decrypt lowercase. { char value = word[j]; int value2 = key2.find(value, 0); word[j] = value2 + 97; } else if(value>64 && value<91) //Decrypt uppercase. { char value = word[j] + 32; int value2 = key2.find(value, 0); word[j] = value2 + 65; } else //If value is not a letter, then skip. { word[j] = value; } } string decryptWord = word; //Store decrypted word in string decryptWord. decVec.push_back(decryptWord); //Store string decryptWord in vector decVec. } vectortoFile(decVec, save); //Save decVec to a file. displayVector(decVec); //Output vector decVec for confirmation. return; } //Declare functions. void menuLoop(); void menuEncrypt(); void menuDecrypt(); int main() { menuLoop(); //Begin the program loop cout << " Goodbye. " << endl; return 0; } void menuLoop() { bool exit = false; //Declare a variable for exiting the program. int choice = 0; //Initialize a variable for user input. while(exit!=true) //Begin menu selection loop. { cout << " [1] Encrypt Data" //Display user options. << " [2] Decrypt Data" << " [3] Exit" << " Please enter your selection: "; cin >> choice; //Get user input. switch(choice) //Switch for encryption or decryption. { case 1: //Selection for encrypting data. { menuEncrypt(); break; } case 2: //Selection for decrypting data. { menuDecrypt(); break; } case 3: //Exit program. { exit = true; break; } default: //Display an error message if an integer is not entered. { cout << " Invalid selection, please try again." << endl; break; } } } return; } void menuEncrypt() //Submenu for performing encryptions. { //Instantiate an object. EncDec Encrypt; int choice = 0; cout << " \t[1] Encrypt using ROT method" //Display user options. << " \t[2] Encrypt using Cryptogram method" << " \t[3] Cancel" << " \tPlease enter your selection: "; cin >> choice; //Get user input. switch(choice) //Switch for ROT or Cryptogram encryption method { case 1: //Call the function to encrypt using ROT method. { Encrypt.EncryptROT(); break; } case 2: //Call the function to encrypt using cryptogram method. { Encrypt.EncryptCrypto(); break; } case 3: //Cancel out of the menu. { break; } default: //Display an error message if an integer is not entered. { cout << " Invalid selection, please try again." << endl; break; } } return; } void menuDecrypt() //Submenu for performing decryptions. { //Instantiate an object. EncDec Decrypt; int choice = 0; cout << " \t[1] Decrypt using ROT method" //Display user options. << " \t[2] Decrypt using Cryptogram method" << " \t[3] Cancel" << " \tPlease enter your selection: "; cin >> choice; //Get user input. switch(choice) //Switch for ROT or Cryptogram decryption method. { case 1: //Call the function to decrypt using the ROT method. { Decrypt.DecryptROT(); break; } case 2: //Call the function to decrypt using the cryptogram method. { Decrypt.DecryptCrypto(); break; } case 3: //Cancel out of the menu. { break; } default: //Display an error message if an integer is not entered. { cout << " Invalid selection, please try again." << endl; break; } } return; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
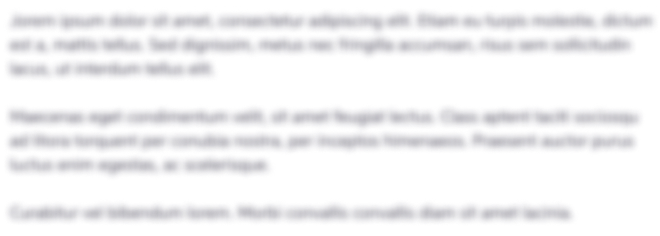
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started