Question
Need help in c program. Thank you so much. Setting up a System Call A system call must be both available in the user space
Need help in c program. Thank you so much.
Setting up a System Call A system call must be both available in the user space and kernel space. It must be available in user space so that it can be used other programs and it must be available in kernel space so has permission to access resources that prohibited from user mode. User Mode Files When we call a system call, we are actually invoking a trap in the kernel. However, we wish to avoid writing assembly code or traps in our programs. So wrappers, written in a high-level language, will wrap around the assembly code which will allow us to use them as functions.
- user.h Listed in this file are all the functions that are available in user space as a wrapper for the system calls. The functions are written in C and it looks very similar to a function prototype. It is important to make sure that the arguments declared with this function matches the arguments when the function is defined (they are defined in sysproc.c which will be discussed later.)
TO DO: Edit user.h to add a line for the time system call.
Hint: Look at how other system calls are declared and follow the pattern that best suits the time system call.
- usys.S
This is an assembly file. This is where the system call is implemented. It is a very simple file and you will see that there is one macro declared:
01 #define SYSCALL(name) \
02 .globl name; \
03 name: \
04 movl $SYS_ ## name, %eax; \
05 int $T_SYSCALL; \
06 ret
A brief walk-through of the macro: Line 01: Macro definition, it takes one argument called name. Line 02: The argument is used to define a global variable with that name. Line 03: A label with the name. Line 04: Then you're going to move something, a number, into the register eax. This indicates what system call you are calling. The number being pushed into eax is the number that was declared in syscall.h that corresponds to the system call. Syscall.h will be discussed later. Line 05: Then you will interrupt the kernel and pass a number, which indicates the corresponding interrupt is a system call. Line 06: Then you will return.
The code the follows after this macro is repeatedly calling the macro for all the different system calls.
TO DO: Edit usys.S to add a line for the time system call. Hint: Look at how other system calls are declared and follow the pattern.
- time.c
This file is the program what will implement the time system call. This in user space and therefore is written in C. Implementing the time system requires you to use other xv6 system calls, in particular uptime, fork, exec, and wait. You can find a detailed explanation of these in Chapter 0 of the xv6 textbook.
You are responsible to create a new file called time.c, and create a program that will:
Accepts arguments from the command line interface. You are expected to do the necessary error checking with the corresponding error message.
Fork a child process, the parent process is responsible to measure the time it takes for the child process to finish executing. o Get the current time using uptime. o Fork and then wait for the child process to terminate. o Then when wait returns in the parent process, get the current time again and calculate the difference.
Return an error message if fork is unsuccessful.
The child process is responsible for executing the command the user wishes to time using the exec command. You must determine how you will pass the arguments from the command line. o The child is responsible to return an error message is execution fails.
TO DO: Create time.c and implement the time system call.
Hint: Code time.c after youve finished making the system call accessible in the kernel and editing the Makefile. This way you can test and debug as you program.
Kernel Mode Files
The files below are where the system call is made available to the kernel.
- syscall.h
This file is the interface between the kernel space and user space. From the user space you invoke a system call and then the kernel can refer to this to know where to find the system call. It is a simple file that defines a system call to a corresponding number, which will be later used as an index for an array of function pointer.
TO DO: Edit syscall.h to add a line for the time system call. Hint: Look at how other system calls are declared and follow the pattern.
- syscall.c This file has two sections where the system call must be added. Starting at line 80, is a portion where all the functions for the system calls are defined within the kernel.
TO DO: Edit syscall.c to add a line for the time system call.
Hint: Look at how other system calls are declared and follow the pattern.
The second part is an array of function pointers. A function pointer in C, is when you can pass a name of a function as an argument to another function. The array is indexed using the values defined in syscall.h. Each element of the array points to a function, which is invoked.
TO DO: Edit syscall.c to add a line in the function pointers array for the time system call. Hint: Look at how other system calls are declared and follow the pattern. Before you add the line for the time system call, notice that the last system call has a comma. That is intentional and you must follow the pattern when you add the time system call.
At the end of the array of function pointers there is the how xv6 implements system calls.
void
syscall(void)
{ int num; num = proc->tf->eax;
if(num > 0 && num < NELEM(syscalls) && syscalls[num])
{ proc->tf->eax = syscalls[num](); }
else { cprintf("%d %s: unknown sys call %d ",
A brief walk-through of the function:
First it gets the number of the system call to from eax.
The number was stored in eax when the macro declared in usys.S got executed.
Then some basic error checking:
Checks if the number is greater than 0;
Checks if the number less than the total number of system call in the system;
Checks if the system call we are calling it not null.
If the system call passes, it call that function and then the function will return a value in eax. In xv6 the convention is to return the value of a function in the eax register.
If it fails, it returns an error and returns a -1 into eax.
- sysproc.c
This is where we are defining all of the function in kernel space. Here you will find how the other system calls are defined. Because the time system call is very simple, it actually does not need to get any information from the process. However, it still needs to be declared in this file.
TO DO: Edit sysproc.c to add a function for the time system call.
Hint: Do not over think this function. It is an empty function and it returns an integer, very similar to an empty main function in C. You may look at the other system call definitions to derive your solution.
Compiling Your New System Call
Change the Makefile to add your time program so that it will be compiled along with the rest of the user programs the when you run the command make qemu.
UPROGS=\
... _stressfs\
_time\ //Add this line
_usertests\
TO DO: Edit Makefile to add the time program under UPROGS (line 159). You only have to do this once for each new user program created.
Testing Time System Call
Once you are done with your program, you can test it and execute make qemu.
Type in a simple ls command and see if the system call is working as intended.
Further instructions
1. If command is time ls, it should display in number of ticks
2. If command is time -s ls, it should display time in seconds (floating point value). (float or double dont work in xv6).
Hint: 100 ticks = 1 second
Note: List of xv6 files that need to be updated to add a system call in this assignment
1. user.h
2. usys.s
3. syscall.h
4. syscall.c
5. sysproc.c
6. makefile
Step by Step Solution
There are 3 Steps involved in it
Step: 1
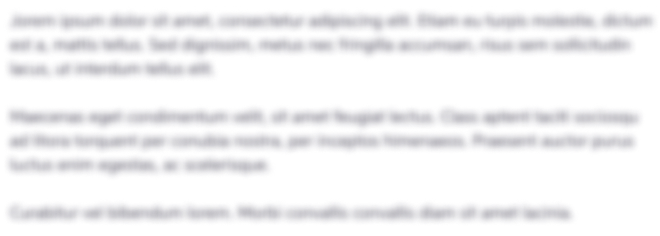
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started