Question
Need help in filling out the rest of the code for the given templates. Pre-lab Tasks: (10 points) Using the program template 1.1 provided, complete
-
- Need help in filling out the rest of the code for the given templates.
-
- Pre-lab Tasks: (10 points)
-
- Using the program template 1.1 provided, complete the program by adding the missing parts using the Arduino IDE that begins incrementing an internal variable, icount after the button is pushed. Your program should increment the variable once every second and should display the count (modulo 10) on the 7-segment display. (3 points)
- Using the program template 1.2 provided on the previous page, complete the program by adding the missing parts using the Arduino IDE that counts the number of times a button is pushed over a 10-second interval. Your program should do the following: (3 points)
- The timed interval starts with a button push.
- The system will count the number of times the button is pushed over a 10 second interval.
- The system should stop the count when the 10 second time interval has expired.
- The program should display all digits (assumed maximum of 2-digit pushes) of the count on the 7-segment LED display. The most significant digit of the count should be displayed first and the next significant digit should be displayed after a 2 second delay.
- After all digits have been displayed, the program should return to the start and wait for a button push to start the next timed interval.
- Write an explanation of how the two codes work. (4 points)
- Pre-lab Tasks: (10 points)
- Using the program template 1.1 provided, complete the program by adding the missing parts using the Arduino IDE that begins incrementing an internal variable, icount after the button is pushed. Your program should increment the variable once every second and should display the count (modulo 10) on the 7-segment display. (3 points)
- Using the program template 1.2 provided on the previous page, complete the program by adding the missing parts using the Arduino IDE that counts the number of times a button is pushed over a 10-second interval. Your program should do the following: (3 points)
Template 1.1:
int icount = 0; int StartButtonPin = 6; boolean startButtonLast = LOW; boolean startButtonCurrent = LOW; void setup() { pinMode(StartButtonPin, INPUT); //Configure pin modes for 7-segment LED display pinMode(7, OUTPUT); pinMode(8, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); pinMode(11, OUTPUT); pinMode(12, OUTPUT); pinMode(13, OUTPUT); } boolean waitForButtonPush(boolean lastStartSwitchState) // This function checks for the button press while { // also performing a debounce operation. boolean currentStartSwitchState = digitalRead(StartButtonPin); if(lastStartSwitchState != currentStartSwitchState) delay(20); currentStartSwitchState = digitalRead(StartButtonPin); return currentStartSwitchState; } void LEDDisplay(int count) { switch(count) // Each case value also corresponds to the decimal value. Ex) Case 0 is decimal 0 and so on. { case 0: digitalWrite(7, LOW); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, HIGH); break; case 1: digitalWrite(7, HIGH); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, HIGH); break; case 2: digitalWrite(7, LOW); digitalWrite(8, LOW); digitalWrite(9, HIGH); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, HIGH); digitalWrite(13, LOW); break; //Here add all the other cases as required.. default: // Display letter 'd'.. digitalWrite(7, HIGH); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, HIGH); digitalWrite(13, LOW); }//switch-case ends }//LED Display function end void loop() { startButtonCurrent = waitForButtonPush(startButtonLast); if(startButtonLast == LOW && startButtonCurrent == HIGH) { while(1) //starts an infinite loop { ; //Fill in this missing line: Hint: Increment the counter icount = ; //Fill in this missing parts of this line: Hint: Compute modulo 10 LEDDisplay(icount); // Display on the 7-segment LED display delay(1000); //Delay of 1 second }//while }//if }//loop
Template 1.2:
int icount; int StartButtonPin = 6; int tensValue; int onesValue; unsigned long currentTimeStamp; //The millis() function unsigned long endTimeStamp; //will return an unsigned long datatype so we remain consistent boolean startButtonLast = LOW; boolean startButtonCurrent = LOW; boolean loopExit; void setup() { Serial.begin(9600); // This line enables the serial monitor to be used for us to pinMode(StartButtonPin, INPUT); // print the number of button presses for reading in the console. //7-Segment LED Display pinMode(7, OUTPUT); pinMode(8, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); pinMode(11, OUTPUT); pinMode(12, OUTPUT); pinMode(13, OUTPUT); } void LEDDisplay(int count) //Can be re-used once you have this completed in the previous template. { switch(count) // Each case value also corresponds to the decimal value. Ex) Case 0 is decimal 0 and //so on. { case 0: digitalWrite(7, LOW); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, HIGH); break; case 1: digitalWrite(7, HIGH); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, HIGH); break; //Here add all the other cases as required.. default: // Display letter 'd'.. digitalWrite(7, HIGH); digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, HIGH); digitalWrite(13, LOW); }//switch-case ends }//LED Display function end //This function waits for button push and debounces button boolean waitForButtonPush(boolean lastStartSwitchState) { //This function waits for a button push and also debounces it.. boolean currentStartSwitchState = digitalRead(StartButtonPin); if(lastStartSwitchState != currentStartSwitchState) delay(20); currentStartSwitchState = digitalRead(StartButtonPin); return currentStartSwitchState; } void loop() { if(loopExit != 1) { Serial.println("Press button and continue pressing button as many times as you wish."); Serial.println("within a duration of 10 seconds: The total no of times pushed will be printed."); Serial.print(' '); //Newline } loopExit = 1; //Used as a flag for checking condition for displaying digits on the 7-segment LED icount = 0; LEDDisplay(10); //10 is chosen arbitrarily to force the default case in the switch-case structure //which will turn the LED counter off when the button isn't pushed startButtonCurrent = waitForButtonPush(startButtonLast); //This is a debouncing measure for the button when it is pressed. if(startButtonLast == LOW && startButtonCurrent == HIGH) { currentTimeStamp = millis(); // After button is pressed the current time the program has elapsed for endTimeStamp = millis() + 10000; // is recorded with millis(), we know 10,000 ms = 10 seconds while(currentTimeStamp < endTimeStamp)// While loop runs for 10 seconds according to the condition while { // also constantly checking for button presses and incrementing upon detection. startButtonCurrent = waitForButtonPush(startButtonLast); if(startButtonLast == LOW && startButtonCurrent == HIGH) { ;// Fill in missing line: Hint: Increment counter Serial.println("Hit!.."); } startButtonLast = startButtonCurrent;//Re-assign current state to previous/last. currentTimeStamp = millis(); // Updates elapsed time since while loop has started. }//while Serial.println("Stop!"); Serial.print(' '); // Assumed that button cannot be hit more than 99 times.. tensValue = ;//Fill in missing parts: Hint: extract 10's digit from icount onesValue = ;// Fill in missing parts: Hint: extract unit's digit from icount while (loopExit == 1) // This while statement displays both digits for a 0-99 { //count button push. it will display both digits then exit Serial.print("Button was pushed "); Serial.print(icount); Serial.println(" times."); Serial.print(' '); Serial.println("Waiting for 5 seconds...LOOK AT THE LED DISPLAY "); delay(5000); // Wait for five seconds after stop message. LEDDisplay(MISSING...); //Fill in missing part: Hint: Display the tens digit on the LED delay(2000); LEDDisplay(MISSING...); //Fill in missing part: Hint: Display the units digit on the LED delay(2000); ; // Fill in missing line: Hint: forces exiting the while loop, use correct variable }//while startButtonLast = startButtonCurrent; //current state becomes last state for new repetition of the function loop() }//if }//loop
Step by Step Solution
There are 3 Steps involved in it
Step: 1
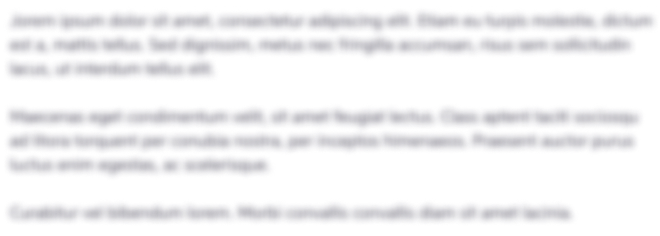
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started