Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need help inplementing a sorting function called sortMovies according to the problem description and c++ code below. Output before applying the sort function and after
Need help inplementing a sorting function called sortMovies according to the problem description and c++ code below.
Output before applying the sort function and after applying the sort function:
Main.cpp:
#include#include //#include "Movie.h" #include "MovieTheater.h" #include #include using namespace std; void PressKeyToContinue(); void LoadMovies(Movie movies[]); void LoadRooms(Movie movies[], int size); void SellTickets(int numTickets, Movie movies[], int size); void Operate(MovieTheater cinema); void PrintPrice(int numTickets); void GetTicketNumber(int &tickets); int SelectMovie(Movie movies[], int size); int GetMovieIndex(Movie movies[], int size, string movie); int main(){ MovieTheater cinema; LoadMovies(cinema.movies); LoadRooms(cinema.movies, cinema.numMovies); Operate(cinema); return 0; } void Operate(MovieTheater cinema){ int numTickets = 0; while(true){ cinema.PrintMovies(); GetTicketNumber(numTickets); SellTickets(numTickets, cinema.movies, cinema.numMovies); numTickets = 0; system("CLS"); } } void PressKeyToContinue(){ string key; cout>key; } void SellTickets(int numTickets, Movie movies[], int size){ int movieIndex = -1; movieIndex = SelectMovie(movies, size); if(movieIndex != -1 && movies[movieIndex].available >= numTickets){ movies[movieIndex].available = movies[movieIndex].available- numTickets; PrintPrice(numTickets); } else if(movieIndex!=-1 && movies[movieIndex].available >tickets; cout390){ cout> movie; if(movie == "cancel"){ cout>numMovies; for(int i=0; i >movies[i].title; infile>>movies[i].runTime; infile>>movies[i].expectedYield; } infile.close(); } void LoadRooms(Movie movies[], int size){ ifstream infile; infile.open("rooms.txt"); for(int i=0; i >movies[i].roomID; infile>>movies[i].capacity; movies[i].available = movies[i].capacity;; //initial availability is equal to capacity as no tickets are sold } infile.close(); }
movies.txt:
9 Gemini_Man 117.9 2 Joker 122.8 2 Zombieland 99.5 2 Knives_Out 130.1 1 Judy 118.3 0 Downton_Abbey 123.8 0 It 170.0 2 Black_and_Blue 108.4 1 Terminator_Dark_Fate 134.9 1
rooms.txt:
A 390 B 390 C 300 D 300 E 300 F 250 G 250 H 250 I 250
Movie.h:
#ifndef MOVIE_H #define MOVIE_H #include#include using namespace std; struct Movie{ Movie(); string title; double runTime; int expectedYield; char roomID; int capacity; int available; }; #endif // MOVIE_H
Movie.cpp:
#include "Movie.h" Movie::Movie() { title = ""; runTime = 0; expectedYield = 0; capacity = 0; available = 0; }
MovieTheater.h:
#ifndef MOVIETHEATER_H #define MOVIETHEATER_H #include "Movie.h" #include#include struct MovieTheater { //member functions MovieTheater(); //constructor ~MovieTheater(); //destructor void PrintMovies(); int GetNumMovies(); string ConvertYieldToString(int yield); void sortMovies(Movie list[], int number); //member variables int numMovies; Movie *movies; }; #endif // MOVIETHEATER_H
MovieTheater.cpp:
#include "MovieTheater.h" MovieTheater::MovieTheater(){ numMovies = GetNumMovies(); movies = new Movie[numMovies]; } MovieTheater::~MovieTheater(){ delete[] movies; } int MovieTheater::GetNumMovies(){ int num; ifstream infile; infile.open("movies.txt"); if(!infile){ cout>num; infile.close(); return num; } } void MovieTheater::sortMovies(Movie list[], int number) { } void MovieTheater::PrintMovies(){ cout Problem description: In this assignment, you must add functionality to a program that sells movie tickets and assigns theater rooms to movies. The program is intended to be used by movie theater tellers. The available movies are loaded from the movies.txt file while the rooms in which the movies are displayed are loaded from "rooms.txt file. The "movies.txt" file contains the number of films playing at the theater in the first line. Then each line corresponds to a film, and includes the title, the runtime, and the expected performance in terms of sales ("2" indicates high expected yield, 1 medium, and "0" low yield). The expected yield is used when assigning the films to a room, as each of the ten rooms has a different number of seats. When assigning films to rooms, the films with a high expected yield must be assigned to the bigger rooms when possible. The "rooms.txt" file contains the room ID which is represented by a letter (A, B, C, etc.), and the number of seats in the room. Once the films have been assigned to rooms, tickets can be sold to viewers. When a ticket is purchased, the number of remaining seats in the room decrements. A viewer can purchase multiple tickets as long as enough seats remain available. Thus, prior to selling a ticket, the films must be displayed along with the total number of seats, available seats, runtime, expected yield (high, medium, low), and room ID. When purchasing a ticket, the viewer is prompted for the number of tickets he/she wishes to purchase. Then the teller can enter the movie title to check its availability and prompt for another title if no seats remain. The teller can always cancel a transaction by inserting "cancel" instead of a movie title. Once a customer has selected the movie and the number of tickets, the price must be displayed. A single ticket costs 7.25 dollars. Implementation Details You have been provided with working code. The program utilizes two structs, Movie, and Movie Theater- The Movie struct has the following member variables: 1. string title: title of the movie, no spaces allowed 2. double runtime: how long the movie lasts in minutes 3. int expected Yield Possible values (2,1,0) which correspond to (high, medium, low) 4. char roomID: ID of the room movie is playing in. Possible values: A, B, C, D, etc. 5. int capacity: How many viewers can fit in room. 6. int available: How many available for purchase still remain. Movie Theater struct has the following member variables: 1. Movie *movies: dynamic array of Movie objects. 2. int num Movies: number of movies in array Use of global variables will incur a deduction of 10 points from your total points. Task 2: In the current state of the program, the movies and rooms are loaded in order from the file. This results in movies with a low expected yield to be assigned rooms of high capacity. This can be seen in the figure below, where the film "Judy" which has a low expected yield, is assigned a bigger room than It which has a high yield. To fix this issue, you must implement a SortMovies function as a member function of the Movie Theater struct. This function will place films with higher yield, earlier on the list. Thus, if this function is called before the "LoadRooms" function, it will assign the bigger rooms to the films with higher expected yield. Note that this is possible, because the rooms are written in order of capacity in "rooms.txt. Once implemented and called appropriately, the initial output of the program should look like the figure below. Playing Now TOTAL SEATS 390 390 300 FILM Gemini_Man Joker Zombieland Knives_out budy Downton_Abbey It Black_and_Blue Terminator_Dark_Fate AVAIL. SEATS 390 390 300 300 RUNTIME 117.9 122.8 99.5 130.1 118.3 123.8 170 108.4 134.9 EXPECTED YIELD ROOM high high high medium 10W low high medium medium m HTG Figure 1. Without Sort FILM Gemini_Man Joker Zombieland TOTAL SEATS 390 390 300 300 300 AVAIL. SEATS 390 390 300 300 RUNTIME 117.9 122.8 99.5 170 130.1 108.4 134.9 118.3 123.8 EXPECTED YIELD ROOM high high high high medium medium medium TOW low Knives_out Black_and_Blue Terminator_Dark_Fate Judy Downton_Abbey - Figure 2. With Sort Problem description: In this assignment, you must add functionality to a program that sells movie tickets and assigns theater rooms to movies. The program is intended to be used by movie theater tellers. The available movies are loaded from the movies.txt file while the rooms in which the movies are displayed are loaded from "rooms.txt file. The "movies.txt" file contains the number of films playing at the theater in the first line. Then each line corresponds to a film, and includes the title, the runtime, and the expected performance in terms of sales ("2" indicates high expected yield, 1 medium, and "0" low yield). The expected yield is used when assigning the films to a room, as each of the ten rooms has a different number of seats. When assigning films to rooms, the films with a high expected yield must be assigned to the bigger rooms when possible. The "rooms.txt" file contains the room ID which is represented by a letter (A, B, C, etc.), and the number of seats in the room. Once the films have been assigned to rooms, tickets can be sold to viewers. When a ticket is purchased, the number of remaining seats in the room decrements. A viewer can purchase multiple tickets as long as enough seats remain available. Thus, prior to selling a ticket, the films must be displayed along with the total number of seats, available seats, runtime, expected yield (high, medium, low), and room ID. When purchasing a ticket, the viewer is prompted for the number of tickets he/she wishes to purchase. Then the teller can enter the movie title to check its availability and prompt for another title if no seats remain. The teller can always cancel a transaction by inserting "cancel" instead of a movie title. Once a customer has selected the movie and the number of tickets, the price must be displayed. A single ticket costs 7.25 dollars. Implementation Details You have been provided with working code. The program utilizes two structs, Movie, and Movie Theater- The Movie struct has the following member variables: 1. string title: title of the movie, no spaces allowed 2. double runtime: how long the movie lasts in minutes 3. int expected Yield Possible values (2,1,0) which correspond to (high, medium, low) 4. char roomID: ID of the room movie is playing in. Possible values: A, B, C, D, etc. 5. int capacity: How many viewers can fit in room. 6. int available: How many available for purchase still remain. Movie Theater struct has the following member variables: 1. Movie *movies: dynamic array of Movie objects. 2. int num Movies: number of movies in array Use of global variables will incur a deduction of 10 points from your total points. Task 2: In the current state of the program, the movies and rooms are loaded in order from the file. This results in movies with a low expected yield to be assigned rooms of high capacity. This can be seen in the figure below, where the film "Judy" which has a low expected yield, is assigned a bigger room than It which has a high yield. To fix this issue, you must implement a SortMovies function as a member function of the Movie Theater struct. This function will place films with higher yield, earlier on the list. Thus, if this function is called before the "LoadRooms" function, it will assign the bigger rooms to the films with higher expected yield. Note that this is possible, because the rooms are written in order of capacity in "rooms.txt. Once implemented and called appropriately, the initial output of the program should look like the figure below. Playing Now TOTAL SEATS 390 390 300 FILM Gemini_Man Joker Zombieland Knives_out budy Downton_Abbey It Black_and_Blue Terminator_Dark_Fate AVAIL. SEATS 390 390 300 300 RUNTIME 117.9 122.8 99.5 130.1 118.3 123.8 170 108.4 134.9 EXPECTED YIELD ROOM high high high medium 10W low high medium medium m HTG Figure 1. Without Sort FILM Gemini_Man Joker Zombieland TOTAL SEATS 390 390 300 300 300 AVAIL. SEATS 390 390 300 300 RUNTIME 117.9 122.8 99.5 170 130.1 108.4 134.9 118.3 123.8 EXPECTED YIELD ROOM high high high high medium medium medium TOW low Knives_out Black_and_Blue Terminator_Dark_Fate Judy Downton_Abbey - Figure 2. With Sort
Step by Step Solution
There are 3 Steps involved in it
Step: 1
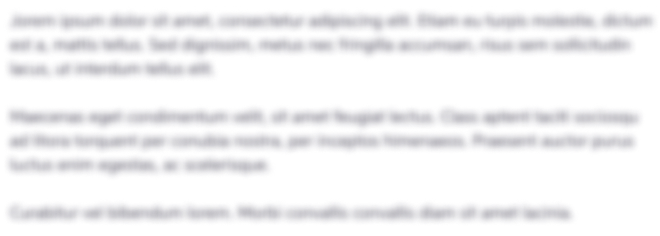
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started