Question
Need help seeing if I am doing this correctly and finsihing it please. Nibble(); Nibble(const Nibble &); Nibble(int parm); // Already WRITTEN Nibble(const int array[]);
Need help seeing if I am doing this correctly and finsihing it please.
Nibble(); Nibble(const Nibble &); Nibble(int parm); // Already WRITTEN Nibble(const int array[]); ~Nibble(); // Already WRITTEN int getValueAsDecimal() const; // Already WRITTEN string getValueAsBinaryString() const; char getValueAsHexCharacter() const; void getValueAsCppString( char answer[]) const; NOTE: In a number the low order digit is on the right and the high order digit is on the left, for 1010 the low order digit is the rightmost 0 and the high order digit is the leftmost 1
1. In the file nibbleImplementation.cpp write the member functions: Nibble::Nibble() Nibble::Nibble(const Nibble & right) Nibble::Nibble(const int array[]) string Nibble::getValueAsBinaryString() const char Nibble::getValueAsHexCharacter() const void Nibble::getValueAsCppString( char answer[]) const
2. In nibbleDriver using the menu execute the automatic computer test for each method you wrote. A perfect score is 5 for each method.
3. In nibbleDriver complete the code for all of the Visual Tests for each method that you wrote. Below is an example of a Visual Test - the student visually verifies that the answer is correct: //5 NibbleGetDecimalValue { // new scope, can use any names cout << "test of get decimal value" << endl; Nibble b(14); cout << "b is "<< b.getValueAsDecimal() << endl; } Should see "b is 14" in the Console Window.
Nibble.h
#ifndef NIBBLE_H
#define NIBBLE_H
#include
#include
using namespace::std;
// Assume there is NO invalid data
const int NUMBER_DIGITS = 4;
class Nibble
{
friend ostream & operator<<(ostream & out, const Nibble & item); // overloaded operator <<
public:
Nibble(); // Default Constructor
Nibble(const Nibble &); // Copy Constructor
Nibble(int parm); // Assume 0 <= parm <= 15 Store parm as
binary number in digits 13 ==> 1101
Nibble(const int array[]); // Assume array holds 4 cells, all either 0 or 1
~Nibble(); // Destructor
int getValueAsDecimal() const; // will return an int between 0 and 15
string getValueAsBinaryString() const; // will return a string object consisting of 0's and 1's
char getValueAsHexCharacter() const; // will return a char representing the hex digit
void getValueAsCppString( char answer[]) const; // will place in the parameter four char 0's and 1's followed by the '\0' char
// OPERATORS - Do Not Write for this Lab, will write in the next lab
// Assignment operator
const Nibble & operator=(const Nibble & r) ;
// Relational opertors
bool operator==(const Nibble & r) const;
bool operator<(const Nibble & r) const;
// Arithmetic operators
Nibble operator+(const Nibble & r) const; // NOTE: because of overflow,
results > 15 will be wrong
// NOTE: work right to left, binary digit by binary digit NEED TO USE A carry bit
// NOTE: MUST USE ARRAY PROCESSING WITH A LOOP
// CANNOT CONVERT TO DECIMAL AND DO THE WORK IN DECIMAL
Nibble operator*(const Nibble & r) const; // NOTE: because of overflow, results > 15 will be wrong
// NOTE: * is repeated addition 2 * 3 is 2 + 2 + 2
// use a for loop for repeated addition
private:
int digits[NUMBER_DIGITS]; // 4 1's or 0's, high order digit (left most digit) in cell 0, low order digit (right most digit) in cell 3
const static char tableOfHex[16]; // holds the character for the first 16 hex numbers 0 - F
};
#endif
nibbleImplementation.cpp
#include "nibbleHeader.h"
const char Nibble::tableOfHex[16] =
{ '0','1','2','3','4','5','6','7','8','9','A','B','C','D','E','F' };
Nibble::Nibble()
{
digits[0]; //am I initializing this correctly for a default constructor
}
Nibble::Nibble(const Nibble& right)
{
//need help here
}
Nibble::Nibble(int parm) // Assume 0 <= parm <= 15
{
digits[0] = parm / 8; // high order digit goes in cell 0
parm = parm % 8;
digits[1] = parm / 4;
parm = parm % 4;
digits[2] = parm / 2;
parm = parm % 2;
digits[3] = parm / 1; // low order digit goes in cell 3
}
Nibble::Nibble(const int array[]) // Assume array holds 4 cells, all either 0 or 1
{
// need help here
}
Nibble::~Nibble()
{
}
int Nibble::getValueAsDecimal() const // will return an int between and 15
{
int answer = digits[0] * 8 + digits[1] * 4 + digits[2] * 2 + digits[3] * 1;
return answer;
}
string Nibble::getValueAsBinaryString() const // will return a string consisting of 0's and 1's
{
// need help here
string answer = "";
// build the answer digit by digit using string concatenation
return answer;
}
char Nibble::getValueAsHexCharacter() const // will return a char representing the hex digit
{
// need help herelllllok
// use table look up: first get decimal value with becomes the subscript into the table of hex digits
return '?'; // Dummy code so that the project will compile
}
void Nibble::getValueAsCppString(char answer[]) const // will place in the
//parameter four char 0's and 1's followed by the '\0' char
{
// need help here
}
// DO NOT WRITE THE CODE FOR THE FOLLOWING IN THIS LAB
// 9 NibbleOpertorAssignment
const Nibble & Nibble::operator=(const Nibble & r)
{
// leave blank for now
return *this;
}
ostream& operator<<(ostream& out, const Nibble& item) // friend function
{
// Allows the user to view the data as output
for (int i = 0; i < NUMBER_DIGITS; i++)
out << item.digits[i];
return out;
}
bool Nibble::operator==(const Nibble& r) const
{
// leave blank for now
return false;
}
bool Nibble::operator<(const Nibble& r) const
{
// leave blank for now
return false;
}
Nibble Nibble::operator+(const Nibble& r) const // NOTE: because of overflow, results > 15 will be wrong
// NOTE: work right to left, binary digit by binary digit NEED TO
//USE A carry bit
// NOTE: MUST USE ARRAY PROCESSING WITH A LOOP
// CANNOT CONVERT TO DECIMAL AND DO THE WORK IN DECIMAL
{
Nibble ans;
// leave blank for now
return ans;
}
Nibble Nibble::operator*(const Nibble& r) const // NOTE: because of overflow, results > 15 will be wrong
// NOTE: * is repeated addition 2 * 3 is 2 + 2 + 2
// use a for loop for repeated addition
{
Nibble ans;
// leave blank for now
return ans;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
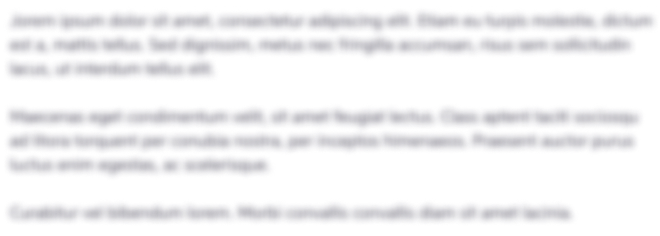
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started