Question
need help solving methods Implement a Queue ADT class by completing the provided skeleton code in the file queue_sll.py. You will use a chain of
need help solving methods
Implement a Queue ADT class by completing the provided skeleton code in the file queue_sll.py. You will use a chain of Singly-Linked Nodes (the provided SLNode) as the underlying storage for your Queue ADT. Be sure to review the Exploration on Queues for an example. 2. Once completed, your implementation will include the following methods: enqueue() dequeue() front() 3. We will test your implementation with different types of objects, not just integers. We guarantee that all such objects will have correct implementations of methods __eq__(), __lt__(), __gt__(), __ge__(), __le__(), and __str__(). 4. The number of objects stored in the queue at any given time will be between 0 and 1,000,000 inclusive. The queue must allow for the storage of duplicate elements. 5. RESTRICTIONS: You are not allowed to use ANY built-in Python data structures and/or their methods
#SLNode import
class SLNode: """ Singly Linked List Node class DO NOT CHANGE THIS CLASS IN ANY WAY """ def __init__(self, value: object, next=None) -> None: self.value = value self.next = next
#skeleton code
from SLNode import SLNode class QueueException(Exception): """ Custom exception to be used by Queue class DO NOT CHANGE THIS METHOD IN ANY WAY """ pass class Queue: def __init__(self): """ Initialize new queue with head and tail nodes DO NOT CHANGE THIS METHOD IN ANY WAY """ self._head = None self._tail = None def __str__(self): """ Return content of queue in human-readable form DO NOT CHANGE THIS METHOD IN ANY WAY """ out = 'QUEUE [' if not self.is_empty(): node = self._head out = out + str(node.value) node = node.next while node: out = out + ' -> ' + str(node.value) node = node.next return out + ']' def is_empty(self) -> bool: """ Return True is the queue is empty, False otherwise DO NOT CHANGE THIS METHOD IN ANY WAY """ return self._head is None def size(self) -> int: """ Return number of elements currently in the queue DO NOT CHANGE THIS METHOD IN ANY WAY """ node = self._head length = 0 while node: length += 1
node = node.next return length # ----------------------------------------------------------------------- def enqueue(self, value: object) -> None: """ TODO: Write this implementation """ pass def dequeue(self) -> object: """ TODO: Write this implementation """ pass def front(self) -> object: """ TODO: Write this implementation """ pass # ------------------- BASIC TESTING ----------------------------------------- if __name__ == "__main__": print(" # enqueue example 1") q = Queue() print(q) for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) print(" # dequeue example 1") q = Queue() for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) for i in range(6): try: print(q.dequeue()) except Exception as e: print("No elements in queue", type(e)) print(' #front example 1') q = Queue() print(q) for value in ['A', 'B', 'C', 'D']: try: print(q.front()) except Exception as e: print("No elements in queue", type(e)) q.enqueue(value) print(q)
#examples
enqueue
Example #1: q = Queue() print(q) for value in [1, 2, 3, 4, 5]: q.enqueue(value)m print(q)
Output: QUEUE [] QUEUE [1 -> 2 -> 3 -> 4 -> 5]
dequeue
Example #1: q = Queue() for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) for i in range(6): try: print(q.dequeue()) except Exception as e: print("No elements in queue", type(e)) Output:QUEUE [1 -> 2 -> 3 -> 4 -> 5] 1 2 3 4 5 No elements in queue
front
Example #1: q = Queue() print(q) for value in ['A', 'B', 'C', 'D']: try: print(q.front()) except Exception as e: print("No elements in queue", type(e)) q.enqueue(value) print(q)
Output: QUEUE [] No elements in queue A A A QUEUE [A -> B -> C -> D]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
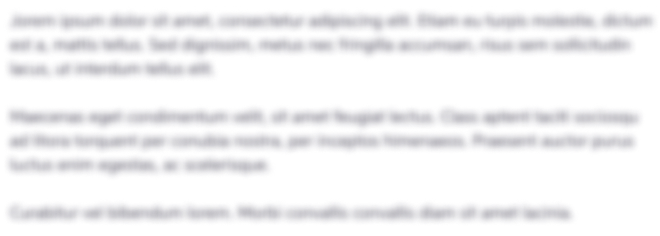
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started