Question
need help solving methods Implement a Queue ADT class that utilizes a circular buffer (as described in the Exploration) by completing the provided skeleton code
need help solving methods
Implement a Queue ADT class that utilizes a circular buffer (as described in the Exploration) by completing the provided skeleton code in the file queue_sa.py. You will use the Static Array data structure from previous assignments as the underlying storage for your Queue ADT. 2. Once completed, your implementation will include the following methods: enqueue() dequeue() front() The following private helper method in the skeleton code is used by __str__() to handle the wraparound in the circular buffer. You may find it helpful for your methods: _increment() There is also a suggested (optional and private) helper method in the skeleton code that you may wish to implement, to assist with resizing: _double_queue() 3. We will test your implementation with different types of objects, not just integers. We guarantee that all such objects will have correct implementations of methods __eq__(), __lt__(), __gt__(), __ge__(), __le__(), and __str__(). 4. The number of objects stored in the queue at any given time will be between 0 and 1,000,000 inclusive. The queue must allow for the storage of duplicate elements. 5. RESTRICTIONS: You are not allowed to use ANY built-in Python data structures and/or their methods
#skelton code
from static_array import StaticArray class QueueException(Exception): """Custom exception to be used by Queue class.""" pass class Queue: def __init__(self) -> None: """Initialize new queue based on Static Array.""" self._sa = StaticArray(4) self._front = 0 self._back = -1 self._current_size = 0 def __str__(self) -> str: """Override string method to provide more readable output.""" size = self._current_size out = "QUEUE: " + str(size) + " element(s). [" front_index = self._front for _ in range(size - 1): out += str(self._sa[front_index]) + ', ' front_index = self._increment(front_index) if size > 0: out += str(self._sa[front_index]) return out + ']' def is_empty(self) -> bool: """Return True if the queue is empty, False otherwise.""" return self._current_size == 0 def size(self) -> int: """Return number of elements currently in the queue.""" return self._current_size def print_underlying_sa(self) -> None: """Print underlying StaticArray. Used for testing purposes.""" print(self._sa) def _increment(self, index: int) -> int: """Move index to next position."""
# employ wraparound if needed index += 1 if index == self._sa.length(): index = 0 return index # ---------------------------------------------------------------------- # def enqueue(self, value: object) -> None: """ TODO: Write this implementation """ pass def dequeue(self) -> object: """ TODO: Write this implementation """ pass def front(self) -> object: """ TODO: Write this implementation """ pass # The method below is optional, but recommended, to implement. # # You may alter it in any way you see fit. # def _double_queue(self) -> None: """ TODO: Write this implementation """ pass # ------------------- BASIC TESTING ----------------------------------------- if __name__ == "__main__": print(" # Basic functionality tests #") print(" # enqueue()") q = Queue() print(q) for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) print(" # dequeue()") q = Queue() for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) for _ in range(q.size() + 1): try: print(q.dequeue()) except Exception as e: print("No elements in queue", type(e))
for value in [6, 7, 8, 111, 222, 3333, 4444]: q.enqueue(value) print(q) q.print_underlying_sa() print(" # front()") q = Queue() print(q) for value in ['A', 'B', 'C', 'D']: try: print(q.front()) except Exception as e: print("No elements in queue", type(e)) q.enqueue(value) print(q) print(" # Circular buffer tests: # ") def action_and_print( header: str, action: callable, values: [], queue: Queue) -> None: """ Print header, perform action, then print queue and its underlying storage. """ print(header) if values: for value in values: action(value) else: action() print(queue) queue.print_underlying_sa() print() q = Queue() # action_and_print("# Enqueue: 2, 4, 6, 8", q.enqueue, [2, 4, 6, 8], q) # Calling the action_and_print() function declared two lines above, # would be equivalent to following lines of code: print("# Enqueue: 2, 4, 6, 8") test_case = [2, 4, 6, 8] for value in test_case: q.enqueue(value) print(q) q.print_underlying_sa() print() action_and_print("# Dequeue a value", q.dequeue, [], q) action_and_print("# Enqueue: 10", q.enqueue, [10], q) action_and_print("# Enqueue: 12", q.enqueue, [12], q) print("# Dequeue until empty") while not q.is_empty(): q.dequeue() print(q) q.print_underlying_sa() print()
action_and_print("# Enqueue: 14, 16, 18", q.enqueue, [14, 16, 18], q) action_and_print("# Enqueue: 20", q.enqueue, [20], q) action_and_print("# Enqueue: 22, 24, 26, 28", q.enqueue, [22, 24, 26, 28], q) action_and_print("# Enqueue: 30", q.enqueue, [30], q
#examples
enqueue
q = Queue() print(q) for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q)
Output: QUEUE: 0 elements. [] QUEUE: 5 elements. [1, 2, 3, 4, 5]
dequeue
q = Queue() for value in [1, 2, 3, 4, 5]: q.enqueue(value) print(q) for i in range(q.size() + 1): try: print(q.dequeue()) except Exception as e: print("No elements in queue", type(e)) for value in [6, 7, 8, 111, 222, 3333, 4444]: q.enqueue(value) print(q) q.print_underlying_sa()
Output: QUEUE: 5 elements. [1, 2, 3, 4, 5] 1 2 3 4 5 No elements in queue QUEUE: 7 element(s). [6, 7, 8, 111, 222, 3333, 4444] STAT_ARR Size: 8 [111, 222, 3333, 4444, 5, 6, 7, 8]
front
Example #1: q = Queue() print(q) for value in ['A', 'B', 'C', 'D']: try: print(q.front()) except Exception as e: print("No elements in queue", type(e)) q.enqueue(value) print(q)
Output: QUEUE: 0 elements. [] No elements in queue A A A QUEUE: 4 elements. [A, B, C, D]
Testing for Circular Buffer print( Circular buffer tests: # ) q = Queue() print(# Enqueue: 2, 4, 6, 8) test_case = [2, 4, 6, 8] for value in test_case: q.enqueue(value) print(q) q.print_underyling_sa() print()
Output: # Enqueue: 2, 4, 6, 8 QUEUE: 4 element(s). [2, 4, 6, 8] STAT_ARR Size: 4 [2, 4, 6, 8]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
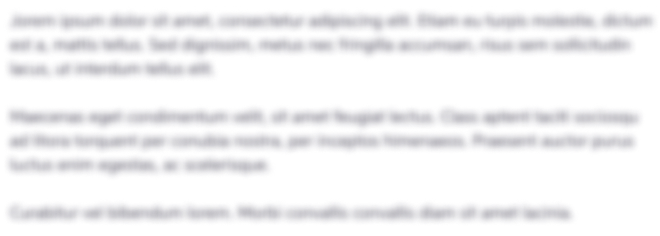
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started