Question
Need help urgent!!! Write a C++ class with separate .h and .cpp file, use partially filled arrays, Create a new class called Pokedex that uses
Need help urgent!!!
Write a C++ class with separate .h and .cpp file, use partially filled arrays, Create a new class called Pokedex that uses a private string array to store the list of Pokemon
pokemon.cpp to implement the functions specified in pokemon.h. All functions in the .h and .cpp file have to be documented. 1-2 lines of documentation is usually sufficient.
You do not need to modify pokedex-commands.cpp.
You can modify .clang-tidy as necessary, but make sure you document any tests you have disabled.
Run create-output.sh script to create output.txt file
Need help with pokedex.cpp:
#include "pokedex.h"
#include
using namespace std;
// Your code goes here
/*
Introduces the following concepts:
- using shell scripts to fully test programs
- command line parameters
- reading strings, integers and whole lines from file using ifstream, good, ignore, getline
- using operator<< to print objects
- const variables
- const functions
- const reference parameters
- const reference return values
- functions with undefined behavior
- using clang-tidy, clang-format and code coverage tools
- g++ flags and valgrind to check for memory leaks
*/
Main.cpp
#include "pokedex.h"
#include
#include
#include
#include
#include
using namespace std;
// forward delaration, defined in library-commands.cpp
void execute_commands(const string &filename);
void test1() {
Pokedex pdx;
stringstream strs;
// // NOLINTNEXTLINE - would normally use empty
assert(pdx.size() == 0);
assert(pdx.empty());
strs << pdx;
assert(strs.str() == "[]");
cout << "test1 complete" << endl;
}
void test2() {
Pokedex pdx;
stringstream strs;
pdx.insert("Pikachu");
assert(pdx.size() == 1);
assert(!pdx.empty());
assert(pdx.at(0) == "Pikachu");
assert(pdx.front() == pdx.back());
strs.str("");
strs << pdx;
assert(strs.str() == "[Pikachu]");
cout << "test2 complete" << endl;
}
void test3() {
Pokedex pdx;
stringstream strs;
pdx.insert("Pikachu");
pdx.insert("Charmander");
assert(pdx.size() == 2);
assert(pdx.at(0) == pdx.front());
assert(pdx.at(1) == pdx.back());
strs.str("");
strs << pdx;
assert(strs.str() == "[Charmander, Pikachu]");
pdx.erase(0);
assert(pdx.front() == pdx.back());
strs.str("");
strs << pdx;
assert(strs.str() == "[Pikachu]");
cout << "test3 complete" << endl;
}
void test4() {
Pokedex pdx;
stringstream strs;
pdx.insert("Charmander");
pdx.insert("Pikachu");
pdx.insert("Bulbasaur");
strs << pdx;
assert(strs.str() == "[Bulbasaur, Charmander, Pikachu]");
pdx.erase(1);
strs.str("");
strs << pdx;
assert(strs.str() == "[Bulbasaur, Pikachu]");
cout << "test4 complete" << endl;
}
int main(int argc, char *argv[]) {
test1();
test2();
test3();
test4();
if (argc >= 1) {
for (int i = 1; i < argc; ++i) {
string commandFile = argv[i];
cout << "*** Executing commands from " << commandFile << endl;
execute_commands(commandFile);
}
}
cout << "Done." << endl;
}
pokedex-commonds.cpp
#include "pokedex.h"
#include
#include
#include
#include
#include
using namespace std;
void execute_commands(const string &filename) {
ifstream ifs(filename);
if (!ifs.good()) {
cout << "Failed to open " << filename << endl;
return;
}
Pokedex pdx;
while (ifs.good()) {
string command;
ifs >> command;
// // single word commands
if (command == "size") {
cout << "Executing: " << command << endl;
cout << pdx.size() << endl;
} else if (command == "max_size") {
cout << "Executing: " << command << endl;
cout << Pokedex::max_size() << endl;
} else if (command == "empty") {
cout << "Executing: " << command << endl;
cout << (pdx.empty() ? "true" : "false") << endl;
} else if (command == "front") {
cout << "Executing: " << command << endl;
cout << pdx.front() << endl;
} else if (command == "back") {
cout << "Executing: " << command << endl;
cout << pdx.back() << endl;
} else if (command == "pop_back") {
// no output
cout << "Executing: " << command << endl;
pdx.pop_back();
} else if (command == "print") {
// call insertion operator
cout << "Executing: " << command << endl;
cout << pdx << endl;
} else if (command == "at") {
int num;
ifs >> num;
cout << "Executing: " << command << " " << num << endl;
cout << pdx.at(num) << endl;
} else if (command == "insert") {
// no output
string book;
// // skip the space after num
ifs.ignore();
// // read the whole line, not just up to the first space
getline(ifs, book);
cout << "Executing: " << command << " " << book << endl;
pdx.insert(book);
} else if (command == "erase") {
// // no output
int num;
ifs >> num;
cout << "Executing: " << command << " " << num << endl;
pdx.erase(num);
} else {
cout << "Invalid command: " << command << endl;
}
}
ifs.close();
}
pokedex.h:
#ifndef POKEDEX_H
#define POKEDEX_H
#include
#include
using namespace std;
class Pokedex {
public:
// constructor - create empty Pokedex
Pokedex();
// Return size
int size() const;
// Return maximum size, capacity of Pokedex
static int max_size();
// return true if Pokedex is empty
bool empty() const;
// return pokemon at given index
// undefined behaviour for n < 0 or n >= size
const string &at(int n) const;
// return pokemon at the front, alphabetically first one
const string &front() const;
// return pokemon at the front, alphabetically last one
const string &back() const;
// Add pokemon to Pokedex, keep the Pokedex list sorted
// Can have multiple pokemon with the same name
// Pokemon is not inserted if Pokedex is already full
void insert(const string &pokemon);
// Delete the last element
void pop_back();
// Erase element at location, move other elements as needed
// undefined behaviour if given index is not valid
void erase(int n);
private:
// maximum capacity of Pokedex
static const int MAX = 10;
// sorted list of pokemon in Pokedex
string pokemons[MAX];
// current internal size
int msize = 0;
};
// insertion operator, so we can use "cout << pdx"
ostream &operator<<(ostream &out, const Pokedex &pdx);
#endif // POKEDEX_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
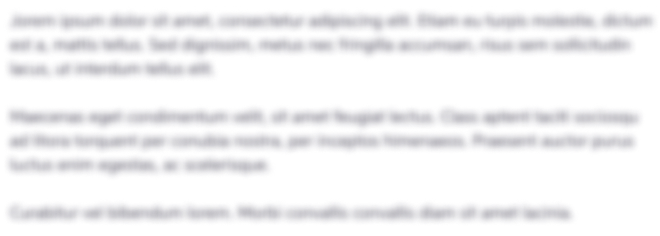
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started