Question
Need help with C++! Please answer this question! Objectives: Writing a class header, class implementation, and test client in separate files. Implementing member functions (including
Need help with C++! Please answer this question!
Objectives:
- Writing a class header, class implementation, and test client in separate files.
- Implementing member functions (including default and parameterized constructors).
- Reading data from a text file.
- Loading and accessing an array of class objects.
Instructions:
Download the files Item.h, Item.cpp, TestClient.cpp, and input.txt from Canvas. Create a new project in Visual Studio for Lab05 and place these files into your project.The Item class is designed to store data about an item being sold at a shop. Accessor functions, mutator functions, and a print function are supported.
Part One
Implement the following member functions according to the TODO comments written in the Item.cpp file.
Part Two
Implement the following functionality in main according to the TODO comments written in the TestClient.cpp file.
Below is the codes:
item.cpp code
#include "item.h"
Item::Item()
{
// TODO: Implement the default constructor.
// Set the member variables to default values.
// The integers and doubles can be set to 0. The strings can
// be set to "unknown". (5 points)
}
Item::Item(int id, string name, double itemPrice, int num)
{
// TODO: Implement the parameterized constructor.
// Set the member variables using the corresponding
// parameters. (5 points)
}
int Item::getItemId() const
{
// TODO: Implement the getter function.
// Return the corresponding member variable. (5 points)
}
string Item::getItemName() const
{
// TODO: Implement the getter function.
// Return the corresponding member variable. (5 points)
}
double Item::getPrice() const
{
// TODO: Implement the getter function.
// Return the corresponding member variable. (5 points)
}
int Item::getNumberInStock() const
{
// TODO: Implement the getter function.
// Return the corresponding member variable. (5 points)
}
void Item::setItemId(int id)
{
// TODO: Implement the setter function.
// Set the corresponding member variable using the parameter.
// (5 points)
}
void Item::setItemName(string name)
{
// TODO: Implement the setter function.
// Set the corresponding member variable using the parameter.
// (5 points)
}
void Item::setPrice(double itemPrice)
{
// TODO: Implement the setter function.
// Set the corresponding member variable using the parameter.
// (5 points)
}
void Item::setNumberInStock(int num)
{
// TODO: Implement the setter function.
// Set the corresponding member variable using the parameter.
// (5 points)
}
void Item::printItem() const
{
// TODO: Implement the print function.
// Output the member variables stored in the object.
// (5 points)
}
item.h code
#pragma once
#include
#include
using namespace std;
class Item
{
public:
Item();
Item(int, string, double, int);
int getItemId() const;
string getItemName() const;
double getPrice() const;
int getNumberInStock() const;
void setItemId(int);
void setItemName(string);
void setPrice(double);
void setNumberInStock(int);
void printItem() const;
private:
int itemId;
string itemName;
double price;
int numberInStock;
};
testClient.cpp
#include
#include
#include
#include "item.h"
using namespace std;
const int ARR_SIZE = 5;
const string FILENAME = "input.txt";
int main()
{
Item itemList[ARR_SIZE];
ifstream inData;
int tempItemId;
string tempItemName;
double tempPrice;
int tempNumberInStock;
// TODO: Open the "input.txt" text file. Use the data in the text file to
// load the itemList array. Specifically, read the value from the input
// file into the temporary variables declared above, and then use the set
// functions you wrote in item.cpp to load the data into the objects.
// (30 points)
// TODO: Iterate through the array of item objects, and print
// each object's data. (15 points)
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
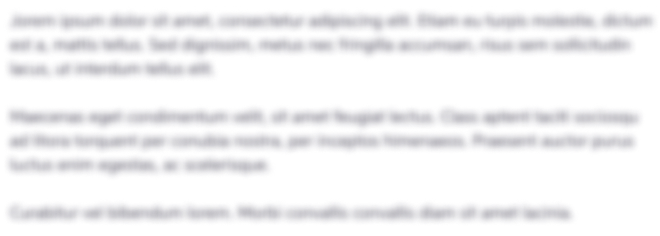
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started