Question
Need help with C++ Use Client.cpp and Box.h to complete the Todo statements on Box.cpp CLIENT.CPP #include Box.h int main() { Box Box1; // Instantiate
Need help with C++
Use Client.cpp and Box.h to complete the Todo statements on Box.cpp
CLIENT.CPP
#include "Box.h"
int main() {
Box Box1; // Instantiate Box1 of type Box
Box Box2; // Instantiate Box2 of type Box
Box Box3; // Instantiate Box3 of type Box
double volume = 0.0; // Store the volume of a box here
// Box1 specification
Box1.setLength(6.0);
Box1.setBreadth(7.0);
Box1.setHeight(5.0);
cout
cout
// Get the volume of Box1
volume = Box1.getVolume();
cout
Box1 = ++Box1;
cout
cout
// Get the new volume of Box1
volume = Box1.getVolume();
cout
// Box2 specification
Box2.setLength(12.0);
Box2.setBreadth(13.0);
Box2.setHeight(10.0);
cout
cout
// Get the volume of Box2
volume = Box2.getVolume();
cout
Box2 = --Box2;
cout
cout
// Get the new volume of Box2
volume = Box2.getVolume();
cout
// Add two Boxes as follows:
Box3 = Box1 + Box2;
cout
cout
// Get the volume of Box3
volume = Box3.getVolume();
cout
return 0;
}
Box.h
#include
using namespace std;
class Box {
public:
Box(); // default constructor
Box(double, double, double); // parameter constructor
double getVolume();
void setLength(double length);
void setBreadth(double breadth);
void setHeight(double height);
Box operator+(Box const&); // addition operator overload, member function
Box& operator++(); // pre-increment operator overload, member function
private:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
friend ostream& operator out, Box& box); // stream insertion operator overload overload, firend function
friend Box& operator--(Box&); // pre-decrement operator overload, firend function
};
Box.cpp
#include "Box.h"
Box::Box()
{
//TODO: Complete the default constructor
//and set the member variables as 0. 5pts
}
Box::Box(double length, double breadth, double height) {
//TODO: Complete the parameter constructor
//and set the member variables. 5pts
}
double Box::getVolume() {
//TODO: Complete the getVolume function and return the volume.
// V = Length * Breadth * Height 5pts
}
void Box::setLength(double length) {
// TODO: Set the member variable length according to the parameter
// by using this pointer. 5pts
}
void Box::setBreadth(double breadth) {
// TODO: Set the member variable length according to the parameter
// by using this pointer. 5pts
}
void Box::setHeight(double height) {
// TODO: Set the member variable length according to the parameter
// by using this pointer. 5pts
}
Box Box::operator+(Box const& other) {
//TODO: Implement the addition operator overload as a member function
//by adding all the three member variables of two Box objects, respectively. 15pts
}
Box& Box::operator++() {
//TODO: Implement the pre-increment operator overload as a member function 15pts
}
ostream& operator
{
// TODO: Implement the stream insertion operator overload as a friend function.
// print the Length, Breadth, Height of the box. 25pts
}
Box& operator--(Box& box) {
if (box.length
cout
//TODO: Implement the pre-decrement operator overload as a friend function
//by decreasing all the three member variables by 1, respectively. 15pts
}
sample output :
Step by Step Solution
There are 3 Steps involved in it
Step: 1
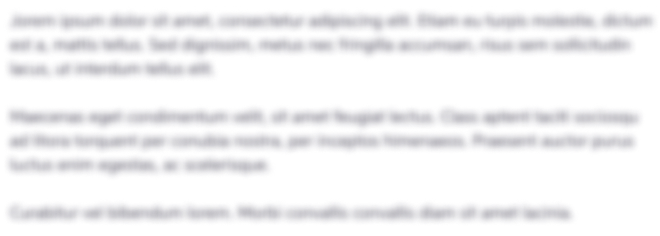
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started