Question
need help with Java programming language This program is for encrypting and decrypting files. it's already done and it works only if the user inputs
need help with Java programming language
This program is for encrypting and decrypting files. it's already done and it works only if the user inputs the right extension for the file
if the user in encrypting they should put the .txt extension after the file name and if the user is decrypting they should put the extension .enc after the file name but if the user put file name without extension the program terminates.
the program is supposed to show an error message "Wrong file extension" and return to the menu again when the user enters the file name without an extension. it should be for both encrypting and decrypting.
Here is the code.
--------------------------------------------------------------------------------------------------------
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.Scanner;
public class Project{
/**
*
* Method to get input from user
*
*/
public static int getMenuOption() {
Scanner in = new Scanner(System.in);
int choi;
// asking the user to input what the want
while (true) {
System.out.println("1.Encrypt");
System.out.println("2.Decrypt");
System.out.println("3.Quit");
System.out.print("What would you like to do? ");
choi = in.nextInt();
if (choi < 1 || choi > 3) {
// if choice is not valid
System.out.println("Invalid choice, try again!");
}
else
break;
}
return choi;// returning choice
}
//this method is to encrypt file
public static void encrypt(File inputFile, int key) throws FileNotFoundException {
//getting the file name from the user
String fileName = inputFile.getName().trim();
//checking for txt extension using string method
if (!fileName.trim().split("\\.")[1].equals("txt")) {
System.out.println("Inavlid file ");
// returning to menu
return;
}
else {
//reading the file
Scanner fileReader = new Scanner(inputFile);
//changing the extension for the file to enc
String outputFile = fileName.split("\\.")[0] + ".enc";
File outFile = new File(outputFile);
PrintWriter writer = new PrintWriter(outputFile);
while (fileReader.hasNext()) {
//reading line by line
String ww = fileReader.nextLine();
String enc_string = "";
//using for loop encoding the string
for (int i = 0; i < ww.length(); i++) {
int ascii = (int) ww.charAt(i);
ascii = ascii + key;
enc_string = enc_string + (char) ascii;
}
//Writing the encrypted string to out put file
writer.write(enc_string + " ");
writer.flush();
}
System.out.println("Encrypted successfully !");
}
}
/**
*
* this method is for decrypting the file
*/
public static void decrypt(File inputFile, int key) throws FileNotFoundException {
//getting the file name for decrypted
String fileName = inputFile.getName().trim();
//checking for file extension .enc
if (!fileName.trim().split("\\.")[1].equals("enc")) {
System.out.println("Inavlid file ");
// returning to main
return;
}
else {
Scanner fileReader1 = new Scanner(inputFile);
//creating the file name ended with .txt
String outputFileName = fileName.split("\\.")[0] + ".txt";
File outFile = new File(outputFileName);
PrintWriter writer = new PrintWriter(outFile);
while (fileReader1.hasNext()) {
//reading file line by line
String t = fileReader1.nextLine();
//decrypting the string using for loop
String dec_string = "";
for (int i = 0; i < t.length(); i++) {
int rrr = (int) t.charAt(i);
rrr = rrr - key;
dec_string = dec_string + (char) rrr;
}
//writting to file
writer.write(dec_string + " ");
writer.flush();
}
System.out.println("Decrypted successfully");
}
}
// Main method for executing program
public static void main(String[] args) throws FileNotFoundException {
Scanner in = new Scanner(System.in);
while (true) {
int choice = getMenuOption();
if (choice == 1) {
System.out.print("Enter the file Name : ");
String filenamee = in.next();
System.out.print("Entet the shift key : ");
int key = in.nextInt();
encrypt(new File(filenamee), key);
}
else if (choice == 2) {
System.out.print("Enter the file Name : ");
String filenamee = in.next();
System.out.print("Entet the shift key : ");
int key = in.nextInt();
decrypt(new File(filenamee), key);
}
else if (choice == 3) {
System.exit(0);
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
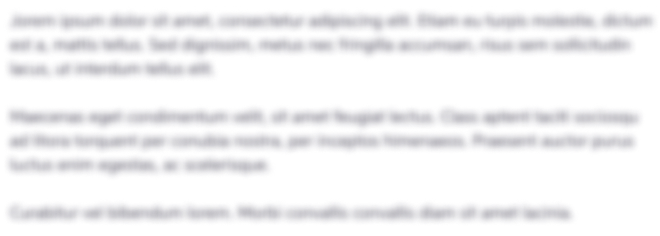
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started