Need help with Java programming please. Write a program that reads a line of text, changes each lowercase letter to uppercase and places each letter
Need help with Java programming please.
Write a program that reads a line of text, changes each lowercase letter to uppercase and places each letter both in a queue and onto a stack.
The program should then verify whether the line of text is a palindrome.
EXCEPT for the following refinements and clarifications:
Write a class that contains a static isPalindrome method to report (as true or false) if a string passed as the parameter is a palindrome or not. Write another class (in a separate file) to test the method.
Your method should push each alpha character in the string onto a stack and also add it to a queue. Then, pop each character off of the stack at the same time as you remove one from the queue and compare them for equality. If they all pass, you have a palindrome (because the LIFO nature of the stack reverses the string while the FIFO nature of the queue does not).
Don't use any of the authors source code, instead, pick a stack and queue class from the Java 8 SE API. Notice that the Stack is a concrete class in Java, but the Queue is an interface (so you will need to choose one of the implementing, concrete Queue classes). Note also that Java 8 features generics, so you can make a stack of characters as follows:
StackmyStack = new Stack ();
Remember, the Java API for Stack is a bit different from that in the textbook so you will use peek, push and pop. Also, for your choice of Queue (perhaps the ArrayDeque or the LinkedList) you would likely use peek, add and remove.
As before, the testing class should use iteration to prompt the user for strings to evaluate, terminating on the entry of an empty string. Only the letters (alpha-characters) in the string are evaluated (spaces, punctuation and numbers are discarded while casing is ignored). Do all the work in the method, not the driver. The pre-condition for the method is only that the parameter is a valid string.
Name your implementation class PalindromeEvaluator and your testing class PalindromeTester (because that is what my script will look for - following all the instructions counts for points).
Also as before, make sure your testing method doesn't re-open your Scanner (or any other class you wrap around System.in) more than once (that would break the standard input, a general programming standard) and be sure your posted solution will compile at the command line with the Java 8 SE compiler. Make sure your output prints true or false followed by the input string as in:
true : Madam false: Sir true : Was it a cat I saw?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
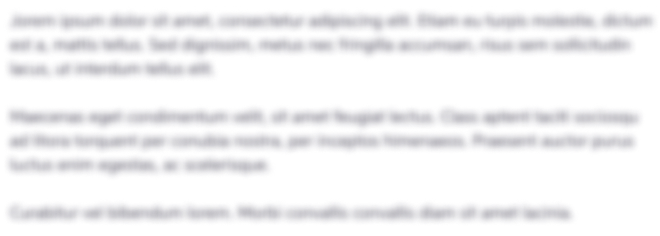
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started