Question
Need Help With NodeList first(), last(), find() and insertAfter. and insert before (numbers 6-10) description here: Provided files down below. /////////////////////////////////////////////////////////////////////////////////////// NodeList.h #ifndef NODELIST_H_ #define
Need Help With NodeList first(), last(), find() and insertAfter. and insert before (numbers 6-10)
description here:
Provided files down below.
\///////////////////////////////////////////////////////////////////////////////////////
NodeList.h
#ifndef NODELIST_H_
#define NODELIST_H_
#include "DataType.h"
class Node{
friend class NodeList; //allows direct accessing of link and data from class NodeList
public:
Node() :
m_next( NULL )
{
}
Node(const DataType& data, Node* next = NULL) :
m_next( next ),
m_data( data )
{
}
Node(const Node& other) :
m_next( other.m_next ),
m_data( other.m_data )
{
}
DataType& GetData(){
return m_data;
}
const DataType& GetData() const{
return m_data;
}
private:
Node* m_next;
DataType m_data;
};
class NodeList{
friend std::ostream& operator
const NodeList& nodeList);
public:
NodeList(); //(1)
NodeList(size_t count, const DataType& value); //(2)
NodeList(const NodeList& other); //(3)
~NodeList(); //(4)
NodeList& operator= (const NodeList& rhs); //(5)
Node* First(); //(6)
Node* Last(); //(7)
Node* Find(const DataType & target, //(8)
Node * & previous,
const Node * start = NULL);
Node* InsertAfter(const DataType& target, //(9)
const DataType& value);
Node* InsertBefore(const DataType& target, //(10)
const DataType& value);
Node* Erase(const DataType& target); //(11)
DataType& operator[] (size_t position); //(12a)
const DataType& operator[] (size_t position) const; //(12b)
size_t size() const; //(13)
bool empty() const; //(14)
void clear(); //(15)
private:
Node * m_head;
};
#endif //NODELIST_H_
////////////////////////////////////////////////////////////////////////////////////////////////////////
DataType.h
#ifndef DATATYPE_H_
#define DATATYPE_H_
#include
class DataType{
friend std::ostream& operator
friend std::istream& operator>>(std::istream& is, DataType& dataType);
public:
DataType();
DataType(int intVal, double doubleVal);
bool operator==(const DataType& other_dataType) const;
DataType& operator= (const DataType& other_dataType);
int GetIntVal() const;
void SetIntVal(int i);
double GetDoubleVal() const;
void SetDoubleVal(double d);
private:
int m_intVal;
double m_doubleVal;
};
#endif //DATATYPE_H
/////////////////////////////////////////////////////////////////////////////////
DataType.cpp
#include "DataType.h"
#include
DataType::DataType(){
m_intVal = 0;
m_doubleVal = 0.0;
}
DataType::DataType(int intVal, double doubleVal){
m_intVal = intVal;
m_doubleVal = doubleVal;
}
bool DataType::operator==(const DataType& rhs) const{
return m_intVal==rhs.m_intVal && m_doubleVal==rhs.m_doubleVal; }
DataType& DataType::operator=(const DataType& rhs){
if (this != &rhs){
m_intVal = rhs.m_intVal;
m_doubleVal = rhs.m_doubleVal;
}
return *this;
}
int DataType::GetIntVal() const{
return m_intVal;}
void DataType::SetIntVal(int i){
m_intVal = i;}
double DataType::GetDoubleVal() const{
return m_doubleVal;}
void DataType::SetDoubleVal(double d){
m_doubleVal = d;}
std::ostream& operator
os
return os;
}
std::istream& operator>>(std::istream& is, DataType& dt){
char in_buf[255];
is >> in_buf;
dt.m_doubleVal = atof(in_buf);
dt.m_intVal = (int)dt.m_doubleVal;
dt.m_doubleVal -= dt.m_intVal;
return is;
}
/////////////////////////////////////////////////////////////////////////////////////
Shallow object copies. (4) Destructor - will destroy the instance of the NodeList object. Nole: Any allocated memory taken up by elements (Nodes) belonging to the list has to be deallocated in here. (5) operator= will assign a new value to the calling NodeList object, which will be an exact copy of the rhs NodeList object. Returns a reference to the calling object to be used for cascading operator as per standard practice. Note: Think what needs to happen before allocating new memory for the new data to be held by the calling object. (6) First returns a pointer to the first element (Node) of the list, or NULL if the list is empty (7Last returns a Pointer to the last clement (Node) of the list, or NULL if the list is empty (8) Find returns a pointer to the first element (Node) of the list, that holds the same value as passed parameter DataType target (the equality operator-as overloaded in class DataType should be used to check that). Ifit fails i does not find the value it searched for inside a Node), it returns NULL. Also, it takes in By-Reference a Node Pointer parameter named previous, and sets it to the Address of the target Node's predecessor element (also a Node) If the scarch fails, or if the target element is found within the first Node of the list and has no predecessor, previous should be set to NULL. (Extra Functionality (not required for 100pt grade): The method also takes in a Node Pointer named start, which indicates where it should start searching in the list. If this is passed as NULL, it denotes to start scarching from the first clement (Nodc). Otherwisc, this can be used to start a recursive search in case an element exists twice (otherwise Find will always rcturn the first clement's address) ) InsertAfter first finds the element (a Node) that contains DataType target, and then inserts after it a new element (a Node) that holds the value DataType value. Returns a Node Pointer to the element (a Node) it inserted (or NULL if it failed). Note. Try to think through what you are doing, and sketch out how it's going to work. Think of all possible cases, e.g. inserting in the middle, at the end, in the start, etc. (10) InsertBefore first finds the element (a Node) that contains DataType target, and then inserts before it a new element (a Node) that holds the value DataType value. Returns a Node Pointer to the element (a Node) it inserted (or NULL if it failed). Note: Try to think through what you are doing, and skctch out how it's going to work. Think of all possible cases, e.g. inserting in the middle, at the end, in the start, etc. (11) Erase first finds the element (a Node) that contains DataType target, and then removes it from the list. Returns a Node Pointer to the element (a Node) right after the one it just removed (if the last it removed was the last Node in the list, it should return NULL). Note. Try to think through what you are doing, and sketch out how it's going to work. Think of all sible cases, e.g. removing in the middle, the first element, the pos emen >(12a,12b) operator (const and non-const qualified) will allow by-Referenc g of a specific Data'Type object within a Node at an index size t position within the list. Note. Since this is not an Array-based implementation, the size_t position index is a "fake index", just an ch that position:0 corresponds to the first element (a Node) in the list cremental valu nd each subs (15) size will return the size of the current list. Note: Since this is not an Array-based implementation, the function has to traverse the list to find how many elements (Nodes) are contained wit (16) empty will rcturn a bool, truc if the list is cmpty, and falsc othcrwis ement correspon ds posi (17) clear will clear the contents of the list, so a s call it will be an empty list ob Note: Doe s this need to perform memory deallocatStep by Step Solution
There are 3 Steps involved in it
Step: 1
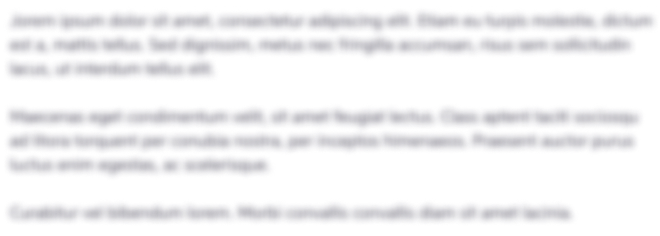
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started