Question
Need help with pokedex.cpp, and string summary in pokemon .cpp. Here, youll implement a fullfeatured Pokdex that contains a collection of Pokmon. Pokmon can be
Need help with pokedex.cpp, and string summary in pokemon .cpp. Here, youll implement a fullfeatured Pokdex that contains a collection of Pokmon. Pokmon can be added, removed, and searched for, and the entire Pokdex can be saved to or loaded from a file. There are two parts: creating a Pokmon.cpp file, and creating a Pokdex.cpp file.
The following files have been given to you, rememeber that only the pokemon.cpp and pokedex.cpp are to be made. Main.cpp and both .h files are given and not to be changed, unless the header files need to to be included. Only use very basic c++ statements, very basic syntax.
1. A C++ header file (pokemon.h) declaring the Pokemon class.
#ifndef POKEMON_H #define POKEMON_H #includeusing namespace std; class Pokemon { public: enum Type {Normal, Fighting, Flying, Poison}; // A summary string is a single string that contains all of a Pokemon's information. // A Pokemon with one type and Ndex 1 has a summary string of the form: // "Name, #001, type1," // Similarly, a Pokemon with two types and Ndex 2 has a summary string of the form: // "Name, #002, type1, type2," // Initializes a Pokemon from a summary string Pokemon(string summary); // Returns the summary string of the Pokemon string summary(); Pokemon(string name, int ndex, Type type1); Pokemon(string name, int ndex, Type type1, Type type2); string name(); int Ndex(); Type type1(); bool is_multitype(); Type type2(); float damage_multiplier(Type attack_type); private: int _ndex; // Stores the Pokemon's Ndex string _name; // Stores the Pokemon's name Type types[2]; // Stores the Pokemon's types (1 or 2 of them) int type_count; // Stores how many types the Pokemon has }; // Returns a string corresponding to the type. Examples: // 1. type_to_string(Pokemon::Poison) returns "Poison". // 2. type_to_string(Pokemon::Normal) returns "Normal". string type_to_string(Pokemon::Type t); // Returns the type corresponding to a string. Examples: // 1. string_to_type("Poison") returns Pokemon::Poison. // 2. string_to_type("Normal") returns Pokemon::Normal. // 3. Allowed to return anything if given string doesn't // correspond to a Pokemon type. Pokemon::Type string_to_type(string s); #endif
2. A C++ header file (pokedex.h) declaring the Pokedex class.
#ifndef POKEDEX_H #define POKEDEX_H #include#include "pokemon.h" using namespace std; class Pokedex { public: // A file containing a Pokedex should contain a line // for each Pokemon in the Pokedex. This line // should be the summary string of the Pokemon. // See pokemon.txt for an example. // Constructs an empty pokedex. Pokedex(); // Constructs a Pokedex with the Pokemon found in // the specified file. Pokedex(string filename); // Writes the Pokedex to the file. void save(string filename); // Adds a pokemon to the pokedex. void add(Pokemon* p); // Removes a pokemon from the pokedex. void remove(Pokemon* p); // Returns a (pointer to a) pokemon in the pokedex with the given name. // If none exists, returns nullptr. // // Hint: loop through all of A, searching for a Pokemon with // the given name. Return the first one found. Pokemon* lookup_by_name(string name); // Returns a (pointer to a) pokemon in the pokedex with the given name. // If none exists, returns nullptr. // // Hint: look in A[ndex]. Pokemon* lookup_by_Ndex(int ndex); // Returns the number of pokemon in the pokedex. int size(); private: // The pokedex is represented as an array of Pokemon pointers. // // Hint: // 1. Initialize the values in A to nullptr. // 2. When adding a Pokemon('s pointer), store the pointer in // the array index equal to the Pokemon's Ndex. // 3. When removing a Pokemon('s pointer), do so by setting the // corresponding array index equal to nullptr. Pokemon* A[1000]; }; #endif
3. A C++ source file (main.cpp) containing a main function with tests.
#include#include #include #include #include #include "pokedex.h" using namespace std; inline void _test(const char* expression, const char* file, int line) { cerr name() == mankey.name()); test(p->Ndex() == mankey.Ndex()); test(p->type1() == mankey.type1()); test(p->is_multitype() == mankey.is_multitype()); p = D2.lookup_by_name("Tornadus"); test(p != nullptr); test(p->Ndex() == tornadus.Ndex()); p = D2.lookup_by_name("Grimer"); test(p != nullptr); test(p->Ndex() == grimer.Ndex()); p = D2.lookup_by_name("Timburr"); test(p != nullptr); test(p->Ndex() == timburr.Ndex()); p = D2.lookup_by_name("Tonkamon"); test(p != nullptr); test(p->Ndex() == tonkamon.Ndex()); p = D2.lookup_by_name("Pidgey"); test(p != nullptr); test(p->name() == pidgey.name()); test(p->Ndex() == pidgey.Ndex()); test(p->type1() == pidgey.type1()); test(p->is_multitype() == pidgey.is_multitype()); test(p->type2() == pidgey.type2()); p = D2.lookup_by_name("Fletchling"); test(p != nullptr); test(p->Ndex() == fletchling.Ndex()); test(D2.lookup_by_name("Zubat") == nullptr); p = D2.lookup_by_name("Toxicroak"); test(p != nullptr); test(p->Ndex() == toxicroak.Ndex()); p = D2.lookup_by_name("Golbat"); test(p != nullptr); test(p->Ndex() == golbat.Ndex()); test(D2.lookup_by_name("Hoothoot") == nullptr); D2.add(&bouffalant); D2.add(&zubat); D2.add(&hoothoot); D2.remove(&mankey); D2.remove(&toxicroak); test(D2.size() == 10); // Remove file made during testing remove("./test_pokedex1.txt"); // Delete old version of test_pokedex2.txt if it exists remove("./test_pokedex2.txt"); // Save Bouffalant, Tornadus, Grimer, Timburr, Tonkamon, // Pidgey, Fletchling, Zubat, Golbat, Hoothoot D2.save("./test_pokedex2.txt"); // Create a Pokedex with: // Bouffalant, Tornadus, Grimer, Timburr, Tonkamon, // Pidgey, Fletchling, Zubat, Golbat, Hoothoot Pokedex D3("./test_pokedex2.txt"); test(D3.size() == 10); p = D3.lookup_by_name("Bouffalant"); test(p->name() == bouffalant.name()); test(p->Ndex() == bouffalant.Ndex()); test(p->type1() == bouffalant.type1()); test(p->is_multitype() == bouffalant.is_multitype()); test(D3.lookup_by_name("Mankey") == nullptr); p = D3.lookup_by_name("Tornadus"); test(p != nullptr); test(p->Ndex() == tornadus.Ndex()); p = D3.lookup_by_name("Grimer"); test(p != nullptr); test(p->Ndex() == grimer.Ndex()); p = D3.lookup_by_name("Timburr"); test(p != nullptr); test(p->Ndex() == timburr.Ndex()); p = D3.lookup_by_name("Tonkamon"); test(p != nullptr); test(p->Ndex() == tonkamon.Ndex()); p = D3.lookup_by_name("Pidgey"); test(p != nullptr); test(p->name() == pidgey.name()); test(p->Ndex() == pidgey.Ndex()); test(p->type1() == pidgey.type1()); test(p->is_multitype() == pidgey.is_multitype()); test(p->type2() == pidgey.type2()); p = D3.lookup_by_name("Fletchling"); test(p != nullptr); test(p->Ndex() == fletchling.Ndex()); p = D3.lookup_by_name("Zubat"); test(p != nullptr); test(p->Ndex() == zubat.Ndex()); p = D3.lookup_by_name("Golbat"); test(p != nullptr); test(p->Ndex() == golbat.Ndex()); test(D3.lookup_by_name("Toxicroak") == nullptr); p = D3.lookup_by_name("Hoothoot"); test(p != nullptr); test(p->Ndex() == hoothoot.Ndex()); // Remove file made during testing remove("./test_pokedex2.txt"); ifstream f; f.open("./pokedex.txt"); assert(f.is_open()); // If this fails, you're missing pokedex.txt f.close(); Pokedex D4("./pokedex.txt"); test(D4.size() == 125); p = D4.lookup_by_name("Staraptor"); test(p != nullptr); test(p->name() == "Staraptor"); test(p->Ndex() == 398); test(p->type1() == Pokemon::Normal); test(p->is_multitype()); test(p->type2() == Pokemon::Flying); p = D4.lookup_by_Ndex(143); test(p != nullptr); test(p->name() == "Snorlax"); test(p->Ndex() == 143); test(p->type1() == Pokemon::Normal); test(!p->is_multitype()); test(D4.lookup_by_name("Pikachu") == nullptr); test(D4.lookup_by_Ndex(25) == nullptr); cout 4. A text file (pokedex.txt) containing a list of all normal, fighting, flying, and poison Pokemon, one per line, in the summary string format described in pokemon.h.Reading and writing to files is done using the library, specifically the ofstream and ifstream classes. Objects of these classes work roughly like cout and cin, but for files instead of the terminal.
Aipom, #190, Normal, Ambipom, #424, Normal, Arbok, #024, Poison, Arceus, #493, Normal, Audino, #531, Normal, Bidoof, #399, Normal, Blissey, #242, Normal, Bouffalant, #626, Normal, Braviary, #628, Normal, Flying, Buneary, #427, Normal, Bunnelby, #659, Normal, Castform, #351, Normal, Chansey, #113, Normal, Chatot, #441, Normal, Flying, Cinccino, #573, Normal, Conkeldurr, #534, Fighting, Croagunk, #453, Poison, Fighting, Crobat, #169, Poison, Flying, Delcatty, #301, Normal, Ditto, #132, Normal, Dodrio, #085, Normal, Flying, Doduo, #084, Normal, Flying, Dunsparce, #206, Normal, Eevee, #133, Normal, Ekans, #023, Poison, Exploud, #295, Normal, Farfetch'd, #083, Normal, Flying, Fearow, #022, Normal, Flying, Fletchling, #661, Normal, Flying, Furfrou, #676, Normal, Furret, #162, Normal, Garbodor, #569, Poison, Glameow, #431, Normal, Golbat, #042, Poison, Flying, Grimer, #088, Poison, Gulpin, #316, Poison, Gurdurr, #533, Fighting, Happiny, #440, Normal, Hariyama, #297, Fighting, Hawlucha, #701, Fighting, Flying, Herdier, #507, Normal, Hitmonchan, #107, Fighting, Hitmonlee, #106, Fighting, Hitmontop, #237, Fighting, Hoothoot, #163, Flying, Normal, Kangaskhan, #115, Normal, Kecleon, #352, Normal, Koffing, #109, Poison, Lickilicky, #463, Normal, Lickitung, #108, Normal, Lillipup, #506, Normal, Linoone, #264, Normal, Lopunny, #428, Normal, Loudred, #294, Normal, Machamp, #068, Fighting, Machoke, #067, Fighting, Machop, #066, Fighting, Makuhita, #296, Fighting, Mankey, #056, Fighting, Meowth, #052, Normal, Mienfoo, #619, Fighting, Mienshao, #620, Fighting, Miltank, #241, Normal, Minccino, #572, Normal, Muk, #089, Poison, Munchlax, #446, Normal, Nidoran, #029, Poison, Nidoran, #032, Poison, Nidorina, #030, Poison, Nidorino, #033, Poison, Noctowl, #164, Normal, Flying, Pancham, #674, Fighting, Patrat, #504, Normal, Persian, #053, Normal, Pidgeot, #018, Normal, Flying, Pidgeotto, #017, Normal, Flying, Pidgey, #016, Normal, Flying, Pidove, #519, Normal, Flying, Porygon, #137, Normal, Porygon-Z, #474, Normal, Porygon2, #233, Normal, Primeape, #057, Fighting, Purugly, #432, Normal, Raticate, #020, Normal, Rattata, #019, Normal, Regigigas, #486, Normal, Riolu, #447, Fighting, Rufflet, #627, Normal, Flying, Sawk, #539, Fighting, Sentret, #161, Normal, Seviper, #336, Poison, Skitty, #300, Normal, Slaking, #289, Normal, Slakoth, #287, Normal, Smeargle, #235, Normal, Snorlax, #143, Normal, Spearow, #021, Normal, Flying, Spinda, #327, Normal, Stantler, #234, Normal, Staraptor, #398, Normal, Flying, Staravia, #397, Normal, Flying, Starly, #396, Normal, Flying, Stoutland, #508, Normal, Swablu, #333, Normal, Flying, Swalot, #317, Poison, Swellow, #277, Normal, Flying, Taillow, #276, Normal, Flying, Tauros, #128, Normal, Teddiursa, #216, Normal, Throh, #538, Fighting, Timburr, #532, Fighting, Tornadus, #641, Flying, Toxicroak, #454, Poison, Fighting, Tranquill, #520, Normal, Flying, Trubbish, #568, Poison, Tyrogue, #236, Fighting, Unfezant, #521, Normal, Flying, Ursaring, #217, Normal, Vigoroth, #288, Normal, Watchog, #505, Normal, Weezing, #110, Poison, Whismur, #293, Normal, Zangoose, #335, Normal, Zigzagoon, #263, Normal, Zubat, #041, Poison, Flying,Pokemon**A 1000 41 56 Pokemon* array Ivysaur 002 Poison "Mankey" 056 "Zubat" 041 Poison Flying 2 Fighting Pokemon objects Figure 1: Storing Pokmon pointers in the Pokdex
Step by Step Solution
There are 3 Steps involved in it
Step: 1
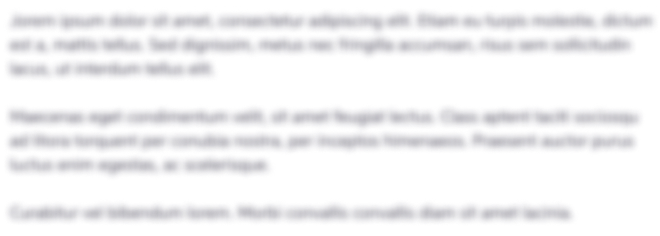
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started