Question
Need help with the bolded portions of this program. No changes to any other parts of the program other than the bolded sections and parts.
Need help with the bolded portions of this program. No changes to any other parts of the program other than the bolded sections and parts.
main.cpp
#include
const int LEN = 3; //the length of words const int ALPHABET = 26; //total number of letters
class Node { friend class LL; private: string el; Node* next; public: Node(const string& s) { el = s; next = NULL; } string getElem() const { return el; } };
class LL { private: Node* front; Node* rear; int num; public: LL() { front = rear = NULL; num = 0; } ~LL(); bool empty()const { return (front == NULL && rear == NULL && num == 0); } void addRear(const string& s); void addRear(Node* p); void append(const LL& other); void clear() { front = rear = NULL; num = 0; } Node* begin() const { return front; } Node* goToNext(Node* curr) const { return curr->next; } int size() const { return num; } };
LL::~LL() { cout << "destructor is called" << endl; Node* p = front; while (!empty()) { front = front->next; delete p; p = front; if (front == NULL) rear = NULL; num--; } }
//add a new node at the end of this list. The element of the new node is s. void LL::addRear(const string& s) { Node* p = new Node(s); //the new node has NULL in the next field.
if (empty()) front = rear = p; else { rear->next = p; rear = rear->next; } num++; }
//add the node pointed to by p (p points to one of the nodes in all list) at the end of this list void LL::addRear(Node* p) { //Don't make a new node.
//You need a special case when the list is empty
//Don't forget to place NULL in the next field of the rear node of this list.
//don't forget to increment num
//Note: this function should have complexity O(1). There is no loop required. }
//appending another linked list at the end of this linked list void LL::append(const LL& other) { //Do you need to think about a special case?
//Don't forget to update num of this list
//Note: this function should have complexity O(1). There is no loop required. }
void radixSort(LL& all); void printLL(const LL& l); void checkBuckets(const LL buckets[], int n); void combineLists(/* ?????????? */); void makeLL(LL& all);
int main() { LL all; //holds all words makeLL(all); //all contains strings in the original order radixSort(all); cout << "Final result ----" << endl; printLL(all); //all contains strings in sorted sorter cout << endl;
return 0; }
//using the radix sort algorithm to sort strings that contain lowercase letters. void radixSort(LL& all) { //Each slot of the buckets array is a LL object. //??? buckets[ALPHABET]; //bucket[0] is for 'a'. There are 26 buckets.
//checking each place //To go through each string in the all list, check printLL() to see how to set an iterator to the first node and move it to the next node. //I used a while loop to iterate the all list. //Go to the correct bucket depending on the letter at the current place and add the current node to the end of the bucket }
//combining all lists from buckets void combineLists(/* all list, buckets */) { //??????? //call clear() to reset the all list. All the nodes went to correct buckets already. //populate the all list by combining the lists from the buckets by calling append() //??????? //reset each list in b[i] by calling clear(). All the nodes were moved to the all list already. }
//Make a linked list of words from an input file void makeLL(LL& all) { string s; fstream fin; fin.open("radix.in"); if (!fin) { cout << "The input file doesn't exist" << endl; exit(1); //quits the program if the input program doesn't exist. }
fin >> s; while (fin) { all.addRear(s); fin >> s; } }
//This function shows the contents of all the buckets void checkBuckets(const LL buckets[], int n) { for (int i = 0; i < n; i++) { cout << i << ": "; printLL(buckets[i]); cout << endl; } }
//This function prints all the elements in l void printLL(const LL& l) { //iterate p from the first node to last node for (Node* p = l.begin(); p != NULL; p = l.goToNext(p)) cout << p->getElem() << ' '; }
radix.in
xdg sai aow fns als ier pls neo wix akw roc arn own eic alw pqh ith six dir dka eiq pdo wke fnc eia pod
Step by Step Solution
There are 3 Steps involved in it
Step: 1
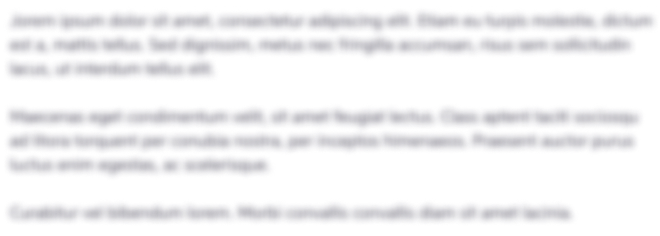
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started