Question
Need help with the following java code, I have most of the code done but i have some minor problems getting the correct output. Most
Need help with the following java code, I have most of the code done but i have some minor problems getting the correct output. Most of the output is correct but some conversions are being converted incorrectly and need help fixing the code to solve these minor issues. I also need help revising my code and fixing it to meet the required instructions in case i missed any of the requirements needed to complete this lab.
// Driver class
import java.util.Scanner;
import java.io.*;
public class Driver {
public static void main(String[] args) throws IOException
{
int choice;
PrintWriter pw = new PrintWriter(new FileWriter("csis.txt"));
Decimal dec = new Decimal(pw);
Binary bin = new Binary(pw);
HexaDecimal hex = new HexaDecimal(pw);
Menu menu = new Menu(pw);
do
{
menu.display();
choice = menu.getSelection();
switch (choice)
{
case 1 : dec.decToBin();
break;
case 2 : dec.decToHex();
break;
case 3 : bin.binToDec();
break;
case 4 : bin.binToHex();
break;
case 5 : hex.hexToDec();
break;
case 6 : hex.hexToBin();
break;
}
} while (choice != 7);
pw.close();
}
}
// Menu class
import java.io.*;
import java.util.*;
import java.util.Scanner;
public class Menu
{
//Declare Variable
private PrintWriter pw;
public Menu (PrintWriter pw)
{
this.pw = pw;
}
public void display()
{
//Display Conversion
System.out.println("Please Choose a Conversion: ");
System.out.println(" 1. Decimal to Binary: ");
System.out.println(" 2. Decimal to Hexadecimal: ");
System.out.println(" 3. Binary to Decimal: ");
System.out.println(" 4. Binary to Hexadecimal: ");
System.out.println(" 5. Hexadecimal to Decimal: ");
System.out.println(" 6. Hexadecimal to Binary: ");
System.out.println(" 7. Exit: ");
pw.println();
pw.println("Please Choose a Conversion: ");
pw.println(" 1. Decimal to Binary: ");
pw.println(" 2. Decimal to Hexadecimal: ");
pw.println(" 3. Binary to Decimal: ");
pw.println(" 4. Binary to Hexadecimal: ");
pw.println(" 5. Hexadecimal to Decimal: ");
pw.println(" 6. Hexadecimal to Binary: ");
pw.println();
}
public int getSelection()
{
//Create Scanner
Scanner menuClass = new Scanner(System.in);
//Declare Variable
int choice = 0;
//Display Choice
System.out.println();
System.out.println("Choice: ");
pw.println("Choice: ");
choice = menuClass.nextInt();
//Display User's Input
System.out.println();
System.out.println("You've Entered " + choice);
System.out.println();
pw.println("You've Entered " + choice);
pw.println();
return choice;
}
}
// Binary class
import java.lang.*;
import java.io.*;
import java.util.Scanner;
public class Binary
{
// Variables
private PrintWriter pw;
String hex = "";
int exp = 0;
int dec = 0;
int temp;
String inputBinToDec;
int i = 0;
public Binary (PrintWriter pw)
{
this.pw = pw;
}
public void binToDec()
{
inputBin();
toDec();
outDec();
}
public void binToHex()
{
inputBin();
toHex();
outHex();
}
private void inputBin()
{
//Declare Variables
dec = 0;
hex = "";
//Creates Scanner
Scanner inputSCB = new Scanner (System.in);
//User Enters Binary Number
System.out.println("Enter Binary Number:");
pw.println("Enter Binary Number:");
String bin = inputSCB.nextLine();
pw.print(bin);
pw.println();
inputBinToDec = bin;
//inputBinToDec = bin;
}
private void toDec()
{
int intValue;
int length = inputBinToDec.length();
int exp = inputBinToDec.length();
for (int i = 0; i
{
intValue = Character.getNumericValue(inputBinToDec.charAt(i));
dec += ((intValue)*Math.pow(2, --exp));
}// end for
}
private void outDec()
{
// Display Binary to Decimal
System.out.println();
System.out.println("Binary to Decimal: ");
System.out.println(dec + " ");
// Sends Output File to csis.text
pw.println("Binary to Decimal: ");
pw.print(dec + " ");
pw.println();
}
private void toHex()
{
// Variables
int decToHex;
int decNum;
int intValue;
int length = inputBinToDec.length();
int twoPower = inputBinToDec.length();
for (int i = 0; i
{
// Converts from String to Charcater
intValue = Character.getNumericValue(inputBinToDec.charAt(i));
dec += ((intValue)*Math.pow(2, --exp));
}
// Assign New Variables
decNum = dec;
String convHex;
while (decNum > 0)
{
// Formula to Convert Binary to Hexadecimal
decToHex = decNum % 16;
decNum = decNum / 16;
convHex = Integer.toString(decToHex);
switch (decToHex)
{
case 10: convHex = "A";
break;
case 11: convHex = "B";
break;
case 12: convHex = "C";
break;
case 13: convHex = "D";
break;
case 14: convHex = "E";
break;
case 15: convHex = "F";
break;
}
hex += convHex;
}
}
private void outHex()
{
// Display Binary to Hexadecimal
System.out.println();
System.out.println("Binary to Hexadecimal: ");
System.out.println(hex + " ");
// Sends Output File to csis.text
pw.println("Binary to Hexadecimal: ");
pw.print(hex+ " ");
pw.println();
}
}
// Decimal Class
import java.lang.*;
import java.io.*;
import java.util.Scanner;
public class Decimal
{
//Declare Variables
private PrintWriter pw;
int inputDec = 0;
int inputDecToBin;
int inputDecToHex;
String bin = " ";
String hex = " ";
public Decimal (PrintWriter pw)
{
this.pw = pw;
}
public void decToBin()
{
inputDec();
toBin();
outBin();
}
public void decToHex()
{
inputDec();
toHex();
outHex();
}
private void inputDec()
{
//Declare Variable
bin = " ";
//Create Scanner
Scanner scanner = new Scanner(System.in);
//User Enters Decimal Number
System.out.println("Please Enter a Decimal Number: ");
String dec = scanner.nextLine();
pw.println("Please Enter a Decimal Number: ");
pw.println(dec);
//Changes Back from String to Integer
inputDec = Integer.parseInt(dec);
}
private void toBin()
{
while(inputDec > 0)
{
//Formula to Convert Decimal to Binary
inputDecToBin = inputDec % 2;
inputDec = inputDec / 2;
bin += inputDecToBin;
}
}
private void outBin()
{
//Display Decimal to Binary
System.out.println();
System.out.println("Decimal to Binary: ");
System.out.println(new StringBuffer (bin).reverse().toString());
System.out.println();
//Sends Output File to csis.txt
pw.println("Decimal to Binary: ");
pw.print(new StringBuffer (bin).reverse().toString());
pw.println();
}
private void toHex()
{
//Declare Variable
hex = "";
do
{
//Formula to Convert Decimal to Binary
inputDecToHex = inputDec % 16;
if (inputDecToHex == 10)
{
hex = 'A' + hex;
}
else if (inputDecToHex == 11)
{
hex = 'B' + hex;
}
else if (inputDecToHex == 12)
{
hex = 'C' + hex;
}
else if (inputDecToHex == 13)
{
hex = 'D' + hex;
}
else if (inputDecToHex == 14)
{
hex = 'E' + hex;
}
else if (inputDecToHex == 15)
{
hex = 'F' + hex;
}
else
{
hex = Integer.toString(inputDec % 16) + hex;
}
inputDec = inputDec / 16;
}while (inputDec > 0);
}
private void outHex()
{
//Display Decimal to Hexadecimal
System.out.println();
System.out.println("Decimal to Hexadecimal: ");
System.out.println(new StringBuffer(hex).reverse() + " ");
//Sends Output File to csis.text
pw.println("Decimal to Hexadecimal: ");
pw.print(new StringBuffer(hex).reverse()+" ");
pw.println();
//Closes the Output File
//pw.close();
}
}
// HexaDecimal Class
import java.lang.*;
import java.io.*;
import java.util.Scanner;
public class HexaDecimal
{
// Declare Variables
private PrintWriter pw;
int dec = 0;
String hexadecimal = "";
String bin = "";
String inputHex;
String inputBinToHex = "";
public HexaDecimal (PrintWriter pw)
{
this.pw = pw;
}
public void hexToDec()
{
inputHex();
toDec();
outDec();
}
public void hexToBin()
{
inputHex();
toBin();
outBin();
}
private void inputHex()
{
//Creates Scanner
Scanner inputHex = new Scanner(System.in);
// User Enters Hexadecimal Number
System.out.println("Please Enter a Hexadecimal Number:" );
hexadecimal = inputHex.nextLine();
// Sends OutputFile to the csis.txt
pw.println("Please Enter a Hexadecimal: ");
pw.println(hexadecimal);
}
private void toDec()
{
// Declare Variables
int h = hexadecimal.length() - 1;
int n = 0;
for(int i=0; i
{
char ch= hexadecimal.charAt(i);
switch(ch)
{
case '1': n=1;
break;
case '2': n=2;
break;
case '3': n=3;
break;
case '4': n=4;
break;
case '5': n=5;
break;
case '6': n=6;
break;
case '7': n=7;
break;
case '8': n=8;
break;
case '9': n=9;
break;
case 'A': n=10;
break;
case 'B': n=11;
break;
case 'C': n=12;
break;
case 'D': n=13;
break;
case 'E': n=14;
break;
case 'F':n=15;
break;
}
// Formula to Convert Hexadecimal to Decimal
dec = (int)(n*(Math.pow(16,h))) + (int)dec;
h--;
}
}
private void outDec()
{
// Display the Hexadecimal to Decimal
System.out.println();
System.out.println("Hexadecimal to Decimal: ");
pw.println("Hexadecimal to Decimal: ");
System.out.println(dec+" ");
pw.print(dec+" ");
pw.println();
}
//Converts the hexadecimal value to a decimal number
private void toBin()
{
//Declare Variables
int intValue = 0;
double intPreFinal = 0;
double intFinal;
int Binary = 0;
int PreFinal = 0;
int hex;
bin = "";
int intStringLength = hexadecimal.length();
int exp = intStringLength;
for (int i = 0; i
{
// Converts from String to Integer
hex = Integer.parseInt(""+hexadecimal.charAt(i),16);
// Formula to Convert from Hexadecimal to Binary
intValue = Character.getNumericValue(hexadecimal.charAt(i));
intPreFinal = intPreFinal + ((intValue)*Math.pow(16, --exp));
}
// Assigning New Variables
intFinal = intPreFinal;
int intTest = (int)intPreFinal;
double grand = intTest;
int j = 0;
for(int i = 0;intTest >= Math.pow(2,i);i++)
{
j = i;
}
for(;j >= 0;j--)
{
Binary = 0;
// Reverses the Order
if (grand >= Math.pow(2,j))
Binary = 1;
grand = grand - Math.pow(2,j) * Binary;
bin += Integer.toString(Binary);
}
}
private void outBin()
{
// Display Hexadecimal to Binary
System.out.println();
System.out.println("Hexadecimal to Binary: ");
System.out.println(bin + " ");
// Sends Output File to csis.text
pw.println("Hexadecimal to Binary: ");
pw.print(bin+ " ");
pw.println();
// Close Output File
pw.close();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
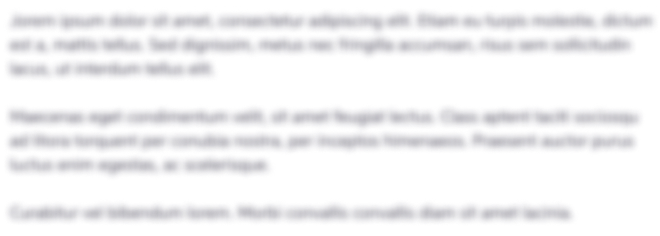
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started