Question
Need help with the JAVA project, below are my question and the code with 5 classes that I have done with another similar project. Please
Need help with the JAVA project, below are my question and the code with 5 classes that I have done with another similar project.
Please help.
Main class is:
package ProductIO;
import java.util.*;
import ProductIO.ProductIO;
public class Main
{
public static void main(String[] args)
{
System.out.println("Welcome to the Product manager");
displaymenu();
//perform 1 or more action
String action = "";
while(!action.equalsIgnoreCase("exit"))
{
//get the input from the user
action = Console.getString("Enter a command: ");
System.out.println();
if(action.equalsIgnoreCase("list"))
{
displayAllProducts();
}
else if(action.equalsIgnoreCase("add"))
{
addProduct();
}
else if(action.equalsIgnoreCase("del") || action.equalsIgnoreCase("delete"))
{
deleteProduct();
}
else if(action.equalsIgnoreCase("help") || action.equalsIgnoreCase("menu"))
{
displaymenu();
}
else if(action.equalsIgnoreCase("exit"))
{
System.out.println("Bye. ");
}
else
{
System.out.println("Please enter vaule command.");
}
}
}
public static void displaymenu()
{
System.out.println("Command Menu");
System.out.println("List - List all products");
System.out.println("add - add a product");
System.out.println("del - delete a product");
System.out.println("help - show this menu");
System.out.println("exit - exit this application");
}
public static void displayAllProducts()
{
System.out.println("Product List");
List
if(products == null)
{
System.out.println("Error unable to get products. ");
}
else
{
Product p;
StringBuilder sb = new StringBuilder();
for(Product product : products)
{
p=product;
sb.append(StringUtil.pad(p.getCode(),12));
sb.append(StringUtil.pad(p.getDescription(),34));
sb.append(p.getPriceFormatted());
sb.append(" ");
}
System.out.println(sb.toString());
}
}
public static void addProduct()
{
String code = Console.getString("Enter a product code: ");
String decription = Console.getString("Enter a decription: ");
double price = Console.getDouble("Enter price: ");
Product product = new Product();
product.setCode(code);
product.setDescription(decription);
product.setPrice(price);
ProductIO.add(product);
System.out.println(" " + decription + "was added to the database. ");
}
public static void deleteProduct()
{
String code = Console.getString("Enter a product code to delete: ");
Product product = ProductIO.get(code);
if(product ==null)
{
System.out.println("Error.");
}
else
{
ProductIO.delete(product);
}
System.out.println(" " + product.getDescription() + "was deleted to the database. ");
}
}
-------------------------------------------------
Console class:
package ProductIO;
import java.util.*;
import java.util.Scanner;
public class Console
{
static Scanner keyboard= new Scanner(System.in);
public static int getInt(String prompt)
{
int i=0;
while(true)
{
System.out.println(prompt);
try
{
i=Integer.parseInt(keyboard.nextLine());
break;
}
catch(NumberFormatException e)
{
System.out.println("Error invalid integer. Try again.");
}
}
return i;
}
public static String getString(String prompt)
{
System.out.print(prompt);
String s=keyboard.nextLine();
return s;
}
public static double getDouble(String prompt)
{
double i=0.0;
while(true)
{
System.out.print(prompt);
try
{
i=Double.parseDouble(keyboard.nextLine());
break;
}
catch(NumberFormatException e)
{
System.out.println("Error invalid Double. Try again. ");
}
}
return i;
}
}
------------------------------------------
Product class:
package ProductIO;
import java.text.*;
public class Product
{
private String code;
private String description;
private double price;
public Product()
{
code="";
description="";
price=0.00;
}
public void setCode(String code)
{
this.code=code;
}
public String getCode()
{
return code;
}
public void setDescription(String description)
{
this.description=description;
}
public String getDescription()
{
return description;
}
public void setPrice(double price)
{
this.price=price;
}
public double getPrice()
{
return price;
}
public String getPriceFormatted()
{
NumberFormat currency=NumberFormat.getCurrencyInstance();
String priceFormatted=currency.format(price);
return priceFormatted;
}
}
-------------------------------------------------
ProductIO class:
package ProductIO;
import java.io.*;
import java.nio.*;
import java.nio.file.*;
import java.util.*;
public class ProductIO
{
private static final Path productsPath = Paths.get("Products.txt");
private static final File productsFile = productsPath.toFile();
private static final String FIELD_SEP ="\t";
private static List
//constructor
private ProductIO()
{
}
public static List
{
if(products !=null)
{
return products;
}
products = new ArrayList();
if(Files.exists(productsPath))
{
try(BufferedReader in = new BufferedReader(new FileReader(productsFile)))
{
//read all products stored in the file in to the array list
String line=in.readLine();
while(line !=null)
{
String[]columns = line.split(FIELD_SEP);
String code = columns[0];
String description = columns[1];
String price = columns[2];
Product p=new Product();
p.setCode(code);
p.setDescription(description);
p.setPrice(Double.parseDouble(price));
products.add(p);
line= in.readLine();
}
}
catch (IOException e)
{
System.out.println(e);
return null;
}
}
return products;
}
public static Product get(String code)
{
for(Product p : products)
{
if(p.getCode().equals(code))
return p;
}
return null;
}
private static boolean saveAll()
{
try(PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter(productsFile))))
{
//write all products in the array list to the file
for(Product p : products)
{
out.print(p.getCode()+FIELD_SEP);
out.print(p.getDescription()+FIELD_SEP);
out.print(p.getPrice());
}
}
catch(IOException e)
{
System.out.println(e);
return false;
}
return true;
}
public static boolean add(Product p)
{
products.add(p);
return saveAll();
}
public static boolean delete(Product p)
{
products.remove(p);
return saveAll();
}
public static boolean update(Product newProduct)
{
Product oldProduct = get(newProduct.getCode());
int i= products.indexOf(oldProduct);
products.remove(i);
products.add(i,newProduct);
return saveAll();
}
}
----------------------------------------------------------------------------
StringUtil class:
package ProductIO;
public class StringUtil
{
public static String pad(String s, int length)
{
if(s.length() { StringBuilder sb=new StringBuilder(s); while(sb.length() { sb.append(" "); } return sb.toString(); } else { return s.substring(0,length); } } public static String spad(String s, int length) { if(s.length() { StringBuilder sb=new StringBuilder(s); while(sb.length() { sb.insert(0, " "); } return sb.toString(); } else { return s.substring(0,length); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
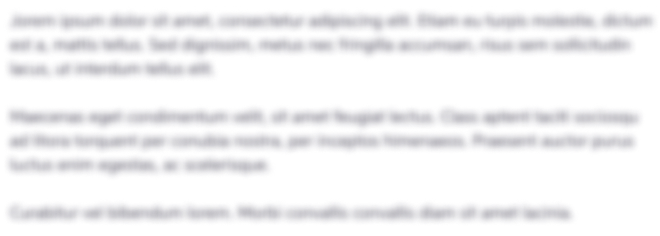
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started