Question
Need help with this code. I need this code to compile and run. Code: #include #include #include personType.h using namespace std; const int NO_OF_REGIONS =
Need help with this code.
I need this code to compile and run.
Code:
#include
#include
#include "personType.h"
using namespace std;
const int NO_OF_REGIONS = 4;
class candidateType : public personType
{
public:
const candidateType& operator=(const candidateType&);
//Overload the assignment operator for objects of the
//type candidateType
const candidateType& operator=(const personType&);
//Overload the assignment operator for objects so that
//the value of an object of type personType can be
//assigned to an object of type candidateType
void updateVotesByRegion(int region, int votes);
//Function to update the votes of a candidate for a
//particular region.
//Postcondition: Votes for the region specified by the
// parameter are updated by adding the votes specified
// by the parameter votes.
void setVotes(int region, int votes);
//Function to set the votes of a candidate for a
//particular region.
//Postcondition: Votes for the region specified by the
// parameter are set to the votes specified by the
// parameter votes.
void calculateTotalVotes();
//Function to calculate the total votes received by a
//candidate.
//Postcondition: The votes in each region are added and
// assigned to totalVotes.
int getTotalVotes() const;
//Function to return the total votes received by a
//candidate.
//Postcondition: The value of totalVotes is returned.
void printData() const;
//Function to output the candidate's name, the votes
//received in each region, and the total votes received.
candidateType();
//Default constructor.
//Postcondition: Candidate's name is initialized to blanks,
// the number of votes in each region, and the total
// votes are initialized to 0.
//Overload the relational operators.
bool operator==(const candidateType& right) const;
bool operator!=(const candidateType& right) const;
bool operator<=(const candidateType& right) const;
bool operator<(const candidateType& right) const;
bool operator>=(const candidateType& right) const;
bool operator>(const candidateType& right) const;
private:
int votesByRegion[NO_OF_REGIONS]; //array to store the votes
// received in each region
int totalVotes; //variable to store the total votes
};
void candidateType::setVotes(int region, int votes)
{
votesByRegion[region - 1] = votes;
}
void candidateType::updateVotesByRegion(int region, int votes)
{
votesByRegion[region - 1] = votesByRegion[region - 1] + votes;
for (int i = 0; i totalVotes += votesByRegion[i]; //adding each region votes to totalVotes } void candidateType::calculateTotalVotes() { totalVotes = 0; for (int i = 0; i < NO_OF_REGIONS; i++) totalVotes += votesByRegion[i]; } int candidateType::getTotalVotes() const { return totalVotes; } void candidateType::printData() const { cout << left << setw(10) << firstName << " " << setw(10) << lastName << " "; cout << right; for (int i = 0; i < NO_OF_REGIONS; i++) cout << setw(7) << votesByRegion[i] << " "; cout << setw(7) << totalVotes << endl; } candidateType::candidateType() { for (int i = 0; i < NO_OF_REGIONS; i++) votesByRegion[i] = 0; totalVotes = 0; } bool candidateType::operator==(const candidateType& right) const { return (firstName == right.firstName && lastName == right.lastName); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
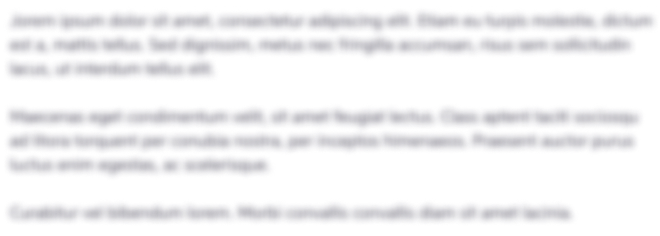
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started