Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need help with this code using C++ *********************************************************** // File Name: StringList.h // // // Represents a list of strings. // Supports adding strings to
Need help with this code using C++
***********************************************************
// File Name: StringList.h
//
//
// Represents a list of strings.
// Supports adding strings to the end of the list, and removing strings by
// index.
#include
using namespace std;
class StringList
{
public:
struct StringNode // the Nodes of the linked list
{
string data; // data is a string
StringNode *next; // points to next node in list
};
StringNode *head; // the head pointer
public:
StringList();
~StringList();
void add(string);
string remove(int);
};
************************************************************
// File Name: StringList.cpp
//
// Represents a list of strings.
// Supports adding strings to the end of the list, and removing strings by
// index.
#include
#include
using namespace std;
#include "StringList.h"
//***********************************************************
// StringList: constructs an empty list
//***********************************************************
StringList::StringList()
{
head = NULL;
}
//***********************************************************
// ~StringList: deallocates the nodes in the list
//***********************************************************
StringList::~StringList()
{
StringNode *p = head; //traversal pointer
StringNode *n; //saves the next node
while (p) {
n = p->next;
delete p;
p = n;
}
}
//***********************************************************
// add: adds a string to the end of the list
// str: the string to add
//***********************************************************
void StringList::add(string str)
{
StringNode *newNode; // points to the new node
newNode = new StringNode;
newNode->data = str;
newNode->next = NULL;
if (!head)
head = newNode;
else {
StringNode *p = head;
while (p->next)
p = p->next;
p->next = newNode;
}
}
//**************************************************************
// remove: takes an index of the node to remove from the list.
// returns true if the node was found and removed, else false.
//**************************************************************
string StringList::remove(int index) {
StringNode *p = head; // to traverse the list
StringNode *prev = NULL; // trailing node (there's no prev yet)
int i = 0;
while (p && i
prev = p;
p = p->next;
++i;
}
// index is found, set links + delete
if (p) {
string result = p->data;
if (prev==NULL) // (or p==head) p points to the first elem.
head = p->next;
else // prev points to the predecessor
prev->next = p->next;
delete p;
return result;
} else
return "";
}
*************************************************************
// File Name: ListDriver.cpp
//
//
// A demo driver for StringList.
//
#include
#include
using namespace std;
#include "Stack.h"
void testEmpty() {
cout
//testing StringList
Stack stack;
// testing on empty list
cout
}
void testSizeOne() {
cout
//testing StringList
Stack stack;
stack.push("Star Wars");
cout
cout
}
void testSizeTwo() {
cout
//testing StringList
Stack stack;
stack.push("Star Wars");
stack.push("Back to the Future");
cout
cout
cout
Stack: Given the class declaration of a Stack in the provided file Stack.h, use a stripped-down version of the StringList dass from Programming Assignment #5 (also provided in files StringList.h and StringList.cpp) to implement the stack operations. This means that in the definitions of the Stack functions you will need to call some of the StringList functions (add(str), remove(int) etc.). Hints: pick the appropriate end of the StringList to be the top of the stack, and keep track of the number of elements in the stack. Please inline your Stack function definitions (place the code in the Stack.h file). You may add private members to Stack.h DO NOT CHANGE StringList.h OR StringList.cpp!!! Test your implementation with the provided driver (StackDriver.cpp) Queue: Repeat the same exercise for the Queue: Use the class declaration in the provided file Queue.h, and use the same unchanged StringList dass files to implement the queue operations. Test your implementation with your own driver. Roller Coaster: Write a program that uses the Queue to simulate the management of the line of people waiting to ride a roller coaster in an amusement park. Here are the assumptions: The roller coaster seats x people. The riders come in groups that have a name and a size. The group size will not be more than x (the number of seats on the train). Groups cannot be split into separate runs of the roller coaster (all must go on the same train). Groups/people cannot jump ahead in line. A coaster train must be as full as possible before it can leave the station. Stack: Given the class declaration of a Stack in the provided file Stack.h, use a stripped-down version of the StringList dass from Programming Assignment #5 (also provided in files StringList.h and StringList.cpp) to implement the stack operations. This means that in the definitions of the Stack functions you will need to call some of the StringList functions (add(str), remove(int) etc.). Hints: pick the appropriate end of the StringList to be the top of the stack, and keep track of the number of elements in the stack. Please inline your Stack function definitions (place the code in the Stack.h file). You may add private members to Stack.h DO NOT CHANGE StringList.h OR StringList.cpp!!! Test your implementation with the provided driver (StackDriver.cpp) Queue: Repeat the same exercise for the Queue: Use the class declaration in the provided file Queue.h, and use the same unchanged StringList dass files to implement the queue operations. Test your implementation with your own driver. Roller Coaster: Write a program that uses the Queue to simulate the management of the line of people waiting to ride a roller coaster in an amusement park. Here are the assumptions: The roller coaster seats x people. The riders come in groups that have a name and a size. The group size will not be more than x (the number of seats on the train). Groups cannot be split into separate runs of the roller coaster (all must go on the same train). Groups/people cannot jump ahead in line. A coaster train must be as full as possible before it can leave the station cout
}
int main()
{
testEmpty();
testSizeOne();
testSizeTwo();
}
*************************************************************
//put your header here
#include "StringList.h"
class Stack {
private:
StringList list;
public:
Stack();
void push(string);
string pop();
bool isEmpty();
};
***********************************************************
//put your header here
#include "StringList.h"
class Queue {
private:
StringList list;
public:
Queue();
void enqueue(string);
string dequeue();
bool isEmpty();
};
*************************************************************
riders.txt
10
Bridgette 2
Selena 5
Javier 3
Paula 4
Lorelei 1
Nigel 1
April 8
Will 10
Matthew 6
Porsche 2
*************************************************************
Expected output
Train #1
--------------
Bridgette 1
Bridgette 2
Selena 1
Selena 2
Selena 3
Selena 4
Selena 5
Javier 1
Javier 2
Javier 3
Train #2
--------------
Paula 1
Paula 2
Paula 3
Paula 4
Lorelei 1
Nigel 1
Train #3
--------------
April 1
April 2
April 3
April 4
April 5
April 6
April 7
April 8
Train #4
--------------
Will 1
Will 2
Will 3
Will 4
Will 5
Will 6
Will 7
Will 8
Will 9
Will 10
Train #5
--------------
Matthew 1
Matthew 2
Matthew 3
Matthew 4
Matthew 5
Matthew 6
Porsche 1
Porsche 2
Total Riders: 42
Total Trains: 5
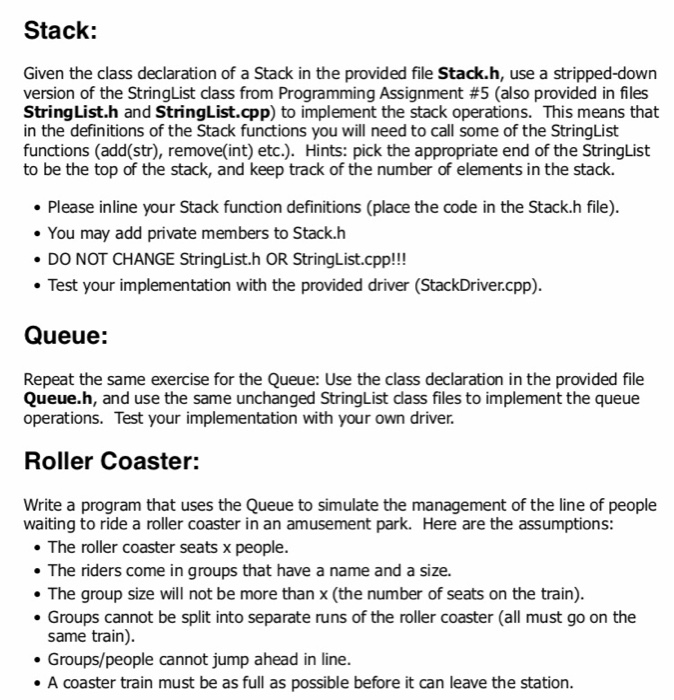
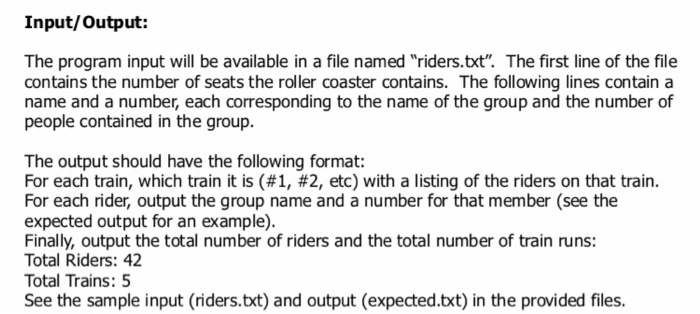
Step by Step Solution
There are 3 Steps involved in it
Step: 1
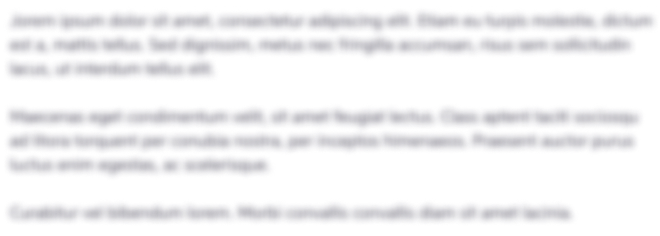
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started