Need help with this java code
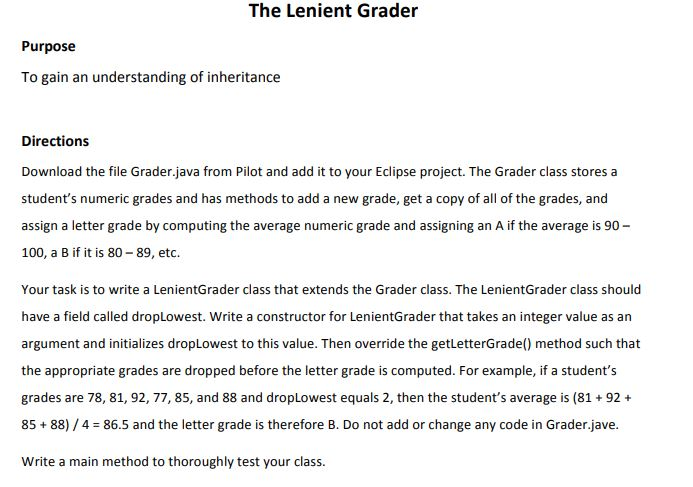
And here is the grader class
import java.util.ArrayList;
public class Grader {
private ArrayList grades; public Grader() { grades = new ArrayList(); } public void addGrade(double grade) { grades.add(grade); }
public ArrayList getGrades() { ArrayList copyOfGrades = new ArrayList(); for (Double grade: grades) { copyOfGrades.add(grade); } return copyOfGrades; } public char getLetterGrade() { double sum = 0.0; for (Double grade : grades) { sum += grade; } double average = sum / grades.size(); if (average >= 90) { return 'A'; } else if (average >= 80) { return 'B'; } else if (average >= 70) { return 'C'; } else if (average >= 60) { return 'D'; } else { return 'F'; } } }
The Lenient Grader Purpose To gain an understanding of inheritance Directions Download the file Grader.java from Pilot and add it to your Eclipse project. The Grader class stores a student's numeric grades and has methods to add a new grade, get a copy of all of the grades, and assign a letter grade by computing the average numeric grade and assigning an A if the average is 90- 100, a B if it is 80- 89, etc. Your task is to write a LenientGrader class that extends the Grader class. The LenientGrader class should have a field called dropLowest. Write a constructor for LenientGrader that takes an integer value as an argument and initializes dropLowest to this value. Then override the getLetterGrade() method such that the appropriate grades are dropped before the letter grade is computed. For example, if a student's grades are 78, 81, 92, 77, 85, and 88 and dropLowest equals 2, then the student's average is (81+92+ 85 +88)/4 86.5 and the letter grade is therefore B. Do not add or change any code in Grader.jave. Write a main method to thoroughly test your class