Question
Need help with this java coding, thank you! ________________________________________________________________________ Class.java coding: public class Circle { // Student Starter code private double radius; // circle radius
Need help with this java coding, thank you!
________________________________________________________________________
Class.java coding:
public class Circle { // Student Starter code private double radius; // circle radius /** * Constructor - Create a new circle * @param inRadius radius of the circle */ public Circle(double inRadius ) { // implement body here } /** * Return the radius of the circle * @return radius of the circle */ public double getRadius() { return radius; } /** * set the radius * @param newRadius new radius of the circle */ public void setRadius(double newRadius) { // implement body here } /** * Compute and return the area of the circle * @return the area of the circle */ public double area() { return Math.PI * radius * radius; } /** * Stretches circle size by multiplying * the radius by the factor provided. * @param factor stretch factor */ public void stretchBy(double factor ) { // implement body here } /** * Return a string representation of a circle. * @return a string representing this circle */ public String toString() { // implement body here } }
________________________________________________________________________
TestCircle.java coding here:
public class TestCircle { public static void main(String [] args) { // read a radius of the circle from command line double radius = Double.parseDouble(args[0]); // Instantiate a Circle object Circle aCircle = new Circle(radius); // Print current status of the circle System.out.println(aCircle); } }
Exercise 1 Exception Handling Concept (5 points) Part 1: Throw Exceptions (Checked Exceptions) (Circle.java) Open the file Circle.java in today's downloads. Add the body to the Constructor that is already present in the Circle class. Check to see if the radius parameter entered is greater than 0.0 or not (i.e. is the circle valid?) If the value of the radius is less than zero, throw an Exception. Otherwise, proceed as normal by initializing the instance variable radius to the parameter entered. A try/catch block is not needed here, but the method signature will require modification Similarly, in the setRadius () method, add the implementation to the body such that if the newRadius parameter is less than or equal to 0.0, throw an Exception Otherwise, set the radius to this newRadius value. Again, in the stretchBy) method, add the implementation to the body such that if the factor parameter is less than or equal to 0.0, throw an Exception. Otherwise change the radius by multiplying it by the factor. Finally, write the toString method to return the string "circle: "with the value of the radius appended to the end. Make sure the class compiles. A test class will be necessary to run the progranm Part 2: User-Defined Exception Class (ShapeException) Create a file called ShapeException.java with the class ShapeException which extends the Exception class This ShapeException class has only ONE method, the constructor that takes ONE parameter, a String message stating the nature of the exception. Inside the constructor, call the parent's one-parameter constructor with the message as a parameter. Note: this is a very short class! Now change the Circle class by replacing Exception with ShapeException. When a ShapeException is thrown (throw new ShapeException ("..."), include Exercise 1 Exception Handling Concept (5 points) Part 1: Throw Exceptions (Checked Exceptions) (Circle.java) Open the file Circle.java in today's downloads. Add the body to the Constructor that is already present in the Circle class. Check to see if the radius parameter entered is greater than 0.0 or not (i.e. is the circle valid?) If the value of the radius is less than zero, throw an Exception. Otherwise, proceed as normal by initializing the instance variable radius to the parameter entered. A try/catch block is not needed here, but the method signature will require modification Similarly, in the setRadius () method, add the implementation to the body such that if the newRadius parameter is less than or equal to 0.0, throw an Exception Otherwise, set the radius to this newRadius value. Again, in the stretchBy) method, add the implementation to the body such that if the factor parameter is less than or equal to 0.0, throw an Exception. Otherwise change the radius by multiplying it by the factor. Finally, write the toString method to return the string "circle: "with the value of the radius appended to the end. Make sure the class compiles. A test class will be necessary to run the progranm Part 2: User-Defined Exception Class (ShapeException) Create a file called ShapeException.java with the class ShapeException which extends the Exception class This ShapeException class has only ONE method, the constructor that takes ONE parameter, a String message stating the nature of the exception. Inside the constructor, call the parent's one-parameter constructor with the message as a parameter. Note: this is a very short class! Now change the Circle class by replacing Exception with ShapeException. When a ShapeException is thrown (throw new ShapeException ("..."), includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
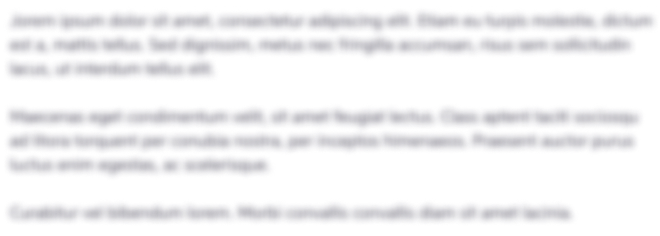
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started