Question
need help with this java program - I wrote this program that is a card game that the end goal is to match 4 cards
need help with this java program -
I wrote this program that is a card game that the end goal is to match 4 cards of the same value(1,2,3,4,5,6 etc..) but with all 4 different suits..for example
*queen of hearts, queen of spades, queen of diamonds, queen of clubs.* -> if a player gets this output then the game is over and that player has won.
I need help to make my computer Player class more functional, for example making it able to win the game. (ComputerPlayer.java)
I need help to make my computer Player class more functional, for example making it able to win the game. (ComputerPlayer.java) This is what i need help doing^^^^
right now it can never win the game. this is the directions for what the computer program is supposed to do:
1. The computer can either draw a card from the draw pile or pick up the card that the human player put onto the discard pile. Then the computer player must place one of its cards onto the discard pile. If the computer player now has four cards with the same value, it wins. Otherwise, it is the human players turn; however, this time the human player can either take a card from the draw pile or pick up the card that the computer player put onto the discard
here is my code so far:
****CardGame.java********
package cardgame;
import java.util.Collections; import java.util.Queue;
import java.util.LinkedList; import java.util.List; import java.util.Random; import java.util.Scanner; import java.util.Stack;
/** * * @author */ public class CardGame {
Queue deck; ComputerPlayer computer; Player human; Stack discardPile;
public CardGame() { char suit[] = {'H', 'S', 'D', 'C'}; deck = new LinkedList(); for (int i = 0; i < 4; i++) { for (int j = 1; j <= 13; j++) { deck.add(new Card(j, suit[i])); } }
discardPile = new Stack();
human = new Player(); computer = new ComputerPlayer(); }
private void shuffle() {
Collections.shuffle((List) deck);
this.deck = (LinkedList)deck;
}
public void start() { shuffle(); for (int i = 0; i < 4; i++) { human.addCard(deck.remove(0)); computer.addCard(deck.remove(0)); } int turn = 0; int choice; Card current; Card top; Scanner in = new Scanner(System.in); while (!human.hasWon() && !computer.hasWon()) { if (deck.isEmpty()) { deck.addAll(discardPile); discardPile.clear(); shuffle(); }
if (turn == 0) { System.out.println("Your cards are: "); human.displayCards(); System.out.println(""); if (discardPile.isEmpty()) { choice = 2; System.out.println("The discard pile is empty, draw a card."); System.out.println("");
} else { top = discardPile.peek(); System.out.println("The top of the discard pile is the " + top); System.out.println("Do you want to pick up the " + top.toString() + "(1) or draw a card (2)"); choice = in.nextInt(); }
if (choice == 1) { current = discardPile.pop(); System.out.println("You picked up " + current);
} else { current = deck.remove(0); System.out.println("You drew " + current); } human.addCard(current); System.out.println("Now your cards are "); human.displayCards(); System.out.println(""); System.out.println("Which one do you want to discard ?"); choice = in.nextInt(); discardPile.push(human.discard(choice - 1)); } else { if (!discardPile.isEmpty() && discardPile.peek().getValue() == computer.getMaxValue()) { top = discardPile.pop(); System.out.println("I will pick up the " + top); computer.addCard(top);
} else { System.out.println("I will draw a new card."); current = deck.remove(0); computer.addCard(current); } current = computer.discard(); discardPile.push(current); System.out.println("I will discard the " + current); }
turn = 1 - turn; }
}
public void finish() { System.out.println("--------------------------"); System.out.println("My cards are "); computer.displayCards(); System.out.println("--------------------------"); System.out.println("Your cards are "); human.displayCards(); System.out.println("--------------------------"); if (human.hasWon()) { System.out.println("You have won !"); } else { System.out.println("the computer wins !"); } System.out.println("--------------------------"); }
public static void main(String[] args) { CardGame game = new CardGame(); game.start(); game.finish(); } }
***end of cardgame.java******
here is player class
******player.java***************
package cardgame;
import java.util.ArrayList; import java.util.Collections;
public class Player {
String name; ArrayList cards;
public Player(String playerName) { name = playerName; }
public Player() { cards = new ArrayList(); }
public void addCard(Card card) { cards.add(card); Collections.sort(cards); }
public Card discard(int index) { return cards.remove(index); }
public Card getCard(int index) { return cards.get(index); }
public boolean hasWon() { int value = cards.get(0).getValue(); for (int i = 1; i < cards.size(); i++) { if (cards.get(i).getValue() != value) { return false; } else { } }
return true; }
public void displayCards() { for (int i = 0; i < cards.size(); i++) { System.out.println(i + 1 + ". " + cards.get(i)); } }
}
*****end of player.java*****
here is computerplayer class
*****ComputerPlayer.java************
package cardgame;
public class ComputerPlayer extends Player {
private int decideDiscard() { int pValue = cards.get(0).getValue(); int index = 0; int count = 1; int min = 1; for (int i = 1; i < cards.size(); i++) { if (cards.get(i).getValue() != pValue) { if (count < min) { min = count; index = i - 1; pValue = cards.get(i).getValue();
} count = 1;
} else { count++; } } return index; }
public Card discard() { return cards.remove(decideDiscard()); }
public int getMaxValue() { int pValue = cards.get(0).getValue(); int count = 1; int max = 1;
for (int i = 1; i < cards.size(); i++) { if (cards.get(i).getValue() != pValue) { if (count > max) { max = count; pValue = cards.get(i).getValue(); } count = 1; } else { count++; } } return pValue; } }
***end of computerplayer class********
here is card class
*****Card.java*********
package cardgame;
public class Card implements Comparable {
int value; char suit; private static final String[] VALUENAMES = {"", "Ace", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"};
public Card(int cardValue, char cardSuit) { value = cardValue; suit = cardSuit; }
int getValue() { return value;
}
char getSuit() { return suit; }
@Override public int compareTo(Card c) { if (value < c.value) { return -1; } else if (value > c.value) { return 1; } else { return 0; } }
@Override public String toString() { String suits = VALUENAMES[value] + " of "; switch (suit) { case 'H': suits += "Hearts"; break; case 'S': suits += "Spades"; break; case 'D': suits += "Diamonds"; break; default: suits += "Clubs"; break; }
return suits; }
}
****end of card.java**************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
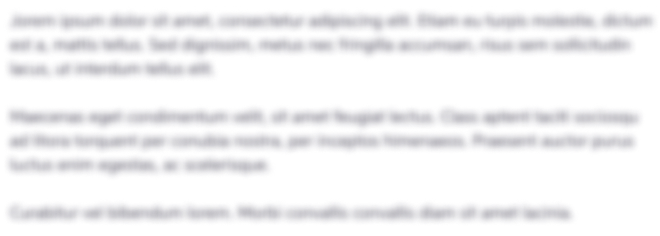
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started