Question
Need help with this my current code is: import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; class Athlete { private String type; private double swimSpeed;
Need help with this my current code is:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
class Athlete {
private String type;
private double swimSpeed;
private double bikeSpeed;
private double runSpeed;
private String name;
public Athlete(String type, double swimSpeed, double bikeSpeed, double runSpeed, String name) {
this.type = type;
this.swimSpeed = swimSpeed;
this.bikeSpeed = bikeSpeed;
this.runSpeed = runSpeed;
this.name = name;
}
public String getType() {
return type;
}
public double getSwimSpeed() {
return swimSpeed;
}
public double getBikeSpeed() {
return bikeSpeed;
}
public double getRunSpeed() {
return runSpeed;
}
public String getName() {
return name;
}
public void printAthlete() {
if (type.equals("t")) {
System.out.println(name + " is a Triathlete and is an athlete that bikes at " + bikeSpeed + " mph");
System.out.println("During the Ironman triathlon, I swim 2.4 miles, bike 112 miles, then run 26.2 miles.");
} else if (type.equals("d")) {
System.out.println(name + " is a Duathlete and is an athlete that bikes at " + bikeSpeed + " mph");
System.out.println("I run, bike, then sometimes run again. In a long distance duathlon, I run 6.2 miles, bike 93 miles, then run 18.6 miles");
} else if (type.equals("m")) {
System.out.println(name + " is a Marathoner and is an athlete that runs at " + runSpeed + " mph");
System.out.println("During a full marathon I run 26.2 miles!");
}
}
}
class main1 {
public static void main(String[] args) throws FileNotFoundException {
ArrayList
Scanner sc = new Scanner(new File("athletes.txt"));
int count = sc.nextInt();
sc.nextLine();
for (int i = 0; i
String[] parts = sc.nextLine().split(" ");
String type = parts[0];
double swimSpeed = Double.parseDouble(parts[2]);
double bikeSpeed = Double.parseDouble(parts[3]);
double runSpeed = Double.parseDouble(parts[1]);
String name = parts[4];
Athlete athlete = new Athlete(type, swimSpeed, bikeSpeed, runSpeed, name);
athletes.add(athlete);
}
sc.close();
System.out.println("ATHLETES THAT BIKE!");
System.out.println("----------------------");
for (Athlete athlete : athletes) {
if (athlete.getType().equals("t") || athlete.getType().equals("d") && athlete.getBikeSpeed() > 0) {
athlete.printAthlete();
}
}
System.out.println(" ATHLETES THAT DO NOT SWIM!");
System.out.println("--------------------------");
for (Athlete athlete : athletes) {
if (athlete.getSwimSpeed() == 0) {
athlete.printAthlete();
}
}
System.out.println(" SLOWEST RUNNER!");
System.out.println("--------------------------");
Athlete slowestRunner = null;
for (Athlete athlete : athletes) {
if (athlete.getType().equals("m") && (slowestRunner == null || athlete.getRunSpeed()
slowestRunner = athlete;
}
}
System.out.println("Slowest runner is " + slowestRunner.getName() + " who is a Marathoner with a running speed of " + slowestRunner.getRunSpeed() + " mph");
}
}
The file contains:
7 t 9.52 2.88 25.84 Dave Scott d 8.32 0 23.47 Kenny Souza a 9.01 2.04 0 Hannah Kitchen m 6.67 0 0 Will Ferrell t 9.57 3.00 26.35 Mark Allen a 8.90 2.54 0 Thomas Roos m 7.01 0 0 George Bush
My code produces the desired output but is supposed to be formatted differently than I have it
CS 1450 Data Structures and Algorithms Assignment \#3 Purpose: Learn to write abstract classes and interfaces. Practice using arrays and ArrayLists. Effort: Individual Points: 4.5 Deliverables: Upload a .zip file with the ONE source code file (one .java file) along with your design notebook in a document file to Canvas by due date (no late assignments accepted). Assignment Description The table below shows different types of athletes and their disciplines during competition - running, swimming, and biking. Each type of athlete has a fixed set of disciplines as is listed in the table with a Y. Specifications 1. Create a Java class called LastNameFirstNameAssignment3 2. Follow "CS1450 Programming Assignments Policy" 3. Write a test program (i.e. main) that performs the following steps: a. Creates a polymorphic array to store the athlete objects. b. For each line in the file athletes.txt: i. Read the information for the athlete. ii. Create an athlete object. iii. Place the athlete object into the array. c. AFTER all athlete objects are in the array, perform these 3 tasks on the array of athletes: i. Find which athletes are bikers ii. Find which athletes do not swim iii. Find the athlete with the slowest running speed For tasks i and ii implement the following methods where each method takes the array of all athletes and returns an ArrayList of specific athletes. public static ArrayList findBikers (Athlete[ ] athletes) public static ArrayList findDoNotSwim (Athlete [] athletes) For task iii implement a method that returns the array index of the slowest runner: public static int findSlowestRunner (Athlete[] athletes) NOTE: be sure to place these 3 methods inside the Assignment3 class, right after main. d. Displays on the console the following for each method in step 3c (see output below) Athlete's name Type of athlete (triathlete, duathlete, etc.) Athlete's pace (ONLY for findBikers and findSlowestRunner methods) Athlete's discipline details (use abstract disciplines() method) 4. Test file information: a. Run your code on the provided file athletes.txt b. This file is an example so DO NOT assume that your code should work for only 7 athletes in the order specified in the file. 7 t 9.522.8825.84 Dave Scott d 8.32023.47 Kenny Souza a 9.012.040 Hannah Kitchen m 6.6700 Will Ferrell t 9.57 3.00 26.35 Mark Allen a 8.90 2.540 Thomas Roos m 7.0100 George Bush c. 1st line is an integer value representing the number of athletes in the file d. Remaining lines contain details for each athlete. The format is as follows: e. Note: If run speed, swim speed, or bike speed is zero then the athlete does not participate in that discipline (i.e., it's not part of their competition). For example: Aquathletes do not bike; so, their bike speed is set to 0 . Interfaces and Classes Runner, Swimmer, and Biker Interfaces - Description - Each interface represents a specific discipline such as running, swimming, or biking. - Public Methods - Runner interface has a method named run() - Returns a double value representing run speed in mph - Swimmer interface has a method named swim() - Returns a double value representing swim speed in mph - Biker interface has a method named bike() - Returns a double value representing bike speed in mph Athlete Class - Description - ABSTRACT class that represents a generic athlete. - Superclass in the hierarchy. - Private Data Fields - name - String for athlete's name - type - String for type of athlete (i.e. Triathlete, Duathlete, etc.) - Public Methods - Constructor - none - Unlike assignment \#2, setters are provided in the Athlete class to set the instance variables so no constructor is needed in Athlete. - Getter for each data field - Setter for each data field - ABSTRACT method disciplines() - Returns string describing disciplines associated with a specific type of athlete. Triathlete, Duathlete, Aquathlete, and Marathoner Subclasses - Description - Each represents a specific athlete - these are the subclasses in the hierarchy. - A triathlete will swim, bike and run during a triathlon - A duathlete will run, bike, then possibly run again during a duathlon - An aquathlete will run, swim, then possibly run again during an aquathlon - A marathoner will run during a marathon. Each must extend Athlete. Each must implement only the interfaces for the athlete's specific disciplines. - Private Data Fields Instance variables that represent the speed associated with each discipline the specific athlete (subclass) takes part in. - For example, a triathlete needs a swim speed, run speed, and bike speed. - Public Methods - Constructors - Takes the name of the athlete and the speed values for ONLY the disciplines this type of athlete takes part in. For example: Duathlete constructor takes: name, runspeed and bikespeed. public Duathlete (String name, double runspeed, double bikespeed) - Subclass must implement ONLY the interfaces for the disciplines that athlete performs. - Example: Duathlete class only implements Runner and Biker. - This means Duathlete must override the run() and bike() interface methods - Each subclass must override the disciplines() method in the abstract Athlete class. - Use the following strings for the disciplines for each type of athlete Tips Must Do - Use an array to store the athletes you create from the information in the file. - Use an ArrayList to return athletes from the methods. See step 3c. - Do not assume the file provided is what the grader will use. It is important to learn to not make assumptions when writing code. For example, do not assume there are 7 athletes and do not assume the athletes will be in the order they are presented in the file. Casting - Read Liang, section 11.9 Casting Objects and the instanceof Operator and look at listing 11.7, it contains both instanceof and casting. This should help you understand what is required to determine which interface you are working with. - After calling findSlowestRunner, you need to print the slowest runner's run speed. - When obtaining the slowest athlete's run speed, casting to Runner is required. - This is necessary because the run method is in Runner interface and not in Athlete class. - That is, when calling the athlete's run method, you'll need to cast otherwise you'll get the error: "the method run() is undefined for type Athlete" Code Incrementally - Get the code working in bits. - Make sure you can read all the data from the file correctly, then create the athlete objects, etc. Output Your output will look like the following when running against the test file athletes.txt ATHLETES THAT BIKE! Dave Scott is a Triathlete and is an athlete that bikes at 25.84mph During the Ironman triathlon, I Swim 2.4 miles, bike 112 miles, then run 26.2 miles. Kenny Souza is a Duathlete and is an athlete that bikes at 23.47mph I run, bike, then sometimes run again. In a long distance duathlon, I run 6.2 miles, bike 93 miles, then run 18.6 miles Mark Allen is a Triathlete and is an athlete that bikes at 26.35mph During the Ironman triathlon, I Swim 2.4 miles, bike 112 miles, then run 26.2 miles. ATHLETES THAT DO NOT SWIM! Kenny Souza is a Duathlete and does not swim I run, bike, then sometimes run again. In a long distance duathlon, I run 6.2 miles, bike 93 miles, then run 18.6 miles Will Ferrell is a Marathoner and does not swim During a full marathon I run 26.2 miles! George Bush is a Marathoner and does not swim During a full marathon I run 26.2 miles! SLOWEST RUNNER! Slowest runner is Will Ferrell who is a Marathoner with a running speed of 6.67mphStep by Step Solution
There are 3 Steps involved in it
Step: 1
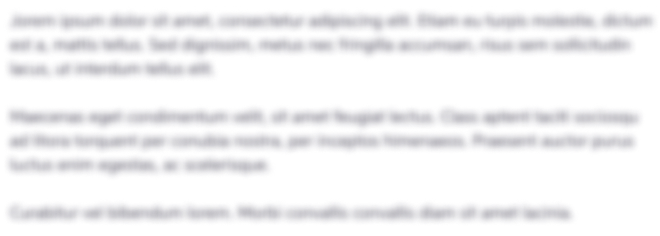
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started