Question
Need help with void exp10ToExp2.(In C) Edit the file exp10to2.c and implement exp10ToExp2. Your code should take the struct Number pointed by parameter pn and
Need help with void exp10ToExp2.(In C)
Edit the file exp10to2.c and implement exp10ToExp2.
Your code should take the struct Number pointed by parameter "pn" and preserve the value .
V=mantissa*2^exp2*10^exp10
he restrictions are as follows:
- mantissa should end up as a value that has bit 63 set. mantissa is a 64-bit unsigned integer.
- exp10 should be 0 when your algorithm is done.
- exp2 can be any integer (signed)
- the represented value v should be preserved as much as possible
To run the program with your specific test input, do the following in CodeBlocks:
- put a break point at the end of exp10ToExp2, you can click slightly to the right of the line number to toggle breakpoint on a line.
- In the "Project" menu, click "set programs' arguments"
- In Program Arguments, enter the following without quotes:"-n 1.23e20" (obviously change the floating point number to the one that you should use), click OK
- when the program stops at the break point, you can examine the expressing *pn
- In the Debugger pane, you can type the following command without the quotes "print *pn", copy-and-paste the output to the HTML editor area
Here is a trace of an example (you have to figure out the loop structure):
./main -n 1.23456789e30 mantissa = 123456789, exp10 = 22, exp2 = 0 mantissa = 123456789, exp10 = 22, exp2 = 0 mantissa = 12345678900000000000, exp10 = 11, exp2 = 0 mantissa = 1543209862500000000, exp10 = 11, exp2 = 3 mantissa = 15432098625000000000, exp10 = 10, exp2 = 3 mantissa = 964506164062500000, exp10 = 10, exp2 = 7 mantissa = 9645061640625000000, exp10 = 9, exp2 = 7 mantissa = 1205632705078125000, exp10 = 9, exp2 = 10 mantissa = 12056327050781250000, exp10 = 8, exp2 = 10 mantissa = 1507040881347656250, exp10 = 8, exp2 = 13 mantissa = 15070408813476562500, exp10 = 7, exp2 = 13 mantissa = 941900550842285157, exp10 = 7, exp2 = 17 mantissa = 9419005508422851570, exp10 = 6, exp2 = 17 mantissa = 1177375688552856447, exp10 = 6, exp2 = 20 mantissa = 11773756885528564470, exp10 = 5, exp2 = 20 mantissa = 1471719610691070559, exp10 = 5, exp2 = 23 mantissa = 14717196106910705590, exp10 = 4, exp2 = 23 mantissa = 1839649513363838199, exp10 = 4, exp2 = 26 mantissa = 18396495133638381990, exp10 = 3, exp2 = 26 mantissa = 1149780945852398875, exp10 = 3, exp2 = 30 mantissa = 11497809458523988750, exp10 = 2, exp2 = 30 mantissa = 1437226182315498594, exp10 = 2, exp2 = 33 mantissa = 14372261823154985940, exp10 = 1, exp2 = 33 mantissa = 1796532727894373243, exp10 = 1, exp2 = 36 mantissa = 17965327278943732430, exp10 = 0, exp2 = 36 mantissa = 17965327278943732430, exp10 = 0, exp2 = 36 mantissa = 17965327278943732430, exp10 = 0, exp2 = 36
Here is the main:
#include
struct Number { int sign; uint64_t mantissa; int16_t exp10; int16_t exp2; };
struct ParseState { const char *ptr; struct Number *pn; };
int parse(const char *str, struct Number *pn);
int parseSign(struct ParseState *pps) { int retValue = 0; switch (*pps->ptr) { case '+': ++pps->ptr; // skip, default sign is fine break; case '-': ++pps->ptr; // advance retValue = 1; break; default: break; } return retValue; }
int isDigit(const char p) { return (p >= '0') && (p <= '9'); }
uint64_t parseUnsignedInt(uint64_t n, struct ParseState *pps) { while (isDigit(*pps->ptr)) { n = n * 10 + (*pps->ptr - '0'); ++pps->ptr; } return n; }
void parseMantissa(struct ParseState *pps) { // after sign, parse the main part pps->pn->mantissa = 0; pps->pn->exp10 = 0; { const char *oldPtr = pps->ptr; pps->pn->mantissa = parseUnsignedInt(0,pps); if (oldPtr != pps->ptr) // pointer advanced { if (*pps->ptr == '.') { ++pps->ptr; const char *oldPtr = pps->ptr; pps->pn->mantissa = parseUnsignedInt(pps->pn->mantissa, pps); pps->pn->exp10 -= (pps->ptr - oldPtr); } } } }
int parse(const char *str, struct Number *pn) { struct ParseState ps; int error = 0; ps.ptr = str; ps.pn = pn; pn->exp10 = 0; pn->exp2=0; pn->sign = parseSign(&ps); { const char *oldPtr = ps.ptr; parseMantissa(&ps); if (oldPtr != ps.ptr) { if (*ps.ptr == 'e') { int expSign; uint64_t exp = 0; ++ps.ptr; expSign = parseSign(&ps); exp = parseUnsignedInt(exp, &ps); pn->exp10 += (expSign ? -exp : exp); } } else { error = 1; } } return error; }
void exp10ToExp2(struct Number *pn);
void exp10ToExp2(struct Number *pn) { // in this function, convert the number pointed to b // pn from a base-10 mantissa and exponent to a base-2 // mantissa and exponent // divide into two main cases: when exp10 > 0, and when // exp10 < 0 while (pn->exp10 < 0) { while (pn->mantissa <= ((UINT64_MAX - 5) / 2)) { pn->mantissa *= 2; pn->exp2--; } pn->mantissa = ((pn->mantissa + 5) / 10); pn->exp10++; } }
int main(int argc, const char **argv) { const char *numberStr = NULL; struct Number n; int error = 0; --argc; ++argv; // skip name of executable itself while (!error && argc) { if (strcmp(*argv,"-n") == 0) { --argc; ++argv; if (argc) { numberStr = *argv; --argc; ++argv; } else { fprintf(stderr,"-n should be followed by a number "); error = 1; } } } if (!error && numberStr) { // no errors encountered parse(numberStr, &n); exp10ToExp2(&n); } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
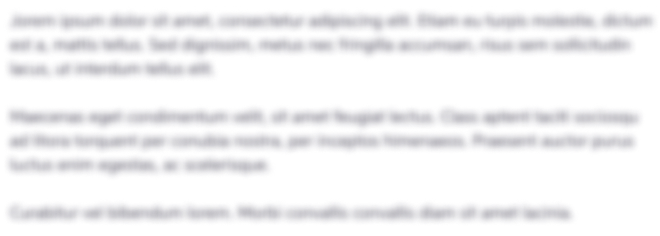
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started