Question
Need help writing a Python program. # NOTE: # You must use small single letters for your variable names, for eg. a, b, c #
Need help writing a Python program.
# NOTE: # You must use small single letters for your variable names, for eg. a, b, c # You may use parenthesis to group your expressions such as 'a and (b or c)'
# Implement the following four functions: # truth_table, count_satisfying, is_tautology and are_equivalent
# Submission: # Submit this file using the checkin system on the course web page.
######## Do not modify the following block of code ######## # ********************** BEGIN *******************************
from functools import partial import re
class Infix(object): def __init__(self, func): self.func = func def __or__(self, other): return self.func(other) def __ror__(self, other): return Infix(partial(self.func, other)) def __call__(self, v1, v2): return self.func(v1, v2)
@Infix def implies(p, q) : return not p or q
@Infix def iff(p, q) : return (p |implies| q) and (q |implies| p)
# You must use this function to extract variables # This function takes an expression as input and returns a sorted list of variables # Do NOT modify this function def extract_variables(expression): sorted_variable_set = sorted(set(re.findall(r'\b[a-z]\b', expression))) return sorted_variable_set
# *********************** END ***************************
############## IMPLEMENT THE FOLLOWING FUNCTIONS ############## ############## Do not modify function definitions ##############
# This function calculates a truth table for a given expression # input: expression # output: truth table as a list of lists # You must use extract_variables function to generate the list of variables from expression # return a list of lists for this function def truth_table(expression): #calling extract_variables to return a sorted list of all variables in expression var_list = extract_variables(expression) pass
# count the number of satisfying values # input: expression # output: number of satisfying values in the expression def count_satisfying(expression): pass
# if the expression is a tautology return True, # False otherwise # input: expression # output: bool def is_tautology(expression): pass
# if expr1 is equivalent to expr2 return True, # False otherwise # input: expression 1 and expression 2 # output: bool def are_equivalent(expr1, expr2): pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
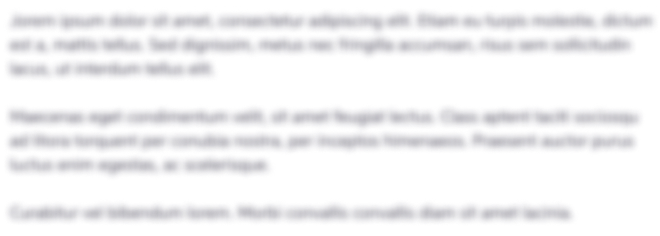
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started