Question
Need help writing the source code for both tasks. Task: Create a simple bank account simulation: Create a new project named Lab9-1. Enter the following
Need help writing the source code for both tasks.
Task: Create a simple bank account simulation:
Create a new project named "Lab9-1". Enter the following skeleton program. The main() function has been implemented for you. There are four functions you will need to implement to complete the lab.
#include
/* Header */
#define MAX_ACCTS 10
int accounts[MAX_ACCTS] =
{20181, 20467, 20813, 21354,21358, 21359, 22027, 22041};
double balances[MAX_ACCTS] =
{0, 145.72, 10133.00, -17.44, 612.98, 1415.30, 313.21, 3131.32};
int findAccount(int accountID) {
int index = -1;
/* Use a linear search to find the accountID in the accounts array. */
/* If found, return the index. */
index = 1; /* This line is just for testing - remove it */
/* If not found, return -1 */
return(index);
}
void printAccountBal(int accountIndex) {
/* Write this function. Use the account index * to find and print the account balance.
* Use good human factor design in your output. * Use correct format for currency. */
}
void deposit(int accountIndex) {
/* Write this function. Use the account index * to access the account balance.
* Prompt for a deposit amount. The deposit amount * should be greater than 0.
* Update the balance in the array. */
}
void withdraw(int accountIndex) {
/* Write this function. Use the account index * to access the account balance.
* Prompt for a withdrawal amount. * The withdrawal amount should be greater than 0.
* Make sure the withdrawal amount is not more than the balance.
* If the amount exceeds the balance,
* provide an error message and ask for a new withdrawal amount.
* Update the balance in the array. */
}
int main(void) {
int choice;
int accountID, accountIndex;
int accountIDgood;
printf("Bank account simulator ");
do {
accountIDgood = 0; /* False */
do {
printf(" Enter account ID: ");
scanf("%d", &accountID);
if (accountID < 20000 || accountID > 29999) {
printf("Account ID is not correct format. ");
} else {
accountIndex = findAccount(accountID);
if (accountIndex == -1) {
printf("Account ID %d is not valid. ",
accountID);
} else {
accountIDgood = 1; /* True */
}
}
} while (!accountIDgood);
printAccountBal(accountIndex);
do {
printf("Choose from the following menu: ");
printf("\t0 - Exit the program ");
printf("\t1 - Change accounts ");
printf("\t2 - Make deposit ");
printf("\t3 - Make withdrawal ");
printf("Enter your choice: ");
scanf("%d", &choice);
printf(" ");
switch (choice) {
case 2:
deposit(accountIndex);
printAccountBal(accountIndex);
break;
case 3:
withdraw(accountIndex);
printAccountBal(accountIndex);
break;
default:
if (choice != 0 && choice != 1) {
printf("Your choice (%d) is not valid. ", choice);
}
break;
}
} while (choice != 0 && choice != 1);
} while (choice != 0);
return(0);
}
Build and test the program. The program is designed so that account numbers are five-digit integers starting with "2." Fix any compile-time syntax errors, and test that the program menu logic is working properly.
Create the code for the functions findAccount(), printAccountBalance(), deposit(), and withdraw(). Build and test the program. Test the program using a valid account number (one which appears in the array) as well as an invalid account number. Be sure to use invalid data in each response, as well as valid data, to verify that your program handles the input validation properly.
Attach all of your source code and program outputs to this worksheet.
Deliverables: Please submit all of your source codes and program outputsto Canvas.
Lab 9 (CS& 131 Unit 9 Lab 2) Strings
Task: Strings
Learning Objectives and Outcomes
- Develop a functional C program that implements a design.
- Create a C program that declares and uses strings.
Required Setup and Tools
- Standard computer lab with Microsoft Visual Studio 2017 and Microsoft Visio installed
Recommended Procedures
Task: Create a program to read and reverse a word.
Create a new project named "Lab9-2". Create a main() function with the usual header and other supporting code. Add a declaration of a string variable named "word" that can hold at least 29 characters (plus the terminator). Add code to print a prompt and add a call to scanf() that reads a string into the variable word. Add another call to printf() that echoes the characters entered back to the output. Build and test the program.
Add a declaration of an int variable named "length." Then add a while loop that begins at the first character element of word and increments length while (word[length]!=0). Add a statement after the loop that prints the length found. Build and test your program, and verify that the proper length of the word is printed. Add a for loop that reverses the word. This can be accomplished as follows:
- Start a loop iterator at the value 0.
- Terminate the loop when the iterator is equal to (length+1)/2.
- Add a char variable named temp.
- In the loop, use the following statements to swap the characters in the string:
temp = word[i]; word[i] = word[length-i-1]; word[length-i-1] = temp;
- Be sure to print the reversed word after exiting the for loop!
Build and test your program using at least three test cases. One test case should use a single-letter word such as "a". A second test case should use a word with an even number of letters, such as "even". A third test case should use a word with an odd number of letters, such as "odd".
Attach all of your source code and program outputs to this worksheet
Deliverables: Please submit all of your source codes and program outputsto Canvas.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
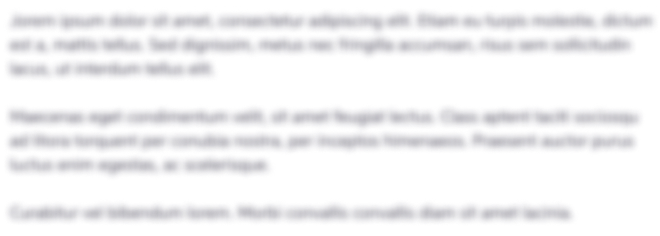
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started