Question
Need help! Your program should allow users to load video game data from a file, save video games to a file, add a video game,
Need help! Your program should allow users to load video game data from a file, save video games to a file, add a video game, remove a video game, display all video game in the library, and remove all video games. Files you will submit in your zip file This program contains multiple files as described below: Text.h header file for a Text class, which is your own version of the C++ String Class Text.cpp source file containing function definitions required for the Text class VideoGame.h header file for a VideoGame class, which has data and functions describing a single video game. VideoGame.cpp source file containing the function definitions required for the VideoGame class. VideoGameLibrary.h header file for a VideoGameLibrary class, which has data and functions describing a video game library (multiple games) VideoGameLibrary.cpp source file containing the function definitions required for the VideoGameLibrary class. Program1.cpp this is the driver for your program which uses the VideoGameLibrary class to create a VideoGameLibrary object. three_ games.txt text file given to you that contains data on three video games that you can use to test your program twenty_games.txt text file given to you that contains data on twenty video games that you can use to test your program runProgram.bat given to you and runs the makefile and then runs the code using a testing text file can only work on Windows operating system Makefile makefile given to you for compiling your program TEST_FILE.txt given to you this is used to test your program an is used by the runProgram batch file. specifications program1.cpp (driver) Your program should ask the user How many video games can your library hold? Once it reads in this number, it should dynamically allocate a VideoGameLibrary object, sending this number as an argument. Then, the program should display a menu of six choices: What would you like to do? 1. Load video games from file. 2. Save video games to a file. 3. Add a video game. 4. Remove a video game. 5. Display all video games. 6. Remove ALL video games from this library and end program. CHOOSE 1-6: If the user chooses to load a video game from a file, then ask the name of the file and call the VideoGameLibrarys loadVideo gamesFromFile function, sending the filename as a string. If the user chooses to save video games to a file, then ask the name of the file and call the VideoGameLibrarys saveToFile function, sending the filename as a string. If the user chooses to add a video game, call the VideoGameLibrarys addVideoGameToArray function. If the user chooses to remove a video game, call the VideoGameLibrarys removeVideoGameFromArray function. If the user chooses to display all video games, call the VideoGameLibrarys displayVideoGames function. If the user chooses to remove all video games from the library and end the program, then you should release (delete) the VideoGameLibrary object and end the program. VideoGameLibrary class Attributes videoGamesArray a pointer to an array of pointers. Each pointer in the array should be able to point to (hold the memory address of) an individual Video game object. [Hint: you will need TWO stars to define this attribute. ] maxGames this is the maximum number of video games the library can hold and is the size of the video gamesArray. numGames this is the current number of video games actually pointed to in the videoGamesArray.
Functions Function name: resizeVideoGameArray Parameters: none Returns: none (void) Purpose: This function is called by addVideoGameToArray when the array size is not big enough to hold a new video game that needs to be added. The function makes the array twice as big as it currently is and then moves all the video game pointers to this new array. Function name: VideoGameLibrary constructor Parameters: An integer containing the maximum size of the video game library Purpose: This function is automatically called when a VideoGameLibrary object is created and it creates a library of video games. Specifications: The function will dynamically allocate an array of pointers to VideoGame objects based on the maximum size and will also set the current number of video games to zero. Function name: ~VideoGameLibrary destructor Purpose: This function is automatically called when the VideoGame object is destroyed. This releases the dynamically created individual video games and then deletes the array. Specifications: Prints a message VideoGameLibrary destructor: Released memory for each game in the video game array and the array itself. Function name: addVideoGameToArray Parameters: none Returns: none (void) Purpose: This function should be called when you need to add a single video game to the video game library. Specifications: It should ask the user for the video game title (read in as c-string, then dynamically create a Text object), platform (read in as c-string, then dynamically create a Text object), video game year (integer), video game genre (read in as c-string, then dynamically create a Text object), video game age rating (read in as c-string, then dynamically create a Text object), and IGDB user rating (between 0 and 100). Then it should dynamically allocate a new VideoGame object, sending the video game data just acquired from the user as arguments to the VideoGame constructor. Then, this function should check to see if numGames is equal to maxGames. If it is equal, then call the resizeVideoGameArray function. Then, it should assign this new video game to the correct pointer in the videoGamesArray. Last, it should increment numGames. Function name: displayVideoGames Parameters: none Returns: none (void) Purpose: This function should be called when the user wants to have all the video games in the library printed to the screen. Specifications: This function loops through the video gamesArray and calls each Video games printVideoGameDetails function. Function name: displayVideoGameTitles Parameters: none Returns: none (void) Purpose: This function should be called when you want to print only the video game titles out of the video game library (when a user wants to remove a video game from library). Specifications: This function should loop through the video gamesArray, retrieve the Video games title by calling the Video games getVideoGameTitle function, and then printing out the title by calling the Texts displayText function. Function name: loadVideoGamesFromFile Parameters: A pointer to a character (c-string or string literal argument) containing the filename Returns: none (void) Purpose: This function should be called when the user wants to read video game data from a file and add the video games to the video game library. The file must have data in the following order: title, platform, year, genre, age rating, IGDB user rating. Specifications: This function will use a loop to read the contents of the file until reaching the end of file. For each video game, it will read the title in with a c-string and then dynamically allocate a Text to hold the title. Then it will read in the video game platforms with a c-string and then dynamically allocate a Text to hold the platform. Then it will read in the video game year. Then it will read in the video game genre with a c-string and then dynamically allocate a Text to hold the genre. Then it will read in the video game age rating with a c-string and then dynamically allocate a Text to hold the rating. Then it will read in the user rating (between 0 & 100). Then, it will dynamically create a new VideoGame object, sending the video game data just acquired from the user as arguments to the Video game constructor. Then, this function should check to see if numGames is equal to maxGames. If it is equal, then call the resizeVideoGameArray function. Then, it should assign this new video game to the correct pointer in the video gamesArray. Then, it should increment numGames. Then, it should print the title of the video game and say was added to the video game library! This should happen for each video game read from the file. At the end of the function, it should print out how many video games were read from the file & added to the library. [Note: The temporary c-string used to load in data should be able to hold a very large string 10000 characters] Function name: removeVideoGameFromArray Parameters: none Returns: none (void) Purpose: This function should be called when the user wants to remove one single video game from the video game library. The video game to be removed must is identified by the user and then removed. Specifications: This function should first make sure that the number of video games is at least 1. if not, it should print that there must always be one video game in the library and the function should end. Then, the function should call the displayVideoGameTitles function to print all the video game titles. Then, ask the user to choose a video game to remove between 1 & numGames. Once the user identifies the video game, your program should print that the video game title has been successfully deleted. Then, release the dynamically allocated space for this video game and move all array elements in video gameArray back 1 starting with this deleted video games element. Last, decrement numGames. Function name: saveToFile Parameters: A pointer to a character (c-string or string literal argument) containing the filename Returns: none (void) Purpose: This function should be called when the user wants to print all the video game data from the video game library to a file. The data is printed in the following order (one piece of data per line): title, platform, year, genre, age rating, IGDB user rating. Specifications: Open the file with the filename that was sent to this function. Then, use a loop to go through the video gameArray and call the Video games printVideoGameDetailsToFile function, sending the file stream object to be printed to. Then, close the file and print a confirmation that all video games have been printed to the filename. videogame classAttributes title a pointer to a Text object, which will hold the title of the video game platform a pointer to a Text object, which will hold the platforms that the video game is available on year an integer representing the year the video game was released genre a pointer to a Text object, which will hold the genre of the video game ageRating a pointer to a Text object, which will hold the age rating of the video game userRating a number between 0 and 100 representing the user rating from IGDB Functions Function name: VideoGame constructor Parameters: o A pointer to a Text variable, containing the title of the video game o A pointer to a Text variable, containing the platform(s) of the video game o An integer containing the year the video game was released o A pointer to a Text variable, containing the genre of the video game o A pointer to a Text variable, containing the age rating of the video game o A number containing the IGDB (user) rating of the video game (out of 100) Purpose: This function should be called when all video game information is known and it will create a new video game with this information. Specifications: initialize all attributes to the arguments sent to this function. Function name: ~VideoGame destructor Purpose: This function is automatically called when a Video game object is destroyed. This function releases the dynamically allocated text arrays in the Video game. Specifications: Release the dynamically allocated space for the video gameTitle, platform, genre, and age rating. Prints a message: VideoGame destructor: Released memory for title, platform, genre, & age rating. Function name: printVideoGameDetails Parameters: none Returns: none (void) Purpose: This function should be called when the user wants to print ALL the video game information to the screen. Specifications: Print the title, year, genre, rating & number of stars. Remember that in order to print the Text objects, you must call their displayText function. Function name: printVideoGameDetailsToFile Parameters: a file stream object (sent by reference) Returns: none (void) Purpose: This function should be called when the user wants to print ALL the video game information to the file. Specifications: Print the title, year, genre, rating & user ratinmg to the file stream object that was sent to this function. In order to print the Text objects to the file, you must first retrieve the c-string attribute (calling the getText function) from this Text, and then you can print it to the file. Function name: getVideoGameTitle (accessor function) Parameters: none Returns: a pointer to the Text object containing the video game title Text class Attributes textArray a pointer to a constant character array textLength an integer representing the number of characters stored in the textArray Functions Function Name: Text (constructor) Parameters: Send a pointer to a constant character array or a string literal to this function Purpose: called automatically when Text object is created, dynamically allocates a character array which contains the character array passed to the function. Specifications: dynamically allocate a new character string the size of the string passed to this function plus one (for the null terminator). Then, copy the text sent as an argument to this constructor to the new dynamically allocated c-string. Then, set the textArray attribute to this newly created c-string. Function Name: ~Text (destructor) Purpose: release dynamically allocated memory for the c-string in the Text object Specifications: release the memory for the c-string pointed to by textArray Prints a message: Text destructor: Released memory for textArray. Function Name: displayText Parameters: none Returns: none (void) Purpose: print the c-string (textArray) to the screen Function Name: getText (accessor function) Parameters: none Returns: pointer to a constant character array Function Name: getLength (accessor function) Parameters: none Returns: the length of the string
Your program should allow users to load video game data from a file, save video games to a file, add a video game, remove a video game, display all video game in the library, and remove all video games. Files you will submit in your zip file This program contains multiple files as described below: Text.h - header file for a Text class, which is your own version of the C++ String Class Text.cpp - source file containing function definitions required for the Text class VideoGame.h - header file for a VideoGame class, which has data and functions describing a single video game. VideoGame.cpp - source file containing the function definitions required for the Video Game class. VideoGameLibrary.h - header file for a VideoGameLibrary class, which has data and functions describing a video game library (multiple games) VideoGameLibrary.cpp - source file containing the function definitions required for the Video GameLibrary class. Program1.cpp - this is the driver for your program which uses the Video GameLibrary class to create a Video GameLibrary object. three_ games.txt-text file given to you that contains data on three video games that you can use to test your program twenty_games.txt-text file given to you that contains data on twenty video games that you can use to test your program run Program.bat - given to you and runs the makefile and then runs the code using a testing text file - can only work on Windows operating system Makefile - makefile given to you for compiling your program TEST_FILE.txt - given to you - this is used to test your program an is used by the run Program batch file. specifications program1.cpp (driver) Your program should ask the user "How many video games can your library hold?" Once it reads in this number, it should dynamically allocate a Video GameLibrary object, sending this number as an argument. Then, the program should display a menu of six choices: What would you like to do? 1. Load video games from file. 2. Save video games to a file. 3. Add a video game. 4. Remove a video game. 5. Display all video games. 6. Remove ALL video games from this library and end program. CHOOSE 1-6: If the user chooses to load a video game from a file, then ask the name of the file and call the Video GameLibrary's load Video gamesFromFile function, sending the filename as a string If the user chooses to save video games to a file, then ask the name of the file and call the Video GameLibrary's saveToFile function, sending the filename as a string. If the user chooses to add a video game, call the Video GameLibrary's addVideo GameToArray function. If the user chooses to remove a video game, call the Video GameLibrary's remove Video GameFromArray function. if the user chooses to display all video games, call the Video GameLibrary's display Video Games function. If the user chooses to remove all video games from the library and end the program, then you should release (delete) the Video GameLibrary object and end the program. Video GameLibrary class Attributes videoGamesArray - a pointer to an array of pointers. Each pointer in the array should be able to point to (hold the memory address of) an individual Video game object. [Hint: you will need TWO stars to define this attribute. ) maxGames - this is the maximum number of video games the library can hold and is the size of the video gamesArray numGames - this is the current number of video games actually pointed to in the video GamesArray. Functions Function name: resizeVideoGameArray Parameters: none Returns: none (void) Purpose: This function is called by addVideo Game ToArray when the array size is not big enough to hold a new video game that needs to be added. The function makes the array twice as big as it currently is and then moves all the video game pointers to this new array. Function name: VideoGameLibrary constructor Parameters: An integer containing the maximum size of the video game library Purpose: This function is automatically called when a VideoGameLibrary object is created and it creates a library of video games. Specifications: The function will dynamically allocate an array of pointers to Video Game objects based on the maximum size and will also set the current number of video games to zero. Function name: VideoGameLibrary destructor Purpose: This function is automatically called when the Video Game object is destroyed. This releases the dynamically created individual video games and then deletes the array. Specifications: Prints a message "VideoGameLibrary destructor: Released memory in the vid game array and the array itself." Function name: addVideoGameToArray Parameters: none Returns: none (void) Purpose: This function should be called when you need to add a single video game to the video game library. III . . Function name: addVideoGameToArray Parameters: none Returns: none (void) Purpose: This function should be called when you need to add a single video game to the video game library. Specifications: It should ask the user for the video game title (read in as c-string, then dynamically create a Text object), platform (read in as c-string, then dynamically create a Text object), video game year (integer), video game genre (read in as c-string, then dynamically create a Text object), video game age rating (read in as c- string, then dynamically create a Text object), and IGDB user rating (between 0 and 100). Then it should dynamically allocate a new Video Game object, sending the video game data just acquired from the user as arguments to the Video Game constructor. Then, this function should check to see if numGames is equal to maxGames. If it is equal, then call the resizeVideoGameArray function. Then, it should assign this new video game to the correct pointer in the videoGamesArray. Last, it should increment numGames. . Function name: displayVideoGames Parameters: none Returns: none (void) Purpose: This function should be called when the user wants to have all the video games in the library printed to the screen. Specifications: This function loops through the video gamesArray and calls each Video game's printVideoGameDetails function. Function name: displayVideoGame Titles Parameters: none Returns: none (void) Purpose: This function should be called when you want to print only the video game titles out of the video game library (when a user wants to remove a video game from library). Specifications: This function should loop through the video gamesArray, retrieve the Video game's title by calling the Video game's getVideo Game Title function, and then printing out the title by calling the Text's displayText function. . . Function name: load Video GamesFromFile Parameters: A pointer to a character (c-string or string literal argument) containing the filename Returns: none (void) Purpose: This function should be called when the user wants to read video game data from a file and add the video games to the video game library. The file must have data in the following order: title, platform, year, genre, age rating, IGDB user rating. Specifications: This function will use a loop to read the contents of the file until reaching the end of file. For each video game, it will read the title in with a c-string and then dynamically allocate a Text to hold the title. Then it will read in the video game platforms with a c-string and then dynamically allocate a Text to hold the platform. Then it will read in the video game year. Then it will read in the video game genre with a c-string and then dynamically allocate a Text to hold the genre. Then it will read in the video game age rating with a c-string and then dynamically allocate a Text to hold the rating. Then will read in the user rating (between 0 & 100). Then, it will dynamically create a new Video Game object, sending the video game data just acquired from the user as arguments to the Video game constructor. Then, this function should check to see if numGames is equal to maxGames. If it is equal, then call the resizeVideoGameArray function. Then, it should assign this new video game to the correct pointer in the video gamesArray. Then, it should increment numGames. Then, it should print the title of the video game and say " was added to the video game library!" This should happen for each video game read from the file. At the end of the function, it should print out how many video games were read from the file & added to the library. [Note: The temporary c-string used to load in data should be able to hold a very large string - 10000 characters] Function name: remove Video Game FromArray Parameters: none Returns: none (void) Purpose: This function should be called when the user wants to remove one single video game from the video game library. The video game to be removed must is identified by the user and then removed. Specifications: This function should first make sure that the number of video games is at least 1. if not, it should print that there must always be one video game in the library and the function should end. Then, the function should call the displayVideoGame Titles function to print all the video game titles. Then, ask the user to choose a video game to remove between 1 & numGames. Once the user identifies the video game, your program should print that the video game title has been successfully deleted. Then, release the dynamically allocated space for this video game and move all array elements in video gameArray back 1 starting with this deleted video game's element. Last, decrement numGames. Function name: saveToFile Parameters: A pointer to a character (c-string or string literal argument) containing the filename Returns: none (void) Purpose: This function should be called when the user wants to print all the video game data from the video game library to a file. The data is printed in the following order (one piece of data per line): title, platform, year, genre, age rating, IGDB user rating. Specifications: Open the file with the filename that was sent to this function. Then, use a loop to go through the video gameArray and call the video game's printVideoGameDetailsToFile function, sending the file stream object to be printed to. Then, close the file and print a confirmation that all video games have been printed to the filename. . . videogame classAttributes title - a pointer to a Text object, which will hold the title of the video game platform - a pointer to a Text object, which will hold the platforms that the video game is available on year - an integer representing the year the video game was released genre - a pointer to a Text object, which will hold the genre of the video game ageRating - a pointer to a Text object, which will hold the age rating of the video game userRating - a number between 0 and 100 representing the user rating from IGDB Functions Function name: Video Game constructor Parameters: o A pointer to a Text variable, containing the title of the video game A pointer to a Text variable, containing the platform(s) of the video game O La
Step by Step Solution
There are 3 Steps involved in it
Step: 1
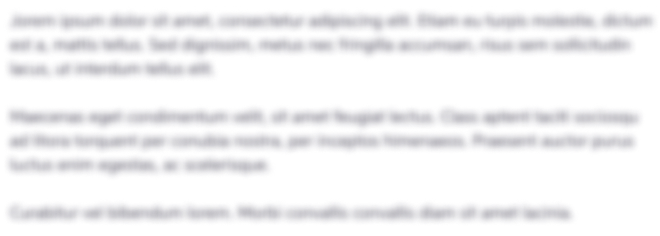
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started