Question
Need major help with Data structures, Just need help on how to set up these classes JAVA Reuse your State class from Project 1 and
Need major help with Data structures, Just need help on how to set up these classes
JAVA
Reuse your State class from Project 1 and 2 (make changes if necessary)
2. Create a class named Stack that will implement a stack of state objects using a doubleended singly linked list. Support the following methods.
a. Constructor that creates the stack.
b. A push method to push a state on the stack. (This must be an O(1) method.)
c. A pop method to pop a state off the stack and return it. (This must be an O(1)
method.)
d. A recursive printStack method to print the stack from top of the stack to bottom.
(This shant change the stack.)
e. An isEmpty method that returns true if the stack is empty, false otherwise
3. Create a class named PriorityQ that implements a priority queue of state objects using a
sorted double-ended doubly linked list. The priority again is based on COVID-19 Death
Rate (DR), the lower the DR, the higher the priority. Support the following methods:
a. Constructor that creates the priority queue.
b. An insert method to insert a State into the priority queue. (This should be an O(N)
method.)
c. A remove method to remove a State from the priority queue and return it. (This
should be an O(1) method.)
d. An intervalDelete method to delete from the priority queue state objects with
COVID-19 DR that belong to the input interval. So this method takes two input
parameters representing this interval, e.g., intervalDelete(40, 70) represent deleting
states with DRs in the interval from 40 to 70, both inclusive. Return true if anything
was found and deleted, and false otherwise.
e. A recursive printPriorityQ method to print the priority queue from highest priority
to lowest. (This shant change the priority queue.)
f. An isEmpty method that returns true if the priority queue is empty, false otherwise
COP3530 Project 3 Linked lists
Liu University of North Florida 2
4. Create a class named Project3 that will:
a. Like in project 2, we define four groups of states based on COVID-19 Death Rate:
VGOOD states as those with DR <50, GOOD no less than 50 but <100, FAIR no less
than 100 but <150, POOR no less than 150.
b. Read a file (csv) of states and create a single stack of state objects containing states
from FAIR, GOOD, and VGOOD groups (discard any states not in those groups, do
not modify the input file.).
c. Print the stack starting with the top of the stack.
d. Create a priority queue. Pop items from the stack, one at a time, and insert them to
the priority queue.
e. Print the priority queue from highest priority to lowest.
f. Repeatedly, prompt the user a short menu:
1) Enter a DR interval for deletions.
2) Print the priority queue.
3) Exit program
(Invalid user choices should be handled.)
g. When option 1) is entered, validity of such interval should be checked too. If valid,
say, the user gave interval [x, y], the intervalDelete method is called to delete states
with COVID-19 DR between x and y in the priority queue.
State class
private int cases, deaths; private double rate, MHI, CaseRate, DeathRate, cfr; private int population; private String name, capital, region; private int seats;
public State() /** * Constructor */ { this.cases = this.deaths = 0;
this.rate = 0.0; this.population = 0; this.name = this.region = ""; this.name = this.capital = ""; }
public State(String name, String capital, String region, int seats, int population, double rate, int cases, int deaths, double mHI, double CaseRate, double DeathRate, double cfr) { this.cases = cases; this.deaths = deaths; this.population = population; this.rate = rate; this.CaseRate = CaseRate; this.DeathRate = DeathRate; this.MHI= mHI; this.seats = seats; this.region = region; this.name = name; this.capital = capital; this.cfr = cfr; /** * All the varibales used in this project */ }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getCapital() { return capital; }
public void setCapital(String capital) { this.capital = capital; }
public int getPopulation() { return population; }
public void setPopulation(int population) { this.population = population; }
public double getRate() { return rate; }
public void setRate(double rate) { this.rate = rate; }
public int getCases() { return cases; }
public void setCases(int cases) { this.cases = cases; }
public int getDeaths() { return deaths; }
public void setDeaths(int deaths) { this.deaths = deaths; } /** * Getters and setters */ @Override public int compareTo(State o) { if (this.getName().compareTo(o.getName()) < 0) return -1; else if (this.getName().compareTo(o.getName()) == 0) return 0; else return 1; } /** * sends the comparisons of data to project 1 */ @Override public String toString() /** * Formats data */ { return(String.format("%20s %15f %15f %15f %15f %15f", getName(), getMHI(), getRate(), getCfr(), getCaseRate(), getDeathRate() )); }
/** * * All the getters and setters */ public String getRegion() { return region; }
public void setRegion(String region) { this.region = region; }
public int getSeats() { return seats; }
public void setSeats(int seats) { this.seats = seats; }
public double getMHI() { return MHI; }
public void setMHI(double mHI) { MHI = mHI; }
public double getCaseRate() { return CaseRate; }
public void setCaseRate(double caseRate) { CaseRate = caseRate; }
public double getDeathRate() { return DeathRate; }
public void setDeathRate(double deathRate) { DeathRate = deathRate; }
public double getCfr() { return cfr; }
public void setCfr(double cfr) { this.cfr = cfr; } } DATA from State.csv
State,Capitol,Region,US House Seats,Population,COVID-19 Cases,COVID-19 Deaths,Median Household Income,Violent Crime Rate Nevada,Carson City,West,4,3080156,256172,3658,60365,493.8 Missouri,Jefferson City,Midwest,8,6137428,431957,6201,55461,495 Massachusetts,Boston,New England,9,6892503,443193,13364,81215,327.6 North Carolina,Raleigh,South,13,10488084,650926,7825,54602,371.8 Kansas,Topeka,Midwest,4,2913314,252041,3355,59597,410.8 North Dakota,Bismarck,Midwest,1,762062,95599,1373,64894,284.6 Rhode Island,Providence,New England,2,1059361,103386,1996,67167,221.1 Mississippi,Jackson,South,4,2976149,248189,5411,45081,277.9
Step by Step Solution
There are 3 Steps involved in it
Step: 1
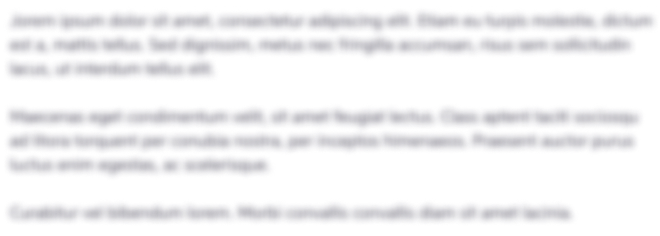
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started