Question
Need some help coding this string exercise. This file contains a set of static methodss and documentation describing them. Implement each method as described. Thank
Need some help coding this string exercise. This file contains a set of static methodss and documentation describing them. Implement each method as described. Thank you!
public class StringExercises {
/**
* Searches for "Marc" in a string.
*
* @param string a non-null string
* @return the index of the first occurrence of "Marc" in string, or -1 if not found
*/
public static int findMarc(String string) {
int index = string.indexOf("Marc");
return index;
}
/**
* Searches for a substring within a string.
* @param string a non-null string
* @param substring a non-null string
* @return the index of the first occurrence of the substring within the string, or -1 if not found
*/
public static int findSubstring(String string, String substring) {
int index = string.indexOf(substring);
return index;
}
/**
* Returns true if and only if the string contains the substring.
* @param string a non-null string
* @param substring a non-null string
* @return true if and only if the string contains the substring
*/
public static boolean contains(String string, String substring) {
int index = string.indexOf(substring);
if(index != -1) {
return true;
}
else {
return false;
}
}
/**
* Splits a string into words, using whitespace to delimit the words.
*
* See the assignment writeup for the magic argument to split().
*
* @param string a non-null string
* @return an array representing the words in the string.
*/
public static String[] splitIntoWords(String string) {
String splitString[] = string.split(" ");
return splitString;
}
/**
* Returns the substring representing the first four characters of the string.
* @param string a non-null string of length >= 4
* @return the substring representing the first four characters of the string
*/
public static String firstFour(String string) {
String result = string.substring(0, 4);
return result;
}
/**
* Returns the substring representing the first n characters of the string.
* @param string a non-null string of length >= n
* @param n an integer >= 0
* @return the substring representing the first n characters of the string
*/
public static String firstN(String string, int n) {
String result = string.substring(0, n);
return result;
}
/**
* Returns the substring representing the last four characters of the string.
* @param string a non-null string of length >= 4
* @return the substring representing the last four characters of the string
*/
public static String lastFour(String string) {
String result = string.substring(string.length()-4);
return result;
}
/**
* Returns the substring representing the last n characters of the string.
* @param string a non-null string of length >= n
* @param n an integer >= 0
* @return the substring representing the last n characters of the string
*/
public static String lastN(String string, int n) {
String result = string.substring(string.length()-n);
return result;
}
}
StringExercises.test
package string.exercises;
import static org.junit.Assert.*;
import org.junit.Rule; import org.junit.Test; import org.junit.rules.Timeout;
public class StringExercisesTest {
// Uncomment these two lines if you want to catch infinite loops // @Rule // public Timeout globalTimeout = Timeout.seconds(10); // 10 seconds
@Test public void testFindMarcEmpty() { assertEquals(-1, StringExercises.findMarc("")); }
@Test public void testFindMarcExact() { assertEquals(0, StringExercises.findMarc("Marc")); }
@Test public void testFindMarc() { assertEquals(0, StringExercises.findMarc("Marc ")); }
@Test public void testFindMarc2() { assertEquals(2, StringExercises.findMarc(" Marc ")); } @Test public void testFindSubstringEmpty() { assertEquals(-1, StringExercises.findSubstring("", "banana")); }
@Test public void testFindSubstringExact() { assertEquals(0, StringExercises.findSubstring("banana", "banana")); }
@Test public void testFindSubstring() { assertEquals(0, StringExercises.findSubstring("banana ", "banana")); }
@Test public void testFindSubstring2() { assertEquals(2, StringExercises.findSubstring(" banana ", "banana")); } @Test public void testFindSubstringBananas() { assertEquals(2, StringExercises.findSubstring(" bananabanana ", "banana")); } @Test public void testContainsEmpty() { assertFalse(StringExercises.contains("", "banana")); }
@Test public void testContainsExact() { assertTrue(StringExercises.contains("foo", "foo")); }
@Test public void testContains() { assertTrue(StringExercises.contains("foo ", "foo")); }
@Test public void testContainsEmbedded() { assertTrue(StringExercises.contains("aasdfsdf", "asdf")); } @Test public void testNotContainsEmbedded() { assertFalse(StringExercises.contains("aasdfsdf", "adsf")); } @Test public void testSplitZero() { assertArrayEquals(new String[] {""}, StringExercises.splitIntoWords("")); }
@Test public void testSplitOne() { assertArrayEquals(new String[] {"banana"}, StringExercises.splitIntoWords("banana")); }
@Test public void testSplitThree() { assertArrayEquals(new String[] {"Larry", "Moe", "Curly"}, StringExercises.splitIntoWords("Larry Moe Curly ")); }
@Test public void testFirstFourExact() { assertEquals("Kate", StringExercises.firstFour("Kate")); }
@Test public void testFirstFour() { assertEquals("Kate", StringExercises.firstFour("Katelyn")); } @Test public void testFirstN() { assertEquals("", StringExercises.firstN("Katelyn", 0)); assertEquals("K", StringExercises.firstN("Katelyn", 1)); assertEquals("Ka", StringExercises.firstN("Katelyn", 2)); assertEquals("Kat", StringExercises.firstN("Katelyn", 3)); assertEquals("Kate", StringExercises.firstN("Katelyn", 4)); assertEquals("Katel", StringExercises.firstN("Katelyn", 5)); assertEquals("Kately", StringExercises.firstN("Katelyn", 6)); assertEquals("Katelyn", StringExercises.firstN("Katelyn", 7)); }
@Test public void testLastFourExact() { assertEquals("Kate", StringExercises.lastFour("Kate")); }
@Test public void testLastFour() { assertEquals("elyn", StringExercises.lastFour("Katelyn")); } @Test public void testLastN() { assertEquals("", StringExercises.lastN("Katelyn", 0)); assertEquals("n", StringExercises.lastN("Katelyn", 1)); assertEquals("yn", StringExercises.lastN("Katelyn", 2)); assertEquals("lyn", StringExercises.lastN("Katelyn", 3)); assertEquals("elyn", StringExercises.lastN("Katelyn", 4)); assertEquals("telyn", StringExercises.lastN("Katelyn", 5)); assertEquals("atelyn", StringExercises.lastN("Katelyn", 6)); assertEquals("Katelyn", StringExercises.lastN("Katelyn", 7)); }}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
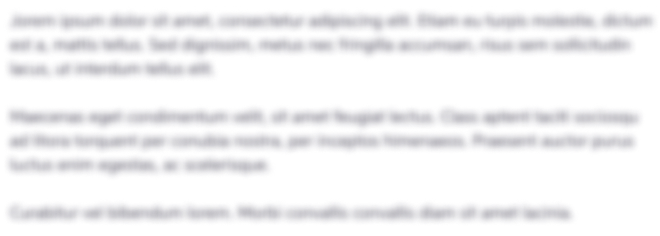
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started