Need some help for a java assignment. Some of the code is done but im missing some more of the parts needed. I need to
Need some help for a java assignment. Some of the code is done but im missing some more of the parts needed. I need to add features to the Line Drawing application i have done so far and the remaining requirments are down below and i put the code i have done down below. any help would be appreciated.
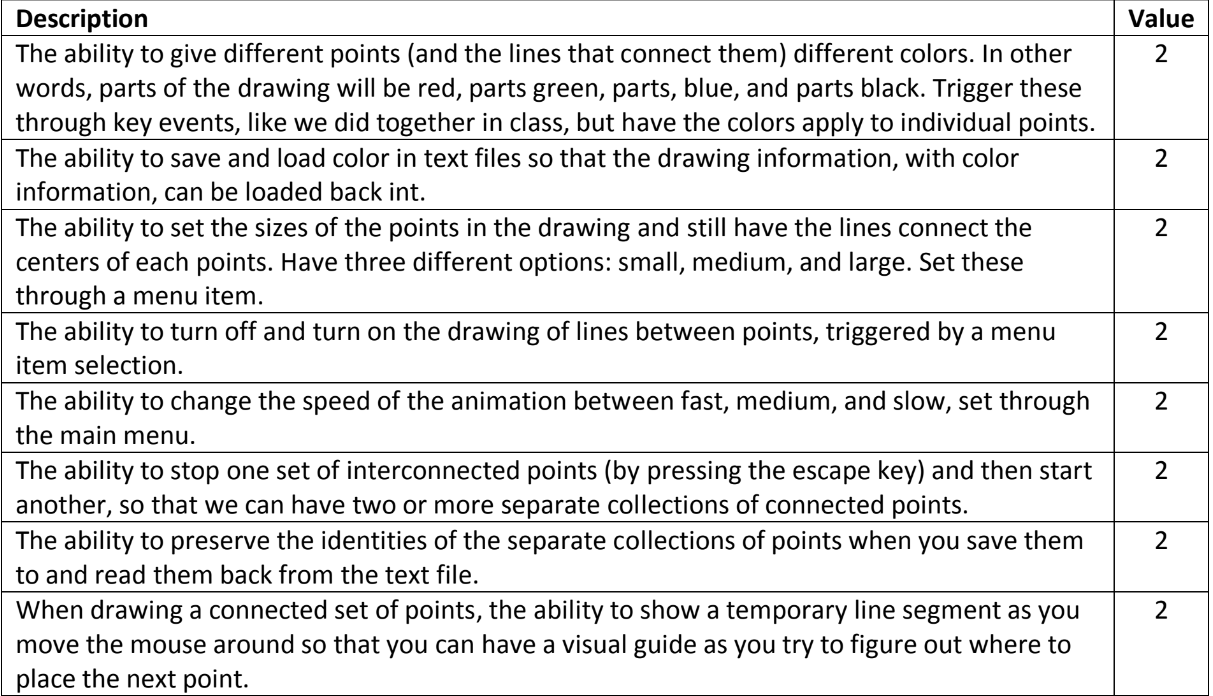
CODE:
import java.util.ArrayList; import java.util.Scanner; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JMenu; import javax.swing.JMenuItem; import javax.swing.JOptionPane; import javax.swing.JMenuBar; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.awt.Graphics; import java.awt.Container; import java.awt.BorderLayout; import java.awt.Color; import javax.swing.JButton; import java.awt.event.MouseListener; import java.awt.event.MouseMotionListener; import java.awt.event.MouseEvent; import javax.swing.Timer; import java.util.Random; import java.io.File; import java.io.PrintWriter; import java.io.FileWriter; import java.io.BufferedWriter; import javax.swing.JFileChooser;
class Point { /** * x represents the x-axis coordinate */ private int x; /** * y represents the y-axis coordinate */ private int y; public void setX(int x) { this.x = x; } public int getX() { return x; } public void setY(int y) { this.y = y; } public int getY() { return y; } public Point() { x = 0; y = 0; } public Point(int x, int y) { setX(x); setY(y); } @Override public String toString() { return String.format("%d %d", x, y); } } class LinePanel extends JPanel implements MouseListener, MouseMotionListener, KeyListener { private ArrayList points; private String message; private Color color; public LinePanel(ArrayList points) { this.points = points; addMouseListener(this); addMouseMotionListener(this); addKeyListener(this); color = Color.BLACK; setFocusable(true); message = "Click the mouse to add points."; } @Override public void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(color); Point prevPoint = null; for (Point p : points) { g.fillOval(p.getX(),p.getY(), 10, 10); if (prevPoint != null) { g.drawLine(prevPoint.getX()+5, prevPoint.getY()+5, p.getX()+5, p.getY()+5); } prevPoint = p; } g.drawString(message, 300, 300); } public void mousePressed(MouseEvent e) { Point p = new Point(e.getX(),e.getY()); points.add(p); repaint(); } public void keyTyped(KeyEvent e) {} public void keyPressed(KeyEvent e) { if (e.getKeyCode() == KeyEvent.VK_R) { color = Color.RED; repaint(); } else if (e.getKeyCode() == KeyEvent.VK_G) { color = Color.GREEN; repaint(); } else if (e.getKeyCode() == KeyEvent.VK_B) { color = Color.BLUE; repaint(); } else if (e.getKeyCode() == KeyEvent.VK_K) { color = Color.BLACK; repaint(); } } public void keyReleased(KeyEvent e) {} public void mouseClicked(MouseEvent e) {} public void mouseReleased(MouseEvent e) {} public void mouseEntered(MouseEvent e) {} public void mouseExited(MouseEvent e) {} public void mouseMoved(MouseEvent e) { requestFocusInWindow(); message = String.format("Mouse is at (%d, %d)", e.getX(), e.getY()); repaint(); } public void mouseDragged(MouseEvent e) { Point p = new Point(e.getX(),e.getY()); points.add(p); repaint(); } } class PointsIO { public boolean savePoints(ArrayList points, File f) { try { PrintWriter pw = new PrintWriter(new BufferedWriter(new FileWriter(f))); for (Point p : points) { pw.println(p); } pw.close(); return true; } catch (Exception ex) { return false; } } public boolean savePoints(ArrayList points, String fname) { try { File f = new File(fname); return savePoints(points,f); } catch (Exception ex) { return false; } } public boolean loadPoints(ArrayList points, File f) { try { // points.clear(); Scanner sc = new Scanner(f); String line; String[] parts; int x, y; Point p; while (sc.hasNextLine()) { line = sc.nextLine().trim(); if (!line.equals("")) { parts = line.split(" "); x = Integer.parseInt(parts[0]); y = Integer.parseInt(parts[1]); p = new Point(x,y); points.add(p); } } sc.close(); return true; } catch (Exception ex) { return false; } } } class LineFrame extends JFrame implements ActionListener { private ArrayList points; private Timer tim; private Random rnd; public void clearPoints() { points.clear(); repaint(); } public void actionPerformed(ActionEvent e) { int dx, dy; for (Point p : points) { dx = -10 + rnd.nextInt(20); dy = -10 + rnd.nextInt(20); p.setX(p.getX() + dx); p.setY(p.getY() + dy); } repaint(); } public void setupMenu() { JMenuBar mbar = new JMenuBar(); JMenu mnuFile = new JMenu("File"); JMenuItem miStartTimer = new JMenuItem("Start timer"); miStartTimer.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { tim.start(); } }); mnuFile.add(miStartTimer); JMenuItem miStopTimer = new JMenuItem("Stop timer"); miStopTimer.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { tim.stop(); } }); mnuFile.add(miStopTimer); JMenuItem miLoad = new JMenuItem("Load"); miLoad.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PointsIO pio = new PointsIO(); JFileChooser jfc = new JFileChooser(); if (jfc.showOpenDialog(null) == JFileChooser.APPROVE_OPTION) { if (pio.loadPoints(points,jfc.getSelectedFile())) { JOptionPane.showMessageDialog(null, "Points were read successfully."); } else { JOptionPane.showMessageDialog(null, "The points file could not be read."); } repaint(); } } }); mnuFile.add(miLoad); JMenuItem miSave = new JMenuItem("Save"); miSave.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PointsIO pio = new PointsIO(); JFileChooser jfc = new JFileChooser(); if (jfc.showSaveDialog(null) == JFileChooser.APPROVE_OPTION) { pio.savePoints(points,jfc.getSelectedFile()); }
} }); mnuFile.add(miSave); JMenuItem miExit = new JMenuItem("Exit"); miExit.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { System.exit(0); } }); mnuFile.add(miExit); mbar.add(mnuFile); JMenu mnuEdit = new JMenu("Edit"); JMenuItem miClear = new JMenuItem("Clear"); miClear.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { clearPoints(); } }); mnuEdit.add(miClear); mbar.add(mnuEdit); setJMenuBar(mbar); } public void setupUI() { setBounds(100,100,500,500); setDefaultCloseOperation(EXIT_ON_CLOSE); setTitle("Line Frame"); Container c = getContentPane(); c.setLayout(new BorderLayout()); JPanel panSouth = new JPanel(); JButton btnClear = new JButton("Clear"); btnClear.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { clearPoints(); } }); panSouth.add(btnClear); c.add(panSouth, BorderLayout.SOUTH); LinePanel panLines = new LinePanel(points); c.add(panLines, BorderLayout.CENTER); setupMenu(); } public LineFrame(ArrayList points) { this.points = points; tim = new Timer(1000, this); rnd = new Random(); setupUI(); } } public class LineDrawMain { public static void main(String[] args) { ArrayList points = new ArrayList(); LineFrame frmLines = new LineFrame(points); frmLines.setVisible(true); } }
Value Description The ability to give different points (and the lines that connect them) different colors. In other2 words, parts of the drawing will be red, parts green, parts, blue, and parts black. Trigger these through key events, like we did together in class, but have the colors apply to individual points. The ability to save and load color in text files so that the drawing information, with color information, can be loaded back int. The ability to set the sizes of the points in the drawing and still have the lines connect the centers of each points. Have three different options: small, medium, and large. Set these through a menu item. The ability to turn off and turn on the drawing of lines between points, triggered by a menu item selection. The ability to change the speed of the animation between fast, medium, and slow, set through 2 the main menu. The ability to stop one set of interconnected points (by pressing the escape key) and then start 2 another, so that we can have two or more separate collections of The ability to preserve the identities of the separate collections of points when you save them 2 to and read them back from the text file. When drawing a connected set of points, the ability to show a temporary line segment as you2 move the mouse around so that you can have a visual guide as you try to figure out where to place the next point. 2 2 connected points