Question
Need some help with Java Project a relative direction, i.e. add or subtract the current angle to the current drawing direction to get the new
Need some help with Java Project
a relative direction, i.e. add or subtract the current angle to the current drawing direction to get the new direction.
Each letter in the alphabet must have a production rule. Starting with the axiom string (iteration 0), we generate all subsequent strings by replacing the letters with copies of their production rules.
For iteration n=0, we get F: (the axiom is a straight line starting at (x,y)=(0,0) and oriented at 0 degrees with length=1, i.e. along the positive X axis). Although, each line segment has length 1.0 we scale this later to fit the fractal curve into our canvas dimensions.
For iteration n=1 we get: F+F-F-F+F, where F is replaced by 1 occurrence of the rule. For this string we move forward in the leftward direction, change the direction by +90 degrees, move forward in this degree direction (upwards), change the direction by -90 degrees, move forward in this direction (leftward), change the direction by -90 degrees, move forward in this direction (downward), change the direction the direction by +90 degrees again and finally move forward in this direction (leftward).
For iteration n=2 we get: F+F-F-F+F + F+F-F-F+F - F+F-F-F+F - F+F-F-F+F + F+F-F-F+F, where each F in iteration n=1 is replaced by F+F-F-F+F. Notes the + and - symbols are never changed. It is now to tedious to specify all the drawing actions that need to be performed!
For iteration n=3 we get:
F+F-F-F+F+F+F-F-F+F-F+F-F-F+F-F+F-F-F+F+F+F-F-F+F + F+F-F-F+F+F+F-F-F+F-F+F-F-F+F-F+F-F-F+F+F+F-F-F+F - F+F-F-F+F+F+F-F-F+F-F+F-F-F+F-F+F-F-F+F+F+F-F-F+F - F+F-F-F+F+F+F-F-F+F-F+F-F-F+F-F+F-F-F+F+F+F-F-F+F + F+F-F-F+F+F+F-F-F+F-F+F-F-F+F-F+F-F-F+F+F+F-F-F+F
We can use a prettyPrint method in the DrawFractal.java DrawFractal.java class to print such strings. For higher values of n it becomes takes considerable more space to write out the result string.
The turtle moves about the screen drawing straight lines at various orientations. The turtle interprets a character string as a sequence of line segments, connected "head to tail" to each other. Depending on the segment lengths and angles between them, the resulting figure can become quite complex but always consists of multiple single lines drawn at different orientations.
We can use a stack to build these complex strings in an iterative fashion. At each iteration, we parse a string (the axion initially at iteration 0) and do the following for each character, going left to right:
If a character is a "-", "+", "[" or "]" push it unto the stack.
If the character is a F or G or X or Y, replace these characters by pushing the characters in the corresponding production rule(s) for those symbols onto the stack in the correct order.
Once the complex string has been processed in this way from the left to the right, we build a new string, s, by popping the stack one string at a time and concatenating the popped string to the left of the string variable s, that is initially the empty string.
To allow for the branching structures found in many plants (trees and bushes), two new constants, "[" and "]", are used to denote a branch. They are interpreted by the turtle as follows:
If "[" is encountered, four pieces of information giving the current position (the x and y coordinates) and drawing angle and line length (relative direction and length) are pushed onto a stack as floating point numbers (doubles).
If "]" is encountered, the current position and drawing angle and line length are popped off the stack and used to reset by the current position and angle/length variables. The turtle now begin drawing from this position.
After a number of iterations we have built a (quite possibly) very complex string of symbols. Then we use a second stack to draw the stack. We process the characters in the string from left to right. We ignore blanks and non-drawable symbols like X and Y. Drawable symbols like "F" and "G" cause a straight line of some length to be printed in some direction. Each line is output as an object. These objects are maintained in an array. Each line (see the class LineInfo.java) has double variables for x1 and y1 and x2 and y2 (the line endpoints) and are represented via line objects. We compute an array of these line objects. We also compute the minimum and maximum x and y values as we process this string. We do this via an update method UpdateXminYminXmaxYmax(x1,y1,x2,y2) as we process each character in the string. The first x1 and y1 we encounter can be used to initialize the minimum and maximum x and y variables. Subsequent x1 and y1 and x2 and y2 values can be used to update these minimum and maximum x and y variables. Note that this method is supplied to you in DrawFractal.java The x and y values can be negative as well as positive and can be quite large. As all lines are computed, we compute the minimum and maximum x and y values to scale these lines so that they fit on a window with lower left coordinates (lowerX,lowerY)=(0,0) and upper right coordinates (upperX,upperY)=(1023,1023) [for a 1024*1024 image). When symbol "[" is encountered the current drawing x and y coordinates, the current drawing direction (angle) and the current drawing length are used to generate an object holding this information and that object is pushed onto a stack. Drawing continues with next symbols, maybe after scaling the current drawing length. When symbol "]" is encountered the top object if popped from the stack and the current location, current drawing length and current drawing direction (angle) are restored.
The Main.java class is provided for you. Its main method contains the Java code needed to setup 6 fractal curves and prints them on a large canvas and to save them as jpg images. The canvas class is given by the class Canvas.java. The main method requires 4 line arguments, see Tutorial 2 on the course web page to see how to do this in Eclipse.
You need to write methods for 2 classes for this assignment, a shell class given in MakeFractal.java and another shell program given inDrawFractal.java
You need to write a method buildFractal() for MakeFractal.java. This method uses a stack to perform the iterations to build the final complex fractal string You process symbols (as Strings) left to right, symbols with production rules are replaced with those rules (and these symbols are pushed onto the stack) while other symbols are just pushed without substitution. At the end of iterations we pop the stack and concatenate it to the front (of an initially empty) string to represent the fractal.
You also need to write a method computeLines in the class DrawFractal.java that takes the string representing the fractal and produces an array of line objects that draw the fractal. A stack is needed to process the symbols "[" and "]" (push and pop) via a stack to handle branching.
The class for storing line information as objects is given in LineInfo.java. Instances of this class are the objects representing all the lines to be drawn for the fractal. We can maintain these in an array of these objects with an extendCapacity helper class (to expand the array size as needed). Another class for storing the current pixel information (coordinates, current drawing length and current drawing direction) when pushing or popping to get branching (necessary for trees and bushes) is given in CurrentPointInfo.java. Class definitions for the Linked List Stack, that we can use without being concerned with its implementation, are given here: EmptyCollectionException.java (needed by the pop method), LinearNode.java , LinkedStack.java and StackADT.java .
The Koch snowflake (see fractal1 in Main.java) can be generated using the L system:
alphabet: F
constants: + - [ ]
axiom: +F--F--F
rules: (F-F--F+F)
angle: 25 degrees
n: 4 iterations
cmd1: java Main kochSnowflake.jpg 1024 1024 1
The Koch curve (see fractal2 in Main.java) can be generated using the L system:
alphabet: F
constants: + -
axiom: F
rules: (F+F-F-F+F
angle: 90 degrees
n: 5 iterations
cmd2: java Main kochcurve.jpg 1024 1024 2
The Sierpinski Triangle (see fractal3 in Main.java) can be generated using L system: pre>
alphabet: F G
li> constants: + -
axiom: F-G-G
rules: (F-G+F+G-F),(G-GG)
angle: 120 degrees
n: 8 iterations
cmd3: java Main sierpinskiTriangle.jpg 1024 1024 3
A Dragon curve (see fractal4 in Main.java) can be generated using L system:
alphabet: X Y
constants: + -
axiom: FX
rules: (X+YF)(FX-Y)li>
angle: 90 degrees
n: 12 iterations
cmd4: java Main dragonCurve.jpg 1024 1024 4
A fractal plant (see fractal5 in Main.java) can be generated using the L system:
alphabet: X F
constants: + - [ ]
axiom: -X
rules: (F-[[X]+X]+F[+FX]-X)(FF)
angle: 25 degrees
n: 8 iterations
cmd5: java Main fractalPlant.jpg 1024 1024 5
A fractal bush (see fractal6 in Main.java) can be generated using the L system:
alphabet: F
constants: + - [ ]
axiom: +++++++++++++++++F
rules: (FF-[-F+F+F]+[+F-F-F])
angle: 16 degrees
n: 6 iterations
cmd6: java Main fractalBush.jpg 1024 1024 6
The last 3 tree fractals (see http://www.kevs3d.co.uk/dev/lsystems/) are made from:
alphabet: F X
constants: + - [ ]
axiom: ----FX
rules: (FF-[-F+F]+[+F-F]), (FF+[+F]+[-F])
angle: 22.5 degrees
n: 5 iterations
cmd7: java Main tree1.jpg 1024 1024 7
alphabet: X F
constants: + - [ ]
axiom: ----X
rules: (F-[[X]+X]+F[+FX]-X),(FF)
angle: 22.5 degrees
n: 6 iterations
cmd8: java Main tree2.jpg 1024 1024 8
alphabet: X F
constants: + - [ ]
axiom: F
rules: (FF[-F++F][+F--F]++F--F)
angle: 25 degrees
n: 6 iterations
cmd9: java Main tree3.jpg 1024 1024 9
here is the supplied code
Main.java --------- supplied to you, do not change. Usage command executed when the number of arguments is 0, prints a string telling the user the required number and type of arguments required, An example list of 9 line commands to print the 9 fractals is then given. For each fractal: the data is setup: symbols, production rules for symbols, scalingFactor (always 1 for this assignment), drawingAngle, axion, number of iterations Then code that builds and draws the specified fractal is invoked. Canvas.java ----------- provides a 1024 by 1024 white canvas to draw the fractal on and save as a jpg file. EmptyCollectionException.java ----------------------------- The exception thrown if an empty statack is popped LineInfo.java ------------- Generates an line object with getters and setters for a line from (x1,y1) to (x2,y2) LinearNode.java, LinkedStack.java and StackADT.java --------------------------------------------------- Classes for a linked list stack implementation. Understanding the LL implementation of stacks is not needed here (but know this for the exam). Just use the stack interface in StackADT.java CurrentPointInfo.java --------------------- The currect drawing position, x amd y, and the drawingLength (always 1 in this asignment) and the drawingDirection. MakeFractal.java ---------------- -mostly provided for you. Generates a MakeFractal object with data and methods associated with this object buildFractal method: you have to write this: { n=0 the computedFractal string is the axion for iter=1:n { for i=0:length of computedFratcal { process each i'th character of computedFractal as follows: { q=computedFractal.substring(i,i+1) Is q a symbol: F, G, X, Y, +,=,[,]? You can use integer method in to determine this. If method in returns NOT_FOUND (-1) push(q) if method in retuns an integer >= 0 This is the symbol index in the symbols array. Use the symbol index to get the production rule (rules(index)) and push(rules(index)) rather than q } } Set comutedFractal to "", the empty string Until the stack is empty, { pop the stack concatenate each popped item to the left of the string computedFractal } This is the new value of computedFractal after this iteration } return computedFractal; } method in does as just explained above method prettPrint prints the string computedFractal 60 characters per line DrawFractal.java ---------------- ok, we have computedFractal, now we have to draw it. You are required to write method computeLines() { Process fractalString character by character { symbol=fractalString.substring(i,i+1) is the i'th character if the symbol is "F" or "G" { store the line data in the lines array: expand capacity if need be, x2=x1+length*Math.cos(drawingDir); y2=y1+length*Math.sin(drawingDir); use LineInfo(x1,y1,x2,y2) to generate a line object and store in lines array call UpdateXminYminXmaxYmax(x1,y1,x2,y2) to update the min and max x and y values } if the symbol is + { drawingAlgle+angle } if the symbol is - { drawingAngle-angle } if the symbol is "X" or "Y" or " " do nothing if the symbol is "[" { use CurrentPointInfo to save x1,x2,drawingAngle, and drawingLength push this object (so you need a stack of this type) } if the symbol is "]" { pop the top of the stack holding the CurrentPointInfo data and use that object's getters to restore x1,x2, drawingAngle,drawingLength } } method printAllLines (provided) - prints the coordinate information for each line. method drawAllLines (provised) - draw the scaled and shifted fractal on the canvas method expandCapacity() - you know what this does!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
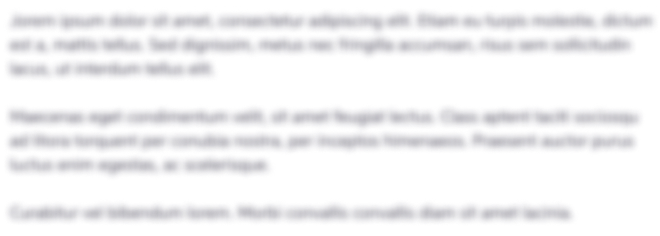
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started