Question
Need the bonus part of this question answered. Code that was done for the previous parts that i did already, are provided down below. Feel
Need the bonus part of this question answered. Code that was done for the previous parts that i did already, are provided down below. Feel free to change anything in my code to answer the question or to fix it. All code in java.
=======================Student.java====================
package student_grade;
@SuppressWarnings("rawtypes")
public class Student implements Comparable {
private String firstName;
private String lastName;
private int pID;
public Student(String aFirst, String aLast, int apID) {
firstName = aFirst;
lastName = aLast;
pID = apID;
}
public int getID() {
return pID;
}
public String getFirst() {
return firstName;
}
public String getLast() {
return lastName;
}
public int compareTo(Object s) {
if (lastName.compareTo(((Student) s).getLast()) != 0)
return lastName.compareTo(((Student) s).getLast());
else if (firstName.compareTo(((Student) s).getFirst()) != 0)
return firstName.compareTo(((Student) s).getFirst());
else
return pID - ((Student) s).getID();
}
}
=====================================Grade.java============================
package student_grade;
public class Grade {
private String letter;
public Grade(String grade) {
letter = grade;
}
public String getLetter() {
return letter;
}
public double getValue() {
switch (letter) {
case "A":
return 4;
case "A-":
return 3.667;
case "B+":
return 3.333;
case "B":
return 3;
case "B-":
return 2.667;
case "C+":
return 2.333;
case "C":
return 2;
case "C-":
return 1.667;
case "F":
return 0;
}
return 0;
}
}
===========================CSVUtil.java===============================
package student_grade;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Map;
public class CSVUtil {
private static final String CSV_SPLIT_BY = ",";
public void read(String filename, Map
BufferedReader br = null;
String line = "";
try {
br = new BufferedReader(new FileReader(filename));
while ((line = br.readLine()) != null) {
// use comma as separator
String[] data = line.split(CSV_SPLIT_BY);
Student student = new Student(data[0], data[1], Integer.valueOf(data[2]));
Grade grade = new Grade(data[3]);
map.put(student, grade);
}
}
catch (FileNotFoundException e) {
e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
finally {
if (br != null) {
try {
br.close();
}
catch (IOException e) {
e.printStackTrace();
}
}
}
}
public void write(String filename, Map
BufferedWriter bw = null;
try {
bw = new BufferedWriter(new FileWriter(filename));
for (Map.Entry
Student student = itr.getKey();
Grade grade = itr.getValue();
bw.write(student.getFirst());
bw.write(CSV_SPLIT_BY);
bw.write(student.getLast());
bw.write(CSV_SPLIT_BY);
bw.write(String.valueOf(student.getID()));
bw.write(CSV_SPLIT_BY);
bw.write(grade.getLetter());
bw.newLine();
}
bw.flush();
}
catch (FileNotFoundException e) {
e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
finally {
if (bw != null) {
try {
bw.close();
}
catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
===============================GradeBooking.java=================
package student_grade;
public interface GradeBooking {
void csvImport(String filename);
void csvExport(String filename);
void csvExport(String filename, Grade grade);
void addGrade(String firstName, String lastName, int pID, String grade);
Grade findGrade(String firstName, String lastName, int pID);
void removeStudent(String firstName, String lastName, int pID);
double findAverage();
}
==========================HashGradeBook.java==============================
package student_grade;
import java.util.HashMap;
import java.util.Map;
import java.util.NoSuchElementException;
public class HashGradeBook implements GradeBooking {
private Map
private CSVUtil csvUtil;
public HashGradeBook() {
map = new HashMap();
csvUtil = new CSVUtil();
}
@Override
public void csvImport(String filename) {
csvUtil.read(filename, map);
}
@Override
public void csvExport(String filename) {
csvUtil.write(filename, map);
}
@Override
public void csvExport(String filename, Grade grade) {
Map
for (Map.Entry
if (grade.equals(itr.getValue())) {
filteredStudent.put(itr.getKey(), itr.getValue());
}
}
csvUtil.write(filename, filteredStudent);
}
@Override
public void addGrade(String firstName, String lastName, int pID, String grade) {
Student student = new Student(firstName, lastName, pID);
Grade newGrade = new Grade(grade);
map.put(student, newGrade);
}
@Override
public Grade findGrade(String firstName, String lastName, int pID) {
Student student = new Student(firstName, lastName, pID);
if (!checkStudentAvailability(student)) {
throw new NoSuchElementException("Student is not found...");
}
return map.get(student);
}
@Override
public void removeStudent(String firstName, String lastName, int pID) {
Student student = new Student(firstName, lastName, pID);
if (checkStudentAvailability(student)) {
map.remove(student);
}
}
@Override
public double findAverage() {
double average = 0;
if (map.isEmpty()) {
throw new NoSuchElementException("No data available...");
}
for (Map.Entry
average += itr.getValue().getValue();
}
return average;
}
private boolean checkStudentAvailability(Student student) {
for (Map.Entry
if(itr.getKey().compareTo(student)==0){
return true;
}
}
return false;
}
}
=============================TreeGradeBook.java=========================
package student_grade;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.TreeMap;
public class TreeGradeBook implements GradeBooking {
private Map
private CSVUtil csvUtil;
public TreeGradeBook() {
map = new TreeMap();
csvUtil = new CSVUtil();
}
@Override
public void csvImport(String filename) {
csvUtil.read(filename, map);
}
@Override
public void csvExport(String filename) {
csvUtil.write(filename, map);
}
@Override
public void csvExport(String filename, Grade grade) {
Map
for (Map.Entry
if (grade.equals(itr.getValue())) {
filteredStudent.put(itr.getKey(), itr.getValue());
}
}
csvUtil.write(filename, filteredStudent);
}
@Override
public void addGrade(String firstName, String lastName, int pID, String grade) {
Student student = new Student(firstName, lastName, pID);
Grade newGrade = new Grade(grade);
map.put(student, newGrade);
}
@Override
public Grade findGrade(String firstName, String lastName, int pID) {
Student student = new Student(firstName, lastName, pID);
if (!map.containsKey(student)) {
throw new NoSuchElementException("Student is not found...");
}
return map.get(student);
}
@Override
public void removeStudent(String firstName, String lastName, int pID) {
Student student = new Student(firstName, lastName, pID);
if (map.containsKey(student)) {
map.remove(student);
}
}
@Override
public double findAverage() {
double average = 0;
if (map.isEmpty()) {
throw new NoSuchElementException("No data available...");
}
for (Map.Entry
average += itr.getValue().getValue();
}
return average;
}
}
==============================StudentGradleHandler.java===========================
package student_grade;
public class StudentGradleHandler {
public static void main(String[] args) {
HashGradeBook book = new HashGradeBook();
book.csvImport("student.csv");
book.csvExport("output.csv");
book.addGrade("Chirs", "Gayle", 222324, "C+");
System.out.println("Grade for Chirs Gayle is: " + book.findGrade("Chirs", "Gayle", 222324));
System.out.println("Average to Hash Grade Book: " + book.findAverage());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
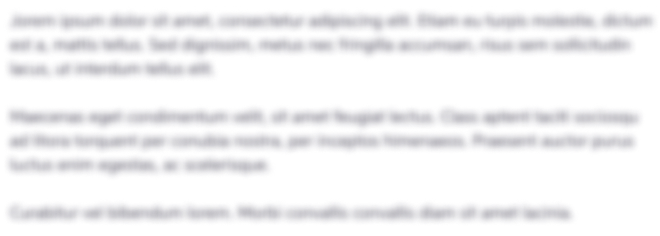
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started