Question
need to complete the following program in JAVA I have already provided code below please use only that code as I have already been working
need to complete the following program in JAVA I have already provided code below please use only that code as I have already been working with this
/**
* Write a description of class RC4 here.
*
* @author (your name)
* @version (a version number or a date)
*/
import java.util.ArrayList;
import java.util.Collections;
public class RC4 {
/**
* Encrypt plaintext with key. Place output in ciphertext.
*/
public void encrypt(ArrayList plaintext, ArrayList key,
ArrayList ciphertext) {
//Ensure key length is between 5 to 16 bytes
assert key.size() >= 5 : "Key too short";
assert key.size() <= 16 : "Key too long";
//Create our state s and set key schedule
ArrayList s = new ArrayList();
genKSA(key, s);
//create a key stream and call genKeyStream() to create the key bytes
// according to the schedule s
ArrayList keyStream = new ArrayList();
for (int i = 0; i < 256; i++)
keyStream.add(i);
genKeyStream(s, keyStream, plaintext.size());
//For debugging
System.out.print("keyStream: ");
for (int c=0; c < plaintext.size(); c++)
System.out.print(Integer.toHexString(keyStream.get(c))+" ");
System.out.println();
//encrypt the plaintext using the key stream
for (int i = 0; i < plaintext.size(); i++)
{
ciphertext.add(plaintext.get(i) ^ keyStream.get(i));
}
}
/**
* Decrypt cyphertext with key. Place output in plaintext.
*/
public void decrypt(ArrayList ciphertext, ArrayList key,
ArrayList plaintext) {
//Need decrytion code
}
/**
* Generate the schedule s corresponding to the key.
*/
private void genKSA(ArrayList key, ArrayList s) {
//This code needs to be done
}
/**
* Generate a key stream keyStream of numBytes bytes, using the schedule s.
*/
private void genKeyStream(ArrayList s, ArrayList keyStream,
int numBytes) {
// need to generate the key stream code for this part
}
/**
* Encrypt the phrase "Attack at dawn" using key "Secret". Use the
* ciphertext and the key to recover the plaintext in order to verify your
* answer.
*
* DO NOT MODIFY
*/
public static void main(String[] args) {
RC4 rc4 = new RC4();
//Use "Secret" as the key
ArrayList key = new ArrayList();
String k = "Secret";
for (int i=0; i < k.length(); i++) {
key.add((int)k.charAt(i));
}
//Set up our plaintext "Attack at dawn"
ArrayList pt = new ArrayList();
String p = "Attack at dawn";
for (int i=0; i < p.length(); i++) {
pt.add((int)p.charAt(i));
}
//Call the encrypt() method and print out the hex representation
ArrayList ct = new ArrayList(pt.size());
rc4.encrypt(pt, key, ct);
System.out.print("Ciphertext: ");
for (int c=0; c < ct.size(); c++)
System.out.print(Integer.toHexString(ct.get(c))+" ");
System.out.println();
//Call the decrypt() method and reproduce the original plaintext
pt.clear();
rc4.decrypt(ct, key, pt);
System.out.print("Recovered plaintext: ");
for (int c=0; c < pt.size(); c++)
System.out.print((char) pt.get(c).intValue());
System.out.println();
}
}
Need to fill in decryption code, genKSA, and genKeyStream please see those methods
Step by Step Solution
There are 3 Steps involved in it
Step: 1
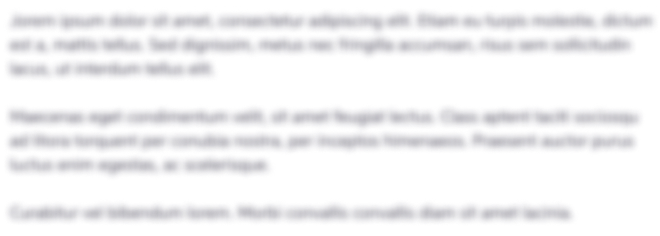
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started