Question
Need to create JUnit Test Case for code below: package code; /** The BankAccount class stores data about a bank account for the BankAccount and
Need to create JUnit Test Case for code below:
package code; /** The BankAccount class stores data about a bank account for the BankAccount and SavingsAccount Classes programming challenge. There is a problem with Deposits and Withdrawals. Step through the program via the Debugger and determine what and where the problem is. Then comment out the bad code and put in new code to fix the problem, and add a comment above your change. */
public class BankAccount { private double balance; // Account balance private int numDeposits; // Number of deposits private int numWithdrawals; // Number of withdrawals private double interestRate; // Interest rate private double monthlyServiceCharges; // Service charges
/** The constructor initializes the account with a balance, an interest rate, and monthly service charges. @param bal The balance. @param intRate The interest rate. @param mon The monthly service charges. */
public BankAccount(double bal, double intRate, double mon) { balance = bal; interestRate = intRate; monthlyServiceCharges = mon; numDeposits = 0; numWithdrawals = 0; }
/** The deposit method makes a deposit into the account. @param amount The amount to deposit. */
public void deposit(double amount) { balance += amount; numDeposits++; }
/** The withdraw method withdraws an amount from the account. @param amount The amount to withdraw. */
public void withdraw(double amount) { balance -= amount; numWithdrawals++; }
/** The calcInterest method calculates the monthly interest and adds it to the account balance. */ private void calcInterest() { // Get the monthly interest rate. double monIntRate = interestRate / 12; // Get the amount of interest for the month. double monInterest = balance * monIntRate; // Add the interest to the balance. balance += monInterest; }
/** The monthlyProcess method subtracts the monthly service charge from the account balance and adds the monthly interest. The number of deposits and number of withdrawals are set to 0. */ public void monthlyProcess() { // Subtract the monthly service charges // from the balance. balance -= monthlyServiceCharges; // Calculate and add the interest for // the month. calcInterest(); // Reset the number of deposits and // withdrawals to zero. numDeposits = 0; numWithdrawals = 0; } /** The setMonthlyServiceCharges method sets the monthly service charge to a specified amount. @param m The amount of monthly service charge. */ public void setMonthlyServiceCharges(double m) { monthlyServiceCharges = m; }
/** The getBalance method returns the account balance. @return The account balance. */ public double getBalance() { return balance; }
/** The getNumDeposits method returns the number of deposits. @return The number of deposits. */ public int getNumDeposits() { return numDeposits; }
/** The getNumWithdrawals method returns the number of withdrawals. @return The number of withdrawals. */ public int getNumWithdrawals() { return numWithdrawals; }
/** The getInterestRate method returns the interest rate. @return The interest rate. */ public double getInterestRate() { return interestRate; }
/** The getMonthlyServiceCharges method returns the monthly service charges @return The motnhly service charges. */ public double getMonthlyServiceCharges() { return monthlyServiceCharges; } }
package code; /** The BankAccount class stores data about a bank account with savings capabilites for the BankAccount and SavingsAccount Classes programming challenge. */
public class SavingsAccount extends BankAccount { private boolean status; // Active or inactive /** The constructor initializes the account with a balance, an interest rate, and monthly service charges. If the balance is less than $25 the account is set as inactive. Otherwise it is set as active. @param bal The balance. @param intRate The interest rate. @param mon The monthly service charges. */
public SavingsAccount(double bal, double intRate, double mon) { super(bal, intRate, mon); if (bal < 25.0) status = false; // Inactive else status = true; // Active }
/** The withdraw method withdraws an amount from the account if the account is active. If the account is inactive, no withdrawal is made. (Overrides the super class method.) @param amount The amount to withdraw. */ public void withdraw(double amount) { if (status) { super.withdraw(amount); if (getBalance() < 25) status = false; } } /** The deposit method makes a deposit into the account. If the account is inactive and the deposit raises the balance to $25 or more, the account is made active. (Overrides the super class method.) @param amount The amount to deposit. */ public void deposit(double amount) { super.deposit(amount); if (!status) { if (getBalance() >= 25) status = true; } }
/** The monthlyProcess method calls the super class's monthlyProcess method. If the number of withdrawals is greater tha n 4, the monthly service charges are increased. */ public void monthlyProcess() { double msc; // Monthly service charge if (getNumWithdrawals() > 4) { // Get the monthly service charges. msc = getMonthlyServiceCharges(); // Increase the monthly service charges. setMonthlyServiceCharges(msc + (getNumWithdrawals() - 4)); // Do the monthly processing. super.monthlyProcess(); // Set the monthly charges back. setMonthlyServiceCharges(msc); } else super.monthlyProcess(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
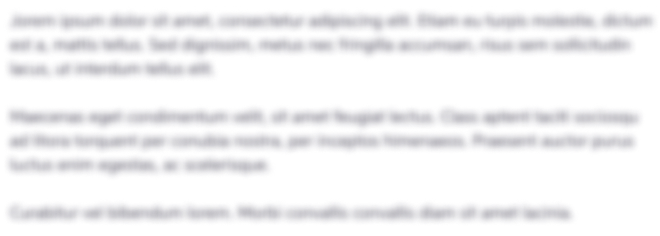
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started