Question
Need to done in C++ Part 1. Rewrite the menu option to display account information to use for_each instead of iterators. Your loop variable should
Need to done in C++
Part 1.
Rewrite the menu option to display account information to use for_each instead of iterators. Your loop variable should be a reference in order to avoid copying the objects in your container. You should not use a ranged for loop for this part of the task.
Part 2.
Add a menu option to remove an account from the system. Use remove_if to perform this operation. After calling remove_if, erase the removed elements permanently from the container using erase.
Note: When using a lambda function, if you need to pass in a variable from outside the lambda function, such as the ID number, look at the following syntax. The variable id is captured by putting it in the square brackets, which allows it to be accessed inside the lambda function. Note also the use of the & symbol to indicate pass-by-reference to avoid copying the list_item.
int id = 1; auto end = remove_if(my_list.begin(), my_list.end(), [id](auto& list_item){ return id == list_item.get_id(); } Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account Your choice: (input1) Account ID: 0 Name: George Balance: $100.00 Account ID: 1 Name: Harry Balance: $200.00 Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account Your choice: (input6) Enter account ID to remove: (input 1) Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account Your choice: (input1) Account ID: 0 Name: George Balance: $100.00
Part 3.
Add a menu option to determine the total amount of deposits in the system. Use accumulate to accomplish this part of the task.
You might want to add a get_balance method to your class to help accomplish this.
Note that you should use 0.0f as the initial starting value for the accumulate function to ensure it returns a floating point number rather than an integer.
Example input and output Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account 7. Show total balance for all accounts Your choice: (input7) Total in all accounts: $300.00
Part 4.
Add a menu option to add a dividend to all of the accounts. The dividend is calculated as a simple percentage of the current balance. Have the user enter the amount of the dividend as a percentage. Use transform to accomplish this task. You could add a new operator *= or a get_balance method to your account class to make this task easier.
Example input and output:
Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account 7. Show total balance for all accounts 8. Add a dividend to all accounts Your choice: (input8) Enter the dividend as a percentage: (input 5) Account Menu: 0. Quit Program 1. Display Account Information 2. Add a deposit to an account 3. Withdraw from an account 4. Add new account 5. Find account by ID 6. Remove account 7. Show total balance for all accounts 8. Add a dividend to all accounts Your choice: (input1) Account ID: 0 Name: George Balance: $105.00 Account ID: 1 Name: Harry Balance: $210.00
;---------------------------------------------------------------------------------------------------------------------------
#include
using namespace std;
class Account { public: string name; double balance; Account(string n, double b) : name(n), balance(b) {} };
void displayAccountInfo(list
void addDeposit(list
int i = 0; list
for (it = accounts.begin(); it != accounts.end(); it++) { if (i == nameid) { it->balance += deposit; cout << "Deposit added successfully" << endl; return; } i++; } cout << "Account not found" << endl; }
void withdraw(list
int i = 0; list
for (it = accounts.begin(); it != accounts.end(); it++) { if (i == nameid) { if (it->balance < withdraw) { cout << "Insufficient funds" << endl; return; } it->balance -= withdraw; cout << "Withdrawal successful" << endl; return; } i++; } cout << "Account not found" << endl; }
void addAccount(list
accounts.emplace_back(name, balance); cout << "Account created successfully" << endl; }
void findAccountById(list
int main() { list
while (true) { int choice; cout << "Main Menu:" << endl; cout << "0. Quit Program" << endl; cout << "1. Display account information" << endl; cout << "2. Add a deposit to an account" << endl; cout << "3. Withdraw from an account" << endl; cout << "4. Add new account" << endl; cout << "5. Find account by ID" << endl; cout << "Your choice: "; cin >> choice; if (choice == 0) { break; } else if (choice == 1) { if (accounts.empty()) { cout << "No accounts found" << endl; continue; } displayAccountInfo(accounts); } else if (choice == 2) { if (accounts.empty()) { cout << "No accounts found" << endl; continue; } addDeposit(accounts); } else if (choice == 3) { if (accounts.empty()) { cout << "No accounts found" << endl; continue; } withdraw(accounts); } else if (choice == 4) { addAccount(accounts); } else if (choice == 5) { if (accounts.empty()) { cout << "No accounts found" << endl; continue; } findAccountById(accounts); } else { cout << "Invalid choice. Please try again." << endl; } } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
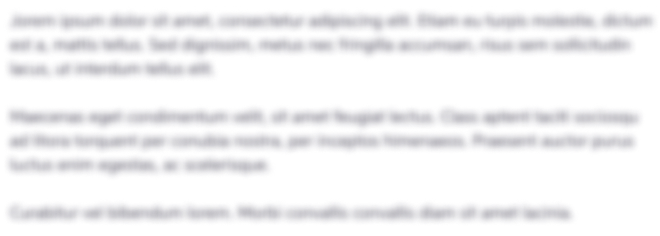
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started