Question
No coding is required for this part. 1. Given an priority queue's empty heap, draw the heap tree structure after each step: -Inserting Zach, Sean,
No coding is required for this part.
1. Given an priority queue's empty heap, draw the heap tree structure after each step:
-Inserting Zach, Sean, Nathan, Crystal
-Then insert Tanner, Kari, Jess
-Then do a remove
2. Add a public method to Priority Queue class that is called CountChildren. It is passed a priority value and returns the number of children nodes that are in the sub-tree that has a root equal to the priority value passed to the method. It should call the following private method and pass the priority value and the heap's root position.
Add a recursive private method, called CountChildren, that accepts a priority value and a tree position. Is must return the number of nodes within a sub-tree where the sub-trees root node has a Value that equals the priority value passed into the method. Do not include the parent node in your count.
given code: for #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace ConsoleApplication1 { public class OurPriorityQueue
private OurEntry
public OurPriorityQueue(int size = 100) { if (size
mTable = new OurEntry
public bool isEmpty() { return mCount == 0; }
public void Clear() { mCount = 0; }
public TValue Peek() { if (isEmpty() == true) throw new ApplicationException("Can't Peek an empty OurPriorityQueue");
return mTable[1].Value; }
public void Add(TPriority aPriority, TValue aValue) { //if( mCount == mTable.Length - 1 ) // m_array.resize( m_array.size( ) * 2 ); if (isFull() == true) return;
// Percolate up int hole = ++mCount; for (; hole > 1 && aPriority.CompareTo(mTable[hole / 2].Priority) (aPriority, aValue); }
public TValue Remove() { if (isEmpty() == true) throw new ApplicationException("Can't Remove from an empty OurPriorityQueue");
TValue value = mTable[1].Value; mTable[1] = mTable[mCount--]; percolateDown(1);
return value; }
private void percolateDown(int hole) { int child; OurEntry
for (; hole * 2
public void buildHeap() { for (int i = mCount / 2; i > 0; i--) percolateDown(i); }
public StringBuilder InOrder() { return InOrder(1); } private StringBuilder InOrder(int position) // why?? What does this do?? { StringBuilder str = new StringBuilder(); if (position
Example:
CountChildren(1) returns 5
CountChildren(2) returns 2
CountChildren(3) returns 1
CountChildren(5) returns 0
This is only an example! The tree can be any size.
3 5 2 4 3 5 2 4Step by Step Solution
There are 3 Steps involved in it
Step: 1
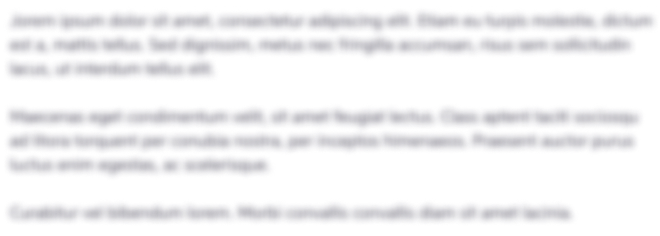
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started