Answered step by step
Verified Expert Solution
Question
1 Approved Answer
NodeDriverTest import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.Test; class NodeDriverTest { @Test void testBuildChain() { int[] data = { 5, 17, 11, 10 }; Node head =
NodeDriverTest
import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.Test; class NodeDriverTest { @Test void testBuildChain() { int[] data = { 5, 17, 11, 10 }; Node head = NodeDriver.buildChain(data); Node curr = head; for (int n : data) { assertEquals(n, curr.data); curr = curr.next; } assertNull(curr); } @Test void testDataToString() { int[] data = { 5, 17, 11, 10 }; Node head = NodeDriver.buildChain(data); assertEquals("5 17 11 10", NodeDriver.dataToString(head)); data = new int[] { -9 }; head = NodeDriver.buildChain(data); assertEquals("-9", NodeDriver.dataToString(head)); } @Test void testSum() { int[] data = { 5, 17, 11, 10 }; Node head = NodeDriver.buildChain(data); assertEquals(43, NodeDriver.sum(head)); assertEquals(38, NodeDriver.sum(head.next)); assertEquals(21, NodeDriver.sum(head.next.next)); assertEquals(10, NodeDriver.sum(head.next.next.next)); } @Test void testSwapEnds() { int[] data = { 5, 17, 11, 10 }; Node head = NodeDriver.buildChain(data); NodeDriver.swapEnds(head); assertEquals("10 17 11 5", NodeDriver.dataToString(head)); data = new int[] { 42 }; head = NodeDriver.buildChain(data); NodeDriver.swapEnds(head); assertEquals("42", NodeDriver.dataToString(head)); } @Test void testGetFrequency() { int[] data1 = { 3, 9, 2, 3, 3, 4, 5, 6, 3, 3 }; int[] data2 = { -13 }; Node head1 = NodeDriver.buildChain(data1); Node head2 = NodeDriver.buildChain(data2); assertEquals(1, NodeDriver.getFrequency(head1, 9)); assertEquals(5, NodeDriver.getFrequency(head1, 3)); assertEquals(0, NodeDriver.getFrequency(head1, 99)); assertEquals(1, NodeDriver.getFrequency(head2, -13)); assertEquals(0, NodeDriver.getFrequency(head2, 9)); } @Test void testAppend() { int[] data1 = { 5, 6, 7 }; int[] data2 = { 4, 3 }; int[] data3 = { 6 }; int[] data4 = { 15, 22, 11, 86 }; int[] data5 = { 15, 22, 11, 86 }; int[] data6 = { 6 }; Node head1 = NodeDriver.buildChain(data1); Node head2 = NodeDriver.buildChain(data2); Node head3 = NodeDriver.buildChain(data3); Node head4 = NodeDriver.buildChain(data4); Node head5 = NodeDriver.buildChain(data5); Node head6 = NodeDriver.buildChain(data6); NodeDriver.append(head1, head2); NodeDriver.append(head3, head4); NodeDriver.append(head5, head6); assertEquals("5 6 7 4 3", NodeDriver.dataToString(head1)); assertEquals("6 15 22 11 86", NodeDriver.dataToString(head3)); assertEquals("15 22 11 86 6", NodeDriver.dataToString(head5)); } @Test void testGetLast() { int[] data = { 5 }; Node head = NodeDriver.buildChain(data); assertEquals(5, NodeDriver.getLast(head)); data = new int[] { 8, 6, 7, 5, 3, 0, 9 }; head = NodeDriver.buildChain(data); assertEquals(9, NodeDriver.getLast(head)); } @Test void testRemoveLast() { int[] data = { 8, 6, 7, 5, 3, 0, 9 }; Node head = NodeDriver.buildChain(data); NodeDriver.removeLast(head); assertEquals("8 6 7 5 3 0", NodeDriver.dataToString(head)); NodeDriver.removeLast(head); assertEquals("8 6 7 5 3", NodeDriver.dataToString(head)); NodeDriver.removeLast(head); assertEquals("8 6 7 5", NodeDriver.dataToString(head)); NodeDriver.removeLast(head); assertEquals("8 6 7", NodeDriver.dataToString(head)); NodeDriver.removeLast(head); assertEquals("8 6", NodeDriver.dataToString(head)); NodeDriver.removeLast(head); assertEquals("8", NodeDriver.dataToString(head)); } @Test void testRemoveFirst() { int[] data = { 8, 6, 7, 5, 3, 0, 9 }; Node head = NodeDriver.buildChain(data); head = NodeDriver.removeFirst(head); assertEquals("6 7 5 3 0 9", NodeDriver.dataToString(head)); head = NodeDriver.removeFirst(head); assertEquals("7 5 3 0 9", NodeDriver.dataToString(head)); head = NodeDriver.removeFirst(head); assertEquals("5 3 0 9", NodeDriver.dataToString(head)); head = NodeDriver.removeFirst(head); assertEquals("3 0 9", NodeDriver.dataToString(head)); head = NodeDriver.removeFirst(head); assertEquals("0 9", NodeDriver.dataToString(head)); head = NodeDriver.removeFirst(head); assertEquals("9", NodeDriver.dataToString(head)); } @Test void testSumAfter10() { int[] data = { 8, 6, 7, 5, 3, 0, 9 }; Node head = NodeDriver.buildChain(data); assertEquals(0, NodeDriver.sumAfter10(head)); data = new int[] { 10 }; head = NodeDriver.buildChain(data); assertEquals(0, NodeDriver.sumAfter10(head)); data = new int[] { 1, 10, 2, 10, 3, 10 }; head = NodeDriver.buildChain(data); assertEquals(5, NodeDriver.sumAfter10(head)); data = new int[] { 10, 2, 10, 3, 10, 4 }; head = NodeDriver.buildChain(data); assertEquals(9, NodeDriver.sumAfter10(head)); data = new int[] { 10, 10, 10, 10 }; head = NodeDriver.buildChain(data); assertEquals(30, NodeDriver.sumAfter10(head)); } @Test void testFoundPair() { int[] data = { 8, 6, 7, 5, 3, 0, 9 }; Node head = NodeDriver.buildChain(data); assertFalse(NodeDriver.foundPair(head)); data = new int[] { 8, 6, 7, 5, 0, 0, 9 }; head = NodeDriver.buildChain(data); assertTrue(NodeDriver.foundPair(head)); data = new int[] { 8, 6 }; head = NodeDriver.buildChain(data); assertFalse(NodeDriver.foundPair(head)); data = new int[] { 6, 6 }; head = NodeDriver.buildChain(data); assertTrue(NodeDriver.foundPair(head)); data = new int[] { 8, 6, 7, 5, 3, 0, 9, 9 }; head = NodeDriver.buildChain(data); assertTrue(NodeDriver.foundPair(head)); data = new int[] { 18 }; head = NodeDriver.buildChain(data); assertFalse(NodeDriver.foundPair(head)); } @Test void testRemoveFirstOccurrence() { int[] data = { 8, 6, 7, 5, 3, 0, 9, 7, 99 }; Node head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 7); assertEquals("8 6 5 3 0 9 7 99", NodeDriver.dataToString(head)); head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 4); assertEquals("8 6 7 5 3 0 9 7 99", NodeDriver.dataToString(head)); head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 8); assertEquals("6 7 5 3 0 9 7 99", NodeDriver.dataToString(head)); head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 99); assertEquals("8 6 7 5 3 0 9 7", NodeDriver.dataToString(head)); data = new int[] { 7, 11 }; head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 7); assertEquals("11", NodeDriver.dataToString(head)); head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 11); assertEquals("7", NodeDriver.dataToString(head)); head = NodeDriver.buildChain(data); head = NodeDriver.removeFirstOccurrence(head, 8); assertEquals("7 11", NodeDriver.dataToString(head)); } }
NodeDriver.Java
public class NodeDriver { public static void main(String[] args) { // Do testing of your own here. It's still a reliable way // to test as you go. But once you think a method is // complete, check the JUnit tester to see if it passes. } /* * Creates a node from the specified non-empty array of * data, where the head node will contain the data at index 0. * Returns the head of the chain of nodes */ public static Node buildChain(int[] nums) { return null; } /* * Returns a String containing all the data in a * chain of nodes starting at the specified node. * The data is space-separated, with no leading or * trailing spaces. */ public static String dataToString(Node start) { return null; } /* * Returns the sum of the data in a non-empty chain of nodes * beginning at the specified node. */ public static int sum(Node start) { return -1; } /* * Modifies a non-empty chain of nodes by swapping the data * in the first and last nodes. This does NOT rewire nodes * but just changes the data. */ public static void swapEnds(Node start) { } /* * Returns the number of Nodes that the key appears * as data in the chain of nodes beginning at the specified * start node. */ public static int getFrequency(Node start, int key) { return -1; } /* * Appends the second chain of linked nodes to the end * of the first chain of linked nodes. For example, * if first were: 3, 4, 5, and second were 8, 4, 9, * then at the end of this method, first will be 3, 4, 5, 8, 4, 9 * Assume each chain contains at least one node. */ public static void append(Node first, Node second) { } /* * Gets the data in the last node of a chain of nodes */ public static int getLast(Node start) { return -1; } /* * Removes the last node of a chain of nodes. Assume * that the chain contains at least 2 nodes. */ public static void removeLast(Node start) { } /* * Removes the first node of a non-empty chain of nodes. * NOTE: this will change the object that start refers to, * but start is just a parameter variable, it is necessary to * RETURN the reference to the new starting node. * Generally speaking when we are writing code that changes * what the starting node points to, we will need to treat * that in a special way. */ public static Node removeFirst(Node start) { return null; } /* * Given a non-empty chain of nodes, compute the sum * of all values that appear immediately after a 10. * For example: * if the chain were 10, 7, 5, 10, 8, 10 then return 15 (7 + 8) * if the chain were 10, 10, 10, 10 then return 30 (10 + 10 + 10) */ public static int sumAfter10(Node start) { return -1; } /* * Given a non-empty chain of nodes, return true * if the chain contains two side-by-side nodes that have * the same value. * if the chain were 10, 7, 5, 10, 8, 10 then return false * if the chain were 1, 7, 8, 8 then return true * if the chain were 15 then return false */ public static boolean foundPair(Node start) { return false; } /* * Removes the node containing the first occurrence of the specified key, * if it exists. Otherwise, makes no changes. The method returns the head * of the resulting chain of nodes. Assume the chain has at least * two nodes. * NOTE: this method could end up removing the head node. That's why * it can't be a void method. Any method that changes what the head node * points to needs to be treated in a special way. */ public static Node removeFirstOccurrence(Node start, int key) { return null; } }
8.5.7
Notes about the Node class: A typical Node class has two instance variables: one to hold the data, and one to hold a reference to the next Node object. For this lab, the data will be an int. We will give "package access" to our Node instance variables. This makes it easy to access those variables from another class. We will write one constructor that takes an int as its parameter, and then creates a node that "Wraps" around that int. Notes about NodeDriver class: This is where you will be writing all your methods. It is also the only class you will be turning in. Use the main() method to do basic testing when you write each method create and link some nodes together, and then send the head node to the method. Use JUnit to do thorough testing. We will write buildChain() and dataToString(first, because it will give us an easy way to work with larger chains of nodes. Notes about NodeDriver Test (JUnit) class: This is a JUnit 5 test class. When you add it to a project, you will get multiple errors. This is normal. Instructions to fix this are below. If you run your JUnit tests, for each test you may see: - green (the test passed) - red (an exception was thrown) - blue (an assert statement failed) - or a little triangle indicating that the test is still running. If a test is running too long, it's probably an infinite loop. Stop it by clicking the red stop buttons. Assignment: 1. Start a new Eclipse project called lab2 - Linked Nodes 2. Create a new Node class where the data is an int, and the 2 instance variables have package level access. Include a constructor with an int parameter. (We will write this together.) 3. Download NodeDriver.java and Node Driver Tester.java and add these to your project. 4. You will need to fix the errors caused by adding the JUnit tester to your class. You simply need to update your project to include the necessary JUnit libraries. Here's how. - Open the Node Driver Test class in Eclipse. - On the top line of code on line 1, you will see org.junit underlined: - Put your mouse over the underlined org.junit. Choose the suggested fix: "Fix project setup.." to add the JUnit 5 library to your build path. 5. We will write a few of the methods and some test code together. The rest will be up to you. 6. For this lab, once your code is able to pass all JUnit tests, you are finished. Notes about the Node class: A typical Node class has two instance variables: one to hold the data, and one to hold a reference to the next Node object. For this lab, the data will be an int. We will give "package access" to our Node instance variables. This makes it easy to access those variables from another class. We will write one constructor that takes an int as its parameter, and then creates a node that "Wraps" around that int. Notes about NodeDriver class: This is where you will be writing all your methods. It is also the only class you will be turning in. Use the main() method to do basic testing when you write each method create and link some nodes together, and then send the head node to the method. Use JUnit to do thorough testing. We will write buildChain() and dataToString(first, because it will give us an easy way to work with larger chains of nodes. Notes about NodeDriver Test (JUnit) class: This is a JUnit 5 test class. When you add it to a project, you will get multiple errors. This is normal. Instructions to fix this are below. If you run your JUnit tests, for each test you may see: - green (the test passed) - red (an exception was thrown) - blue (an assert statement failed) - or a little triangle indicating that the test is still running. If a test is running too long, it's probably an infinite loop. Stop it by clicking the red stop buttons. Assignment: 1. Start a new Eclipse project called lab2 - Linked Nodes 2. Create a new Node class where the data is an int, and the 2 instance variables have package level access. Include a constructor with an int parameter. (We will write this together.) 3. Download NodeDriver.java and Node Driver Tester.java and add these to your project. 4. You will need to fix the errors caused by adding the JUnit tester to your class. You simply need to update your project to include the necessary JUnit libraries. Here's how. - Open the Node Driver Test class in Eclipse. - On the top line of code on line 1, you will see org.junit underlined: - Put your mouse over the underlined org.junit. Choose the suggested fix: "Fix project setup.." to add the JUnit 5 library to your build path. 5. We will write a few of the methods and some test code together. The rest will be up to you. 6. For this lab, once your code is able to pass all JUnit tests, you are finished Step by Step Solution
There are 3 Steps involved in it
Step: 1
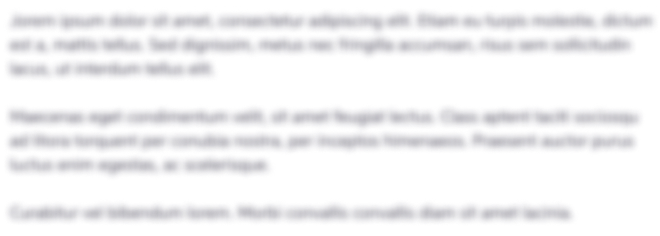
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started