Question
Note: Need help with the public boolean addAll(CiscList
Note: Need help with the public boolean addAll(CiscList extends E> c)
package edu.ust.cisc; import java.util.Iterator; public abstract class CiscAbstractListimplements CiscList { /** * Returns the number of elements in this list. * * @return the number of elements in this list */ @Override public int size() { int size = 0; Iterator itr = iterator(); while(itr.hasNext()) { itr.next(); size++; } return size; } /** * Returns {@code true} if this list contains no elements. * * @return {@code true} if this list contains no elements */ @Override public boolean isEmpty() { return size() == 0; } /** * Returns {@code true} if this list contains the specified element (compared using the {@code equals} method). * * @param o element whose presence in this list is to be tested * @return {@code true} if this list contains the specified element * @throws NullPointerException if the specified element is null */ @Override public boolean contains(Object o) { return indexOf(o) != -1; } /** * Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not * contain the element (compared using the {@code equals} method). * * @param o element to search for * @return the index of the first occurrence of the specified element in this list, or -1 if this list does not * contain the element * @throws NullPointerException if the specified element is null */ @Override public int indexOf(Object o) { int index = 0; if (o == null) { for (E e : this) { if (e == null) { return index; } index++; } } else { for (E e : this) { if (o.equals(e)) { return index; } index++; } } return -1; } /** * Removes all of the elements from this list. The list will be empty after this call returns. */ @Override public abstract void clear(); /** * Returns the element at the specified position in this list. * * @param index index of the element to return * @return the element at the specified position in this list * @throws IndexOutOfBoundsException if the index is out of range */ @Override public E get(int index) { if (index < 0 || index >= size()) { throw new IndexOutOfBoundsException("Index out of bounds: " + index); } int i = 0; for (E e : this) { if (i == index) { return e; } i++; } return null; } /** * Appends the specified element to the end of this list. * * Lists may place the specified element at arbitrary locations if desired. In particular, an ordered list will * insert the specified element at its sorted location. List classes should clearly specify in their documentation * how elements will be added to the list if different from the default behavior (end of this list). * * @param e element to be appended to this list * @return {@code true} * @throws NullPointerException if the specified element is null */ @Override public abstract boolean add(E e); /** * Replaces the element at the specified position in this list with the specified element (optional operation). * * @param index index of the element to replace * @param element element to be stored at the specified position * @return the element previously at the specified position * @throws UnsupportedOperationException if the {@code set} operation is not supported by this list * @throws NullPointerException if the specified element is null * @throws IndexOutOfBoundsException if the index is out of range */ @Override public abstract E set(int index, E element); /** * Returns an array containing all of the elements in this list in proper sequence (from first to last element). * *
The returned array will be "safe" in that no references to it are maintained by this list. (In other words, * this method must allocate a new array even if this list is backed by an array). The caller is thus free to modify * the returned array. * * @return an array containing all of the elements in this list in proper sequence */ @Override public Object[] toArray() { Object[] array = new Object[size()]; Iterator
itr = iterator(); int i = 0; while (itr.hasNext()) { array[i++] = itr.next(); } return array; } /** * Removes the first occurrence of the specified element from this list, if it is present. If this list does not * contain the element, it is unchanged. Returns {@code true} if this list contained the specified element. * * @param o element to be removed from this list, if present * @return {@code true} if this list contained the specified element * @throws NullPointerException if the specified element is null */ @Override public abstract boolean remove(Object o); /** * Removes the element at the specified position in this list. Shifts any subsequent elements to the left * (subtracts one from their indices). Returns the element that was removed from the list. * * @param index the index of the element to be removed * @return the element previously at the specified position * @throws IndexOutOfBoundsException if the index is out of range */ @Override public abstract E remove(int index); /** * Inserts the specified element at the specified position in this list (optional operation). Shifts the element * currently at that position (if any) and any subsequent elements to the right (adds one to their indices). * * @param index index at which the specified element is to be inserted * @param element element to be inserted * @throws UnsupportedOperationException if the {@code add} operation is not supported by this list * @throws NullPointerException if the specified element is null * @throws IndexOutOfBoundsException if the index is out of range */ @Override public abstract void add(int index, E element); /** * Appends all elements in the specified list to the end of this list, in the order that they are returned by the * specified list's iterator (optional operation). * * @param c list containing elements to be added to this list * @return {@code true} if this list changed as a result of the call * @throws UnsupportedOperationException if the {@code addAll} operation is not supported by this list * @throws NullPointerException if the specified list is null */ @Override public boolean addAll(CiscList extends E> c){ return false; } /** * Returns an iterator over the elements in this list in proper sequence. * * @return an iterator over the elements in this list in proper sequence */ @Override public abstract Iterator iterator(); /** * Returns a string representation of this list. This string should consist of a comma separated list of values * contained in this list, in order, surrounded by square brackets (examples: [3, 6, 7] and []). * * @return a string representation of this list */ @Override public String toString() { Iterator itr = iterator(); if (!itr.hasNext()) { return "[]"; } StringBuilder sb = new StringBuilder(); sb.append("["); for (;;) { E e = itr.next(); sb.append(e == this ? "(this Collection)" : e); if (!itr.hasNext()) { return sb.append("]").toString(); } sb.append(", "); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
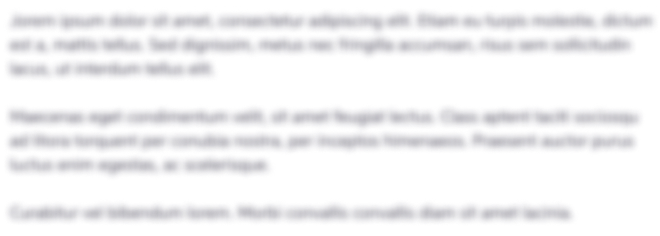
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started