Question
Note: optional must be used to solve this question. 1. Complete the exceptionThrowingList class by doing the // TODOS i. There's only one method to
Note: optional must be used to solve this question.
For example: 11 12 13 *
- you can only create an empty or full one, and only with the included simple factories
- you can never add anything to it once it's created
- you can ask it to findBiggest(), which just means to either throw an exception (if the list is empty), or return the last element in the list 15 18 19 * @author jpratt * @param
the type of thing in the list 29 21 22 public final class ExceptionThrowingList { 23 24 private List thingsInList; private int size; 25 26 27 28 * This is a simple factory to return an empty Exception Throwinglist. It's a static method, so * remember to call it... how? 29 30 31 * @param the type of thing you want to put in this ExceptionThrowinglist * @return an empty ExceptionThrowingList 32 */ 34 public static ExceptionThrowingList emptyList() { 35 return new ExceptionThrowingList (); 36 37 38 } * This is a simple factory to return a "full" ExceptionThrowingList; you give it the stuff you * want to fill it with. It's a static method, so remember to call it...how? 41 42 43 44 @param the type of thing you want to put in this ExceptionThrowinglist * @param filledWithThese the things you want to put in this ExceptionThrowinglist * @return the "full" ExceptionThrowingList 45 * 47 48 public static Exception throwingList fulltist(List filledWithThese) { ExceptionThrowingList theNewList - emptyList(); theNewlist.thingsInList.addAll(filledWithThese); 49 59 51 the Newlist.size += filledWithThese.size(); return theNewList; 52 53 } 54 55 // TODO: complete the findBiggest method 56 57 * Returns the "biggest" element in this ExceptionThrowingList; we'll assume that's the element in * the last index of the underlying thingsInList. 58 59 * 60 * If someone tries to use this method when there's nothing in things Inlist, this method should * throw a NoSuchElementException with the message "You can't findBiggest when it's empty." 61 62 63 64 65 60 * @return the element in the last position of things Inlist * @throws NoSuchElementException if there's nothing in this ExceptionThrowingList */ public I findBiggest() { 67 68 } 69 79 71 * This constructor is private so that it forces clients of this class to use the two simple * factories (emptyList and fulllist) to make lists. 72 74 75 76 private ExceptionThrowingList() { this thingsInList = new ArrayList(); this.size = 0; } } 77 78 1 package main; import java.util.ArrayList; import java.util.List; 4 5 6 /** 7 * This class represents a list of T, but is kind of silly. 8 *
For example: 9 10 11 12 13 *
- you can only create an empty or full one, and only with the included simple factories
- you can never add anything to it once it's created
- you can ask it to findBiggest(), which just means to either to return an empty Optional (if the list is empty), or return an Optional holding the last element in the list *
the type of thing in the list 19 21 public final class OptionalReturningList { 22 23 private List thingsInList; private int size; 24 25 27 * This is a simple factory to return an empty OptionalReturninglist. It's a static method, so * remember to call it... how? 28 29 30 31 @param the type of thing you want to put in this optionalReturningList * @return an empty Optional ReturningList 32 */ 30 public static OptionalReturningList emptyList() { 34 return new OptionalReturningList (); 35 36 37 } 38 /** * This is a simple factory to return a "full" OptionalReturningList; you give it the stuff you * want to fill it with. It's a static method, so remember to call it... how? 40 41 42 43 44 45 * @param the type of thing you want to put in this Optional ReturningList @param filledWithThese the things you want to put in this OptionalReturningList * @return the "full" OptionalReturningList */ public static OptionalReturningList fulllist(List filledwithThese) { OptionalReturningList theNewContainer = emptyList(); theNewContainer things InList.addAll(filledWithThese); 46 47 43 49 50 theNewContainer. size += filledWithThese.size(); return theNewContainer; 51 52 } 53 54 // TODO: complete the findBiggest method 55 56 * Returns the "biggest" element in this OptionalReturningList; we'll assume that's the element in * the last index of the underlying thingsInList. 57 2 * 58 59 * @return an Optional that either holds the element in the last position of things Inlist, or is empty */ public Optional findBiggest() { 61 62 63 64 ) 65 66 67 68 69 /** * This constructor is private so that it forces clients of this class to use the two simple * factories (emptyList and fulllist) to make lists. */ private OptionalReturningList() { this. thingsInList = new ArrayList(); this.size = 0; 70 71 72 73 74 } 1. Complete the exceptionThrowingList class by doing the // TODOS i. There's only one method to complete - findBiggest. Read its docs and tests to get this done correctly. ii. if the ExceptionThrowingList_Find_Biggest_Tests tests all pass, you can consider your ExceptionThrowinglist done. 2. Now do the same kind of thing for OptionalReturningList. 3. Complete the App.java run method so that when you run Main.java , the program behaves as expected by the MainTests. Example Runs Here is an example run of a working solution when the user just hits Enter right away: Enter in a list of zero or more ints, separated by whitespace: Didn't find a darn thing in the Optional ReturnList. Sad now. Didn't find a darn thing in the Exception ThrowingList and got this dang exception: You can't findBiggest when it's empty. Here is an example run of a working solution (user input in bold): Enter in a list of zero or more ints, separated by whitespace: 19 54 383 Found me the biggest in the Optional ReturnList! It's: 383 Found me the biggest in the ExceptionThrowingList! It's: 383 package main; 1 2 3 public class Main { 4 5 6 public static void main(String[] args) { App app = new App(); app.run(); } 7 DO } 1 package main; 2 3 4 import java.util.ArrayList; import java.util.List; import java.util.Scanner; 5 6 7 public class App { 8 9 private Scanner kbd = new Scanner(System.in); 10 11 public void run() { 12 13 // You need to complete the handleEmptyCase and handleFullCase methods - everything // else here has been done for ya! 14 15 16 17 System.out.print("Enter in a list of zero or more ints, separated by whitespace: "); String input = kbd.nextLine(); 18 19 System.out.println(); 20 21 22 23 if (input.isBlank()) { handleEmptyCase(); } else { handleFullCase(listOfNumsFromString(input)); } 24 25 26 } 27 28 29 30 31 32 // TODO: complete handleEmpty Case. The plan here: // // - create an empty OptionalReturninglist and empty ExceptionThrowinglist // - they should both be typed to Integer - you might wonder how to make them... are there any methods that do that for you? // - call findBiggest on each of the lists; because they're empty, they'll react in different ways (how?) // - output the necessary messages to System.out based on what you got back from each list hint: you can get the message from an exception with the getLocalizedMessage method 33 34 35 36 private void handleEmptyCase() { 37 38 39 } 40 41 42 43 44 45 46 // TODO: complete handleFullCase. The plan here: // create a full OptionalReturninglist and full ExceptionThrowingList // they should both be typed to Integer // you might wonder how to make them...are there any methods that do that for you? 11 call findBiggest on each of the lists; because they're not empty, they'll 1/ both return something that you can use (what?) output the necessary messages to System.out based on what you get back private void handleFullCase(List nums) { // } 48 49 50 51 52 53 54 55 // Turns the whitespace-delimited buncha ints from the user into a purdy // List of Integers through the magic of split, regex, and just a *sprinkle* // of pixie dust. private List listOfNumsFromString(String listOfNumsAsText) { List nums new ArrayList(); 56 57 58 59 60 61 62 63 String[] numsAs Text listOfNumsAsText.split("\\s+"); for (String numAsText : numsAsText) { int num = Integer.parseInt(numAsText); nums.add(num); } 64 65 return nums; 66 } 67 1 package main; 2 3 import java.util.ArrayList; import java.util.List; 4 5 7 * This class represents a list of T, but is kind of silly. 13 * For example: 11 12 13 *
- you can only create an empty or full one, and only with the included simple factories
- you can never add anything to it once it's created
- you can ask it to findBiggest(), which just means to either throw an exception (if the list is empty), or return the last element in the list 15 18 19 * @author jpratt * @param
the type of thing in the list 29 21 22 public final class ExceptionThrowingList { 23 24 private List thingsInList; private int size; 25 26 27 28 * This is a simple factory to return an empty Exception Throwinglist. It's a static method, so * remember to call it... how? 29 30 31 * @param the type of thing you want to put in this ExceptionThrowinglist * @return an empty ExceptionThrowingList 32 */ 34 public static ExceptionThrowingList emptyList() { 35 return new ExceptionThrowingList (); 36 37 38 } * This is a simple factory to return a "full" ExceptionThrowingList; you give it the stuff you * want to fill it with. It's a static method, so remember to call it...how? 41 42 43 44 @param the type of thing you want to put in this ExceptionThrowinglist * @param filledWithThese the things you want to put in this ExceptionThrowinglist * @return the "full" ExceptionThrowingList 45 * 47 48 public static Exception throwingList fulltist(List filledWithThese) { ExceptionThrowingList theNewList - emptyList(); theNewlist.thingsInList.addAll(filledWithThese); 49 59 51 the Newlist.size += filledWithThese.size(); return theNewList; 52 53 } 54 55 // TODO: complete the findBiggest method 56 57 * Returns the "biggest" element in this ExceptionThrowingList; we'll assume that's the element in * the last index of the underlying thingsInList. 58 59 * 60 * If someone tries to use this method when there's nothing in things Inlist, this method should * throw a NoSuchElementException with the message "You can't findBiggest when it's empty." 61 62 63 64 65 60 * @return the element in the last position of things Inlist * @throws NoSuchElementException if there's nothing in this ExceptionThrowingList */ public I findBiggest() { 67 68 } 69 79 71 * This constructor is private so that it forces clients of this class to use the two simple * factories (emptyList and fulllist) to make lists. 72 74 75 76 private ExceptionThrowingList() { this thingsInList = new ArrayList(); this.size = 0; } } 77 78 1 package main; import java.util.ArrayList; import java.util.List; 4 5 6 /** 7 * This class represents a list of T, but is kind of silly. 8 *
For example: 9 10 11 12 13 *
- you can only create an empty or full one, and only with the included simple factories
- you can never add anything to it once it's created
- you can ask it to findBiggest(), which just means to either to return an empty Optional (if the list is empty), or return an Optional holding the last element in the list *
the type of thing in the list 19 21 public final class OptionalReturningList { 22 23 private List thingsInList; private int size; 24 25 27 * This is a simple factory to return an empty OptionalReturninglist. It's a static method, so * remember to call it... how? 28 29 30 31 @param the type of thing you want to put in this optionalReturningList * @return an empty Optional ReturningList 32 */ 30 public static OptionalReturningList emptyList() { 34 return new OptionalReturningList (); 35 36 37 } 38 /** * This is a simple factory to return a "full" OptionalReturningList; you give it the stuff you * want to fill it with. It's a static method, so remember to call it... how? 40 41 42 43 44 45 * @param the type of thing you want to put in this Optional ReturningList @param filledWithThese the things you want to put in this OptionalReturningList * @return the "full" OptionalReturningList */ public static OptionalReturningList fulllist(List filledwithThese) { OptionalReturningList theNewContainer = emptyList(); theNewContainer things InList.addAll(filledWithThese); 46 47 43 49 50 theNewContainer. size += filledWithThese.size(); return theNewContainer; 51 52 } 53 54 // TODO: complete the findBiggest method 55 56 * Returns the "biggest" element in this OptionalReturningList; we'll assume that's the element in * the last index of the underlying thingsInList. 57 2 * 58 59 * @return an Optional that either holds the element in the last position of things Inlist, or is empty */ public Optional findBiggest() { 61 62 63 64 ) 65 66 67 68 69 /** * This constructor is private so that it forces clients of this class to use the two simple * factories (emptyList and fulllist) to make lists. */ private OptionalReturningList() { this. thingsInList = new ArrayList(); this.size = 0; 70 71 72 73 74 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
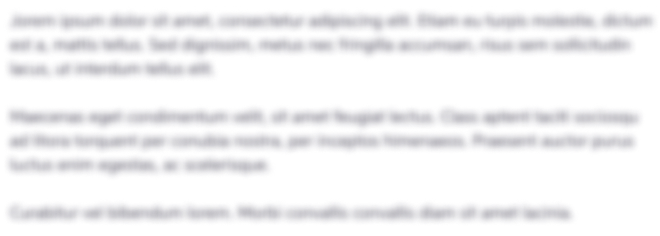
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started