Question
NOTE: OUTPUT HAS TO LOOK SPECIFIED WAY BELOW. MAKE SURE FIREBALL AND POTION WORK. Introduction Focuses on combat between a player and series of monsters.
NOTE: OUTPUT HAS TO LOOK SPECIFIED WAY BELOW. MAKE SURE FIREBALL AND POTION WORK.
Introduction
Focuses on combat between a player and series of monsters. Inheritance will be required to make all the monsters different without changing how battles work.
In this game a hero will encounter and fight a series of enemies.
NOTE: The description below is a bare minimum requirement specification. As your final assignment, you have a lot of implementation freedom and hope you have fun thinking about things like pass/return by reference, const parameters/functions, data types, enumeratrion, classes, encapsilation, overloading, inheritance, dynamic memory, etc.. We want to get used to good design and implementation decisions early and often!
Part 1: The Enemy
Make an enemy class. This will be the parent class of every monster our hero has to fight. It will supply the minimum functions that are needed for a monster to exist in the game.
This is an abstract class, all your methods (with the exception of the constructor) should be virtual and set =0.
A good starting point for you enemy class is as follows:
A way to check how much health the enemy has.
A way to damage the enemy.
An attack method for the enemy to damage another enemy.
A method that prints to the console information about the enemy so the player knows who they are fighting.
The hero will also be a kind of enemy. (It is the enemy to the monsters.)
Part 2: Make Monsters
Create 5 types of monsters, each derived from your Enemy class/interface. All your monsters must meet the following requirements:
Can be defeated.
Can attack an enemy and take damage from and enemy.
Additionally, at least 1 of your monsters MUST have multiple attacks that it decides between at random.
Part 3: Make a Hero
The hero is just a kind of enemy!
Create a hero class that implements the enemy interface. You will need to get input for the user to decide what attack to do.
A hero has the following properties:
A hero has a name, chosen by the player.
A hero has health bar
A hero has 6 potions that each heals some points of damage.
A hero has 10 fireballs, each do damage. (More damage then the sword.)
A hero has a sword that does damage.
A hero has a shield. When the shield is up the hero can't attack but attacks on them only do half damage.
When it is the hero's attack method is called, print out the hero's status and ask for input on which attack to use. Then do what is requested by the player.
The player may quit the game at any time by saying their action is "exit". You will need to use an exception to break out of the code and exit the program when this happens.
Part 4: The Game
Implement a main program that plays the game. The game should go as follows:
Ask for the Hero's name
Ask the user for how many Enemies there will be (you may assume they will enter a positive integer)
Create the number of enemies requested in (2) whose types are randomly selected from your pool of 5 potential enemy types.
For each enemy (you will fight them one at a time)
Print out what monster is attacking
Fight until the enemy or hero is dead
If the monster died, print that out.
Either declare the hero victorious or dead.
A single round of combat should go as follows:
Hero attacks
If the monster is alive, it attacks
Reminder: The player can type exit at any time to throw an exception. If this happens, you should tell the player "Thanks for Playing" and exit the program.
Here is an example of how the game could look with 2 monsters.
Welcome to Adventure Battle! What is the name of your hero? Cloud How many enemies do you want Cloud to battle? 2 You have encountered a Bat! Cloud: 100/100 health Remaining: 10 Fireballs, 6 Potions Enter Command: sword shield fireball potion exit shield Hide Behind Shield! Bat bites you! Cloud: 98/100 health Remaining: 10 Fireballs, 6 Potions Enter Command: sword shield fireball potion exit sword Sword Slash Attack! Bat bites you! Cloud: 93/100 health Remaining: 10 Fireballs, 6 Potions Enter Command: sword shield fireball potion exit sword Sword Slash Attack! Enemy is defeated! You have encountered a Wolf! Cloud: 93/100 health Remaining: 10 Fireballs, 6 Potions Enter Command: sword shield fireball potion exit potion You drank a potion. Wolf scratches you! Cloud: 85/100 health Remaining: 10 Fireballs, 5 Potions Enter Command: sword shield fireball potion exit fireball Fireball Attack Successful! Wolf scratches you! Cloud: 70/100 health Remaining: 9 Fireballs, 5 Potions Enter Command: sword shield fireball potion exit fireball Fireball Attack Successful! Enemy is defeated! You have defeated all enemies and saved the world. Good Job.
Finishing Up
All five of your monsters (h and cpp files) with at least 1 monster that has random attacks.
The hero header and cpp file.
The main file used to implement the game.
Monster.h
#ifndef _BATTLE_MONSTER_
#define _BATTLE_MONSTER_
#include
using namespace std;
//This class defines a generic monster
//It doesn't actually DO anything.
//It just gives you a template for how a monster works.
//We can make any number of monsters and have them fight
//they just all need to INHERIT from this one so that work the same way
//Since this class is not intended to be used
//none of the methods do anything
//The virtual command means we want the children to override these.
//The =0 part means these function's won't even work if you tried.
//This class is impossible to use by itself.
class monster
{
public:
monster();
//Name the monster we are fighting
//The description is printed at the start to give
//additional details
virtual string getName() const=0;
virtual string getDescription() const=0;
//Basic Attack Move
//This will be the most common attack the monster makes
//You are passed a pointer to the monster you are fighting
virtual void basicAttack(monster * enemy)=0;
//Print the name of the attack used
virtual string basicName() const=0;
//Defense Move
//This move is used less frequently to
//let the monster defend itself
virtual void defenseAttack(monster * enemy)=0;
//Print out the name of the attack used
virtual string defenseName() const=0;
//Special Attack
//This move is used less frequently
//but is the most powerful move the monster has
virtual void specialAttack(monster * enemy)=0;
virtual string specialName() const=0;
//Health Management
//A monster at health <= 0 is unconscious
//This returns the current health level
virtual int getHealth() const=0;
//This function is used by the other monster to
//either do damage (positive int) or heal (negative int)
virtual void doDamage(int damage) =0;
//Reset Health for next match
virtual void resetHealth() = 0;
};
#endif
monster.cpp
#include "monster.h"
//Since we don't actually want these to be used they don't need to be useful
//Abstract Classes are another way to do this, but they will come later
monster::monster(){}
hamster.h
#ifndef _HAMSTER_
#define _HAMSTER_
#include
#include "monster.h"
using namespace std;
//This is a hamster.
//It is a very weak monster.
class hamster : public monster
{
private:
string my_name;
int my_health;
bool defense_mode;
public:
hamster(string n="No Name");
//redefine all the Methods of monster
//Note: we can make more methods if we want, but
//we need to redfine the methods of monster
//because those are the ones that will be used in the game.
string getName() const;
string getDescription() const;
void basicAttack(monster * enemy);
string basicName() const;
void defenseAttack(monster * enemy);
string defenseName() const;
void specialAttack(monster * enemy);
string specialName() const;
int getHealth() const;
void doDamage(int damage);
void resetHealth();
};
#endif
hamster.cpp
#include "hamster.h"
hamster::hamster(string n)
{
my_name = n;
my_health=20;
defense_mode=false;
}
string hamster::getName() const
{
return my_name;
}
string hamster::getDescription() const
{
return "The hamster is both fluffy and friendly.";
}
void hamster::basicAttack(monster * enemy)
{
defense_mode=false;//Can't defend and attack
enemy->doDamage(2);
}
string hamster::basicName() const
{
return "Bite";
}
void hamster::defenseAttack(monster * enemy)
{
defense_mode=true;
}
string hamster::defenseName() const
{
return "Hide";
}
void hamster::specialAttack(monster * enemy)
{
defense_mode=false;//Can't defend and attack
enemy->doDamage(3);
}
string hamster::specialName() const
{
return "Ravenous Fury";
}
int hamster::getHealth() const
{
return my_health;
}
void hamster::doDamage(int damage)
{
if(defense_mode)
{
//Defense mode cuts damage in half
my_health=my_health-(damage/2);
}else
{
my_health=my_health-damage;
}
}
void hamster::resetHealth()
{
my_health=20;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
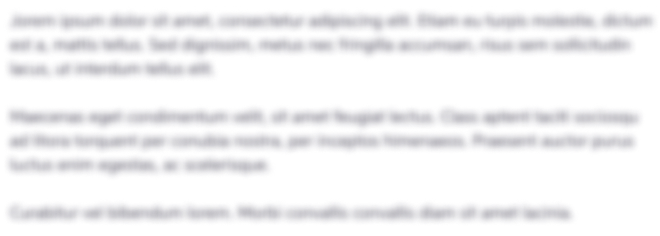
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started