Question
Note: you must follow the rules of encapsulation for all instance fields of all classes you write. You will first creat e an Ingredient class.
Note: you must follow the rules of encapsulation for all instance fields of all classes you write. You will first creat e an Ingredient class. For a chef to keep track of his recipes, he must know two qualities of each ingredient: its kind and its quantity. An ingredient's kind can be any string of characters as long as it is not just spaces, the empty String, or null. As a hint, the String class has a method which returns a string that omits leading and trailing whitespaces (try checking the API)! An ingredient's quantity can be any positive number of grams. When creating a new ingredient for a recipe, we can let chefs specify both a kind and quantity or if they specify neither, we will just assume they mean to createe an ingredient with kind "Salt" and quantity 0.2. If a chef tries to createe an ingredient with an illegal name or quantity, replace the illegal value(s) with "Salt" and 0.2, respectively. Keep in mind that chefs may need to view and change an ingredient's kind and quantity once they are created (hint: what kinds of methods allow us to accomplish this while maintaining encapsulation?). If they attempt to change either quality into an illegal value, do not change that value. You will also createe a Recipe class. A recipe is more than just a collection of ingredients! A recipe has a name, prepTime, numServings, and finally ingredients. A recipe's name can be anything except just spaces, the empty String, or null. A recipe's prep time can be any positive number of whole minutes. Its number of servings, which represents how many people this recipe can serve, should also be a positive whole number. Finally, the recipe's ingredients, a collection of Ingredient objects, must contain at least one ingredient and can contain a maximum of 10 ingredients at a time. A chef must be able to createe a recipe by giving it a name, prep time, number of servings, and an array of ingredients. However, if none of these are specified, they should be assumed to be creating a recipe with name "Lamb Sauce", prep time of 60 minutes, 8 servings, and with ingredients containing only one ingredient that is of kind "Salt" and quantity 0.2. If a chef tries to createe a recipe with any illegal values, replace those illegal value(s) with the values specified in the previous sentence. Additionally, since chefs can also view or change a recipe's qualities through specific methods, if they ever try to change any quality into an illegal value, do not change that value. Thankfully, storing your recipes inJava brings many extra benefits. Among these is the ability to determine which ingredient is most prevalent in a recipe by just calling the dominantIngredient method on it and the specific ingredient with highest quantity in this recipe should be returned. In the event of a tie, return the ingredient which was first considered most dominant. Additionally, a recipe can be scaled by a positive whole number factor to serve more people even though it will cost more ingredients and prep time. For example, if I scaleRecipe by 3 on a recipe that originally takes 15 minutes, serves 2 people, and contains ingredients Salt with quantity 0.2 grams and Lamb with quantity 4 grams, after the method call, the recipe should take 45 minutes to prep, serve 6 people, and contain 0.6 grams of Salt and 12 grams of Lamb. If a chef tries to scale a recipe by an impossible factor (see constraintsabove), do not change the recipe.
Furthermore, and most excitingly, you will also createe a Chef class. A chef has a name, specialty, and keeps a collection of recipes. The chef's name may be any String which is not-empty, not comprised solely by whitespaces, and not null. Likewise, the chef's specialty may be any String which is not-empty, not comprised solely by whitespaces, and not null. Any chef's collection of recipes will contain at least one recipe and can contain a maximum of 10 recipes at a time. When initializing a chef, a default constructor should createe a chef with name "Gordon Ramsay", specialty "Lamb Sauce", and an array of recipes which only contains the earlier described default recipe. A three-arg constructor will take in values for each of the above mentioned instance fields. In the event that any of the arguments received by this constructor are invalid, the default constructor values will be used for all instance fields. Chefs can be asked to prepare any recipe in their repertoire! Given an index of a recipe in the chef's known recipes, the cook method prints: "Bon Appetit! Using
Additionally, if the chef is asked to cook a recipe which matches their specialty, the recipe's prep time reported should be half the one listed in the recipe (it is okay for this to cause prep time to become 0). If the chef is asked to cook a recipe whose index is out of bounds of his recipes or if the index requested does not contain a recipe, this method should print: "What is this nonsense? Get out of my kitchen, I won't make that!" Building upon the chef's previous abilities, they can also be asked to prepare food for a certain number of people, or cater. The cater method causes the chef to look through their recipes and cook the first (and only the first) recipe which properly serves at least the number of people given as an argument. If the chef does not find a recipe that meets the requirements, this method should print: "My gran could do better... than me?!"
Lastly, you will create a driver Kitchen class. We highly encourage you to test your code in this driver class. Think about any edge cases that may cause your code to "break." The only firm requirement is that you make use of the main method to create at least one chef.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
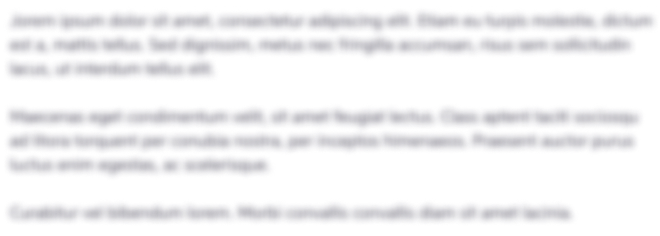
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started