Question
Now that you have your GUI operational, it is time to take the application a step further. Management would like you to write the entered
Now that you have your GUI operational, it is time to take the application a step further. Management would like you to write the entered data into a file. They intend to use an application to read this file, evaluate the entered data, and display results. These results will help management to make decisions on sales force direction and expansion. Each time the ENTER button is pressed, the entered sales representatives data will be written out to a file. A new button, EVALUATE will be added that reads in the sales representatives data file after it has been created.
Design Requirements:
You must use pseudocode to design your algorithm for the ENTER button functionality. These design artifacts (pseudocode) will be inserted into a design document to be reviewed by your classmates and submitted with the final application for the final task.
Application Requirements:
Expand your Swing application to write the entered data out to a text file. Instead of displaying the data in the jTextArea when ENTER is pressed, change this functionality so that the data is written to a file. Name the output file salesrep.txt. Each time the ENTER button is pressed, the data will be written to the file. Write the data in the following format to a text file, using white spaces as the delimiter. Include the categories (SUPPLIES, BOOKS, PAPER) in your file to label the dollar amount sold for each category. The sales district entered should be converted to upper case (NORTH, SOUTH, EAST, WEST). Independent line separators should be used in the output file. Code for the ENTER button should be well commented.
Format of your file:
salesRepID firstName lastName SUPPLIES totalAmountSuppliesSold BOOKS totalAmountBooksSold PAPER totalAmountPaperSold district contactMeans
Example output file contents:
1001 Jennifer Ward SUPPLIES 2140.20 BOOKS 5200.10 PAPER 455.23 NORTH Phone 1003 Athena Andrews SUPPLIES 5155.55 BOOKS 6300.50 PAPER 223.25 SOUTH Email
Each time the ENTER button is pressed, the application should append a new line to the file. When the application starts, the file should be opened for appending. This file is to keep a running history of the entered data.
HERE IS MY JAVA CODE:
package swinggui; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.ButtonGroup; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JRadioButton; import javax.swing.JTextArea; import javax.swing.JTextField;
//Declare Main Swing Class. Create JFrame and event listener. public class SwingGUI extends JFrame implements ActionListener { JLabel lb1,lb2,lb3,lb4,lb5,lb6; //Declare JLabel's for Text Field's and Buttons JTextField tf1,tf2,tf3,tf4,tf5,tf6,tf7;//Declare Text Field's JButton bt1,bt2;//Declare buttons for Enter and Quit JComboBox cb1;//Declare combo box drop down for North, etc. JRadioButton rb1,rb2,rb3;//Declare radio button variables for phone, etc. ButtonGroup bg;//Group up radio buttons for one section JTextArea ta;//Declare Text area for output
public SwingGUI() { //Assign values for labels, should all be string's. lb1=new JLabel("Sales Rep ID"); lb2=new JLabel("Rep First Name"); lb3=new JLabel("Rep Last Name"); lb4=new JLabel("Total Sold"); lb5=new JLabel("Sales District"); lb6=new JLabel("Contact Method"); //Create and assign all text fields's for value input and text area. ta=new JTextArea(); tf1=new JTextField(); tf2=new JTextField(); tf3=new JTextField(); tf4=new JTextField("Office Supplies"); tf5=new JTextField("Books"); tf6=new JTextField("Paper"); tf7=new JTextField(); //Assign string value array for Combo box drop down selection. String str[]={"North","South","East","West"}; cb1=new JComboBox(str); //Create button group and radio buttons for contact information. bg=new ButtonGroup(); rb1=new JRadioButton("Phone",true); rb1.setActionCommand("Phone"); rb2=new JRadioButton("Email"); rb2.setActionCommand("Email"); rb3=new JRadioButton("Visit"); rb3.setActionCommand("Visit"); //Create JButtons for quit and enter and assign listener to this constructor. bt1=new JButton("Quit"); bt2=new JButton("Enter"); bt1.addActionListener(this);//@Suppresswarning Leaking in this constructor. Doesn't work in netbeans. bt2.addActionListener(this);//@Suppresswarning Leaking in this constructor. Doesn't work in netbeans. //Add radio buttons. bg.add(rb1); bg.add(rb2); bg.add(rb3); ///Start layout as null and assign values for boundaries for Labels, Text Field, Combo Box, Radio Button, Enter/quit buttons, and text area respectively. setLayout(null); lb1.setBounds(50,50,100,20); lb2.setBounds(50,80,100,20); lb3.setBounds(50,110,100,20); lb4.setBounds(50,140,100,20); lb5.setBounds(50,170,100,20); lb6.setBounds(50,200,100,20); tf1.setBounds(200,50,100,20); tf2.setBounds(200,80,100,20); tf3.setBounds(200,110,100,20); tf4.setBounds(200,140,70,20); tf5.setBounds(270,140,70,20); tf6.setBounds(340,140,70,20); cb1.setBounds(200,170,100,20); rb1.setBounds(200,200,70,20); rb2.setBounds(270,200,70,20); rb3.setBounds(340,200,70,20); tf7.setBounds(200,230,200,20); bt1.setBounds(100,300,100,20); bt2.setBounds(250,300,100,20); ta.setBounds(450,50,200,300);
//Add declared, assigned, and instantiated values to the JFrame for use. add(lb1); add(lb2); add(lb3); add(lb4); add(lb5); add(lb6); add(tf1); add(tf2); add(tf3); add(tf4); add(tf5); add(tf6); add(tf7); add(cb1); add(rb1); add(rb2); add(rb3); add(bt1); add(bt2); add(ta); } //Set main, create GUI size and visibility. public static void main(String args[]) { SwingGUI sg=new SwingGUI(); sg.setSize(900,500); sg.setVisible(true); } //Override constructor for implementation in start of package. Action event for quit button and enter. @Override public void actionPerformed(ActionEvent e) { String str=e.getActionCommand(); //String value for Quit button assignment. //String Selection = this.bg.getSelection().getActionCommand(); May be needed for displaying button group selection in Text Area... if(str.equals("Quit")){ System.exit(0); //Exit when quit is selected. } else //When enter button is pressed preform this action, or if any other button besides quit is selected. { ta.setText("ID ="+tf1.getText()+" Name is= "+tf2.getText()+" "+tf3.getText()+" Total Supply is= Office "+tf4.getText()+" \tBooks "+tf5.getText()+" \tPaper "+tf6.getText()+" District is= "+cb1.getSelectedItem()+" Contact is= "+bg.getSelection().getActionCommand()+ " " +tf7.getText()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
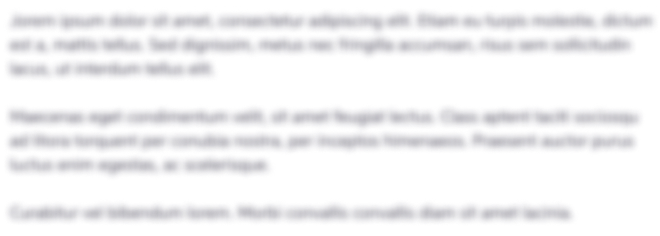
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started