Answered step by step
Verified Expert Solution
Question
1 Approved Answer
O k Student Name: Student ID: Project: Classes Type: Group Assignment Students should ONLY USE programming constructs covered in the course material. Submissions using
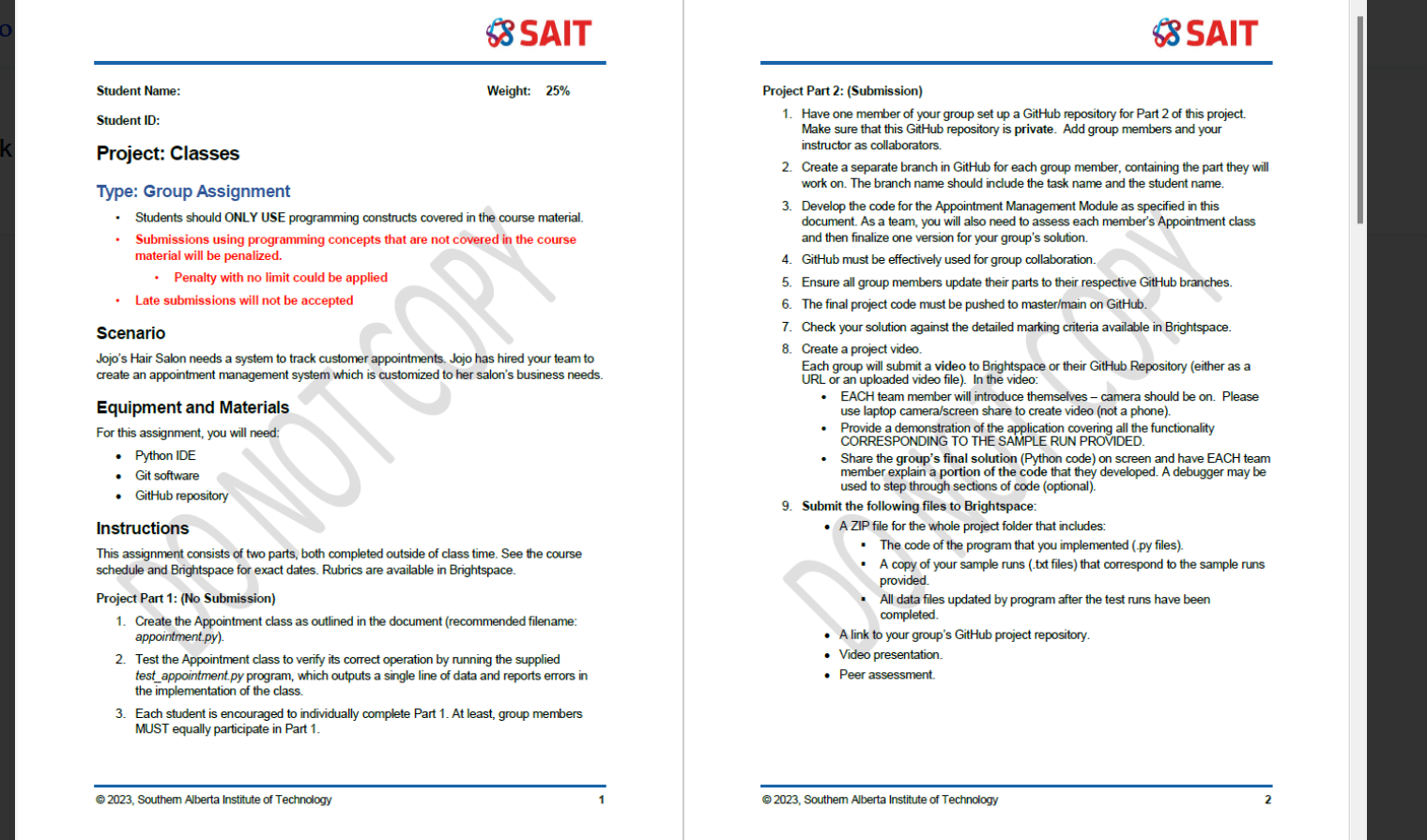
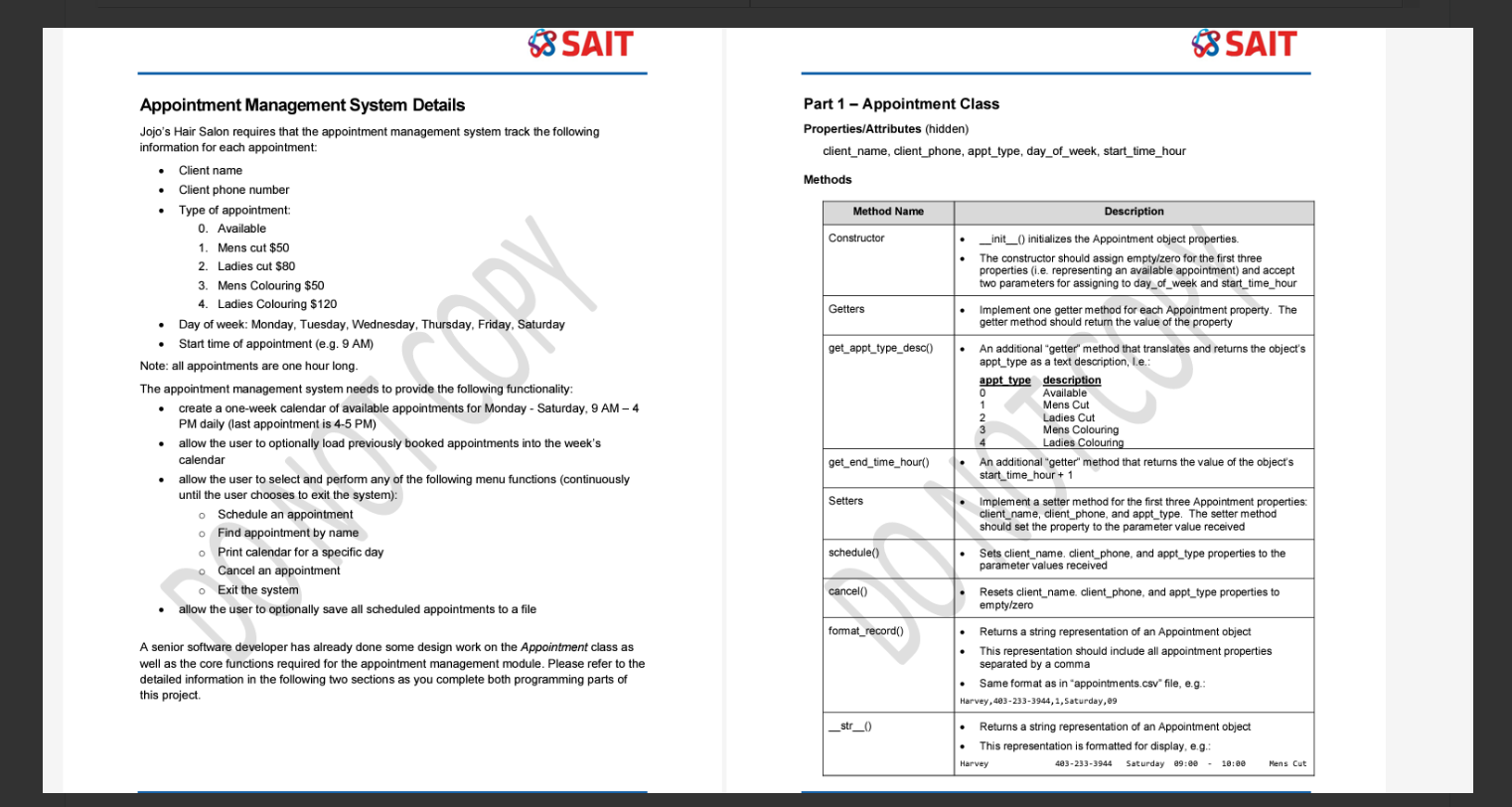
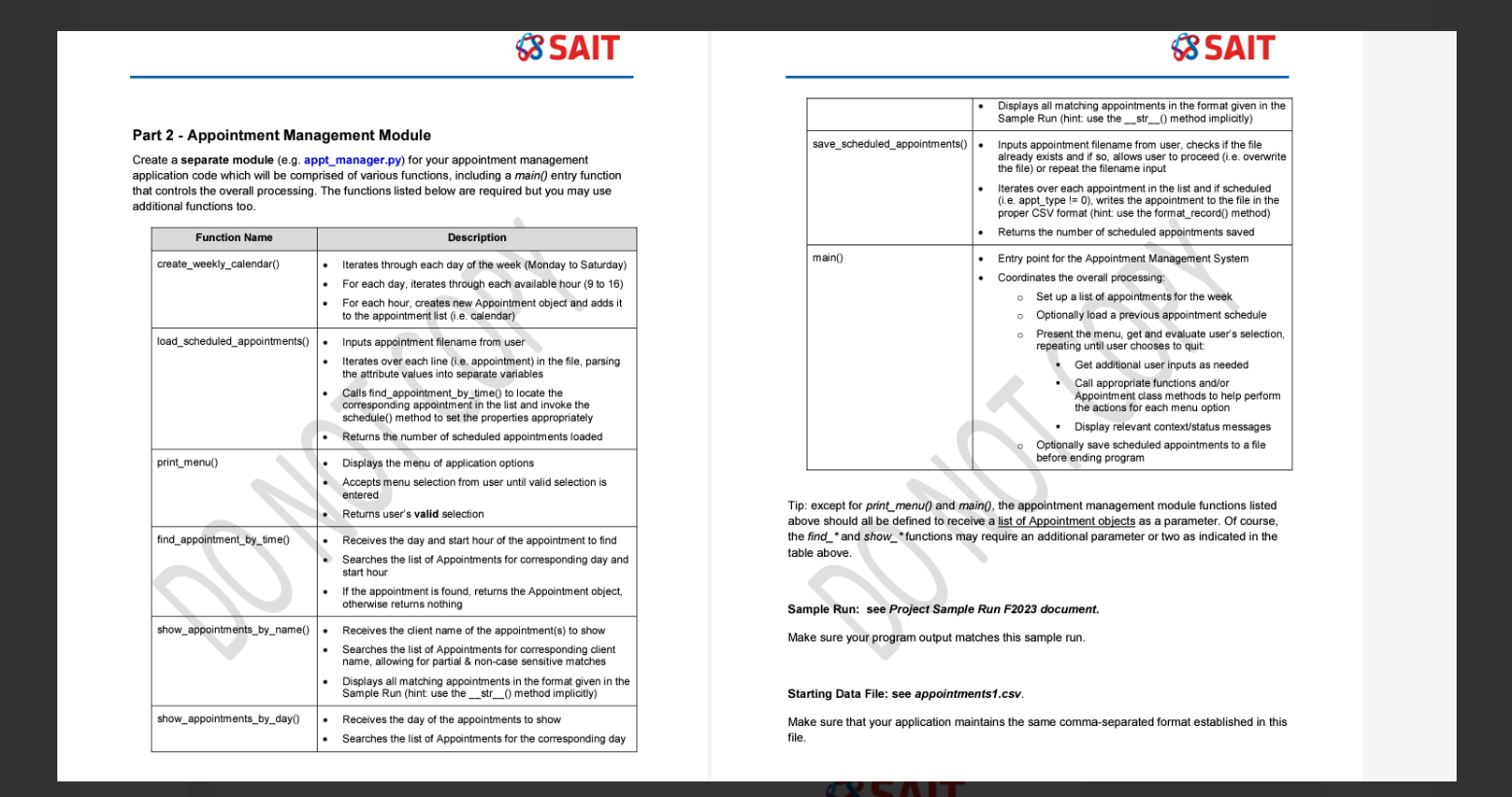
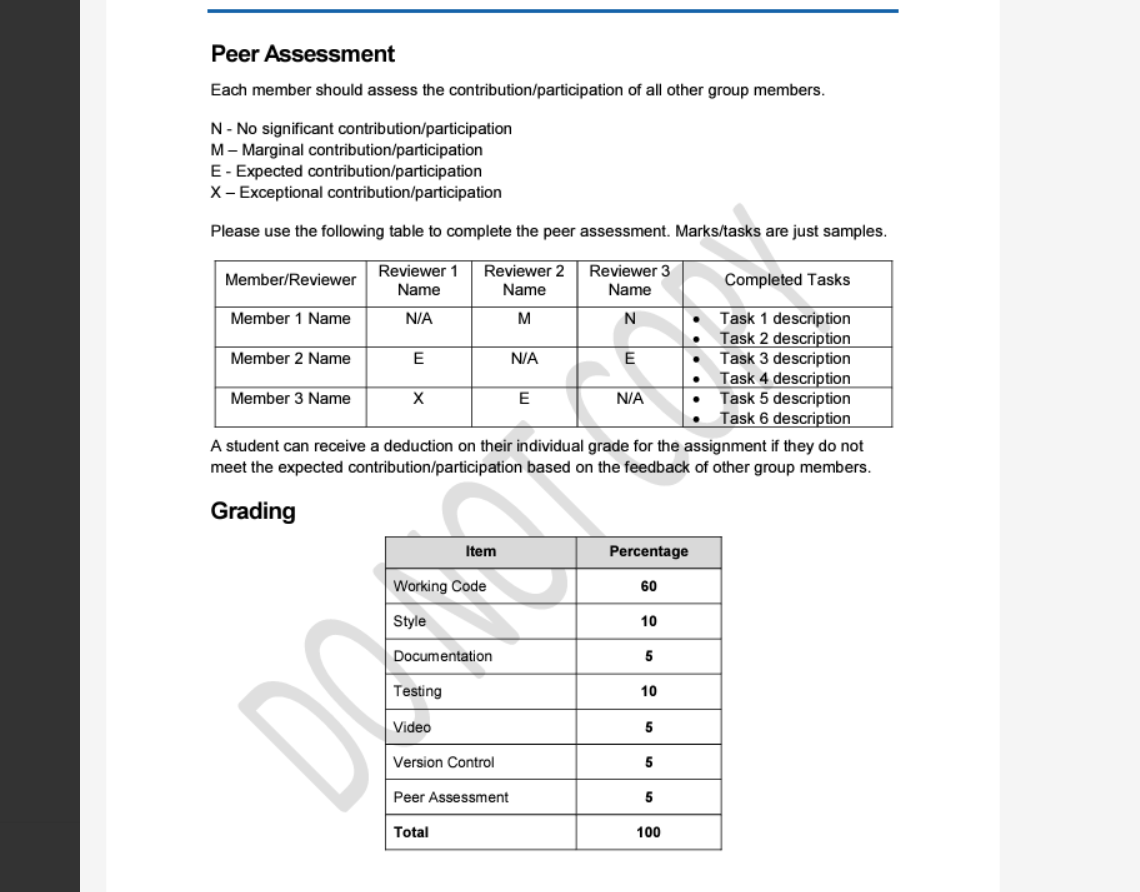
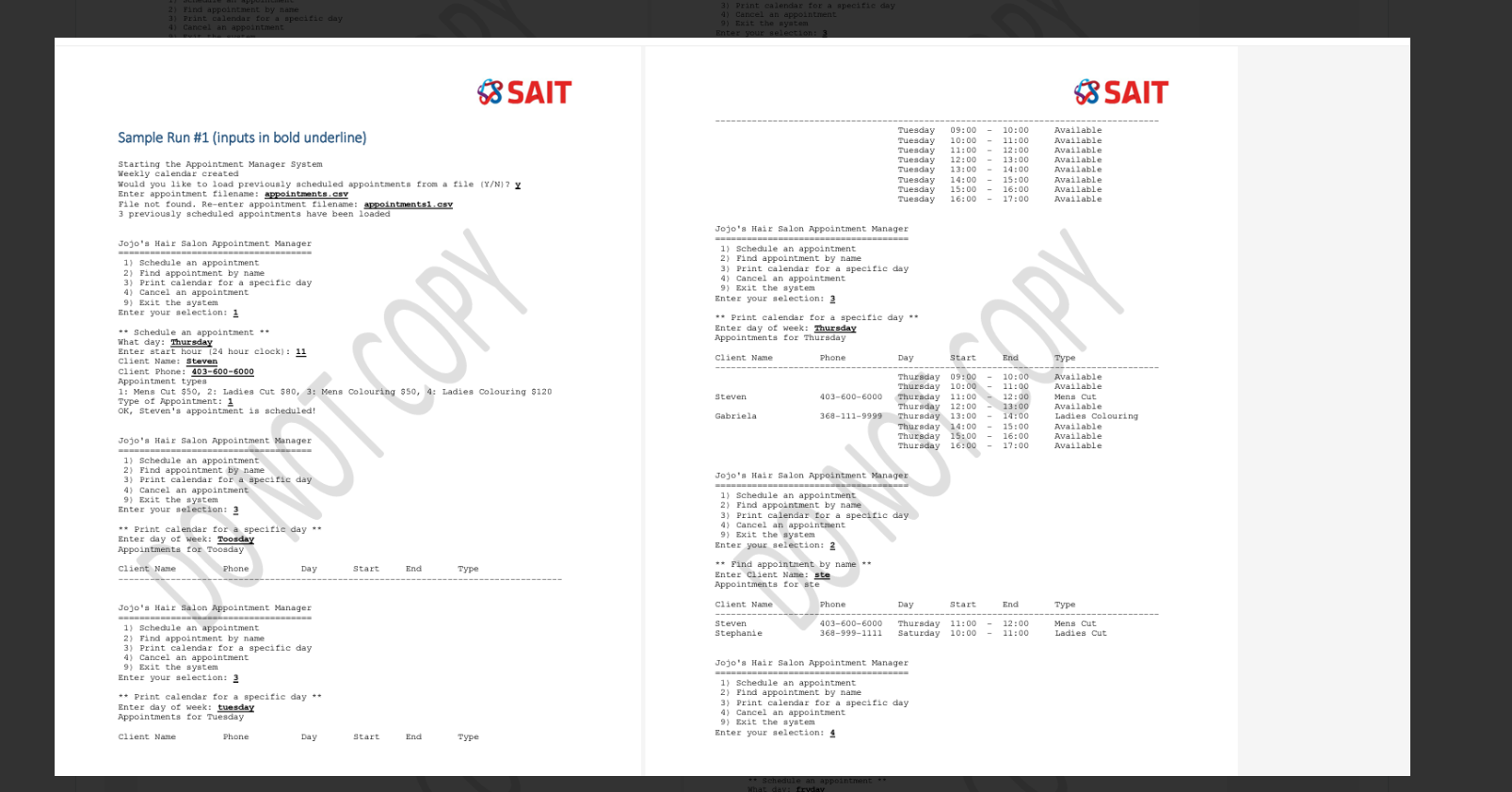
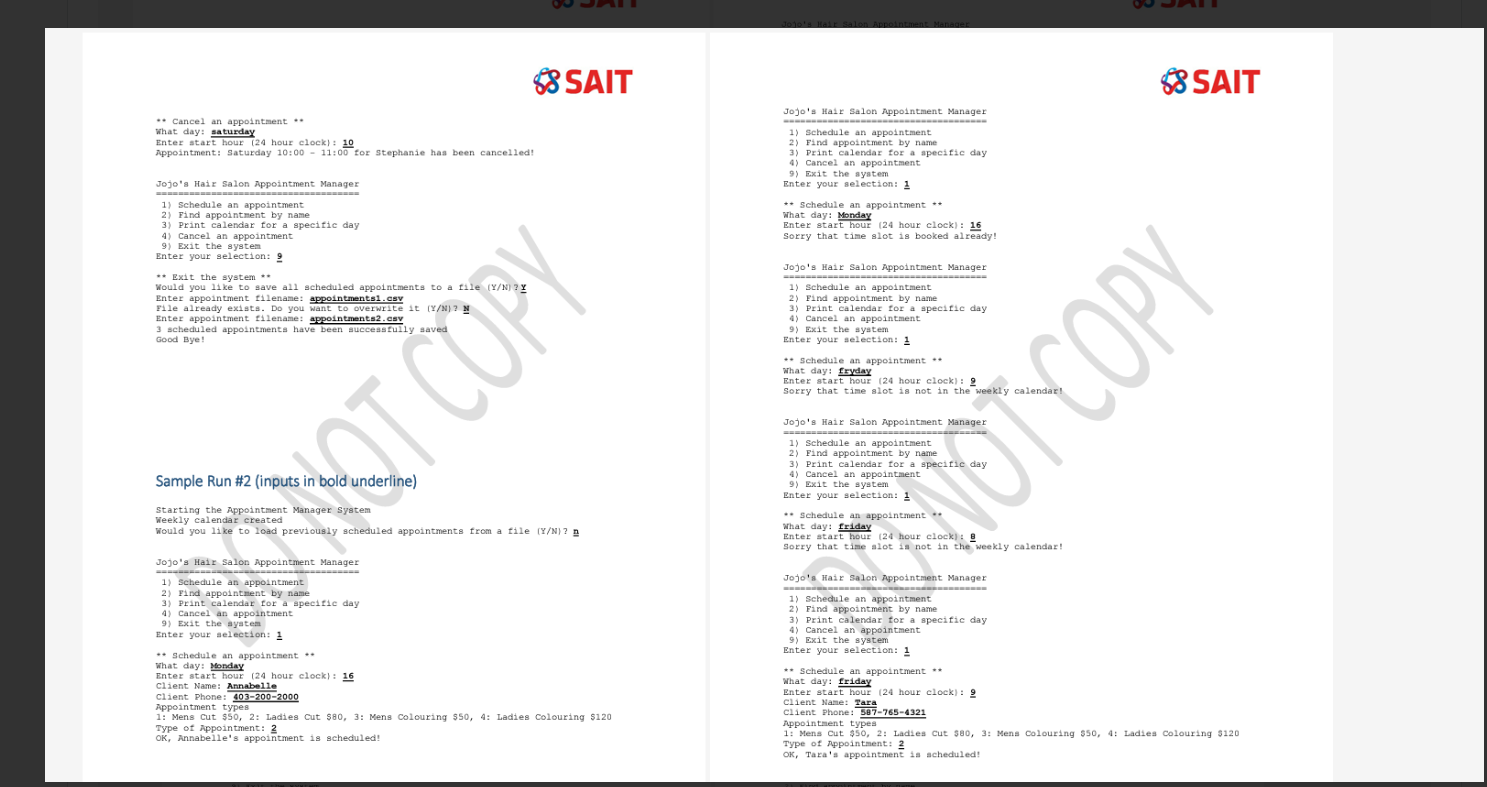
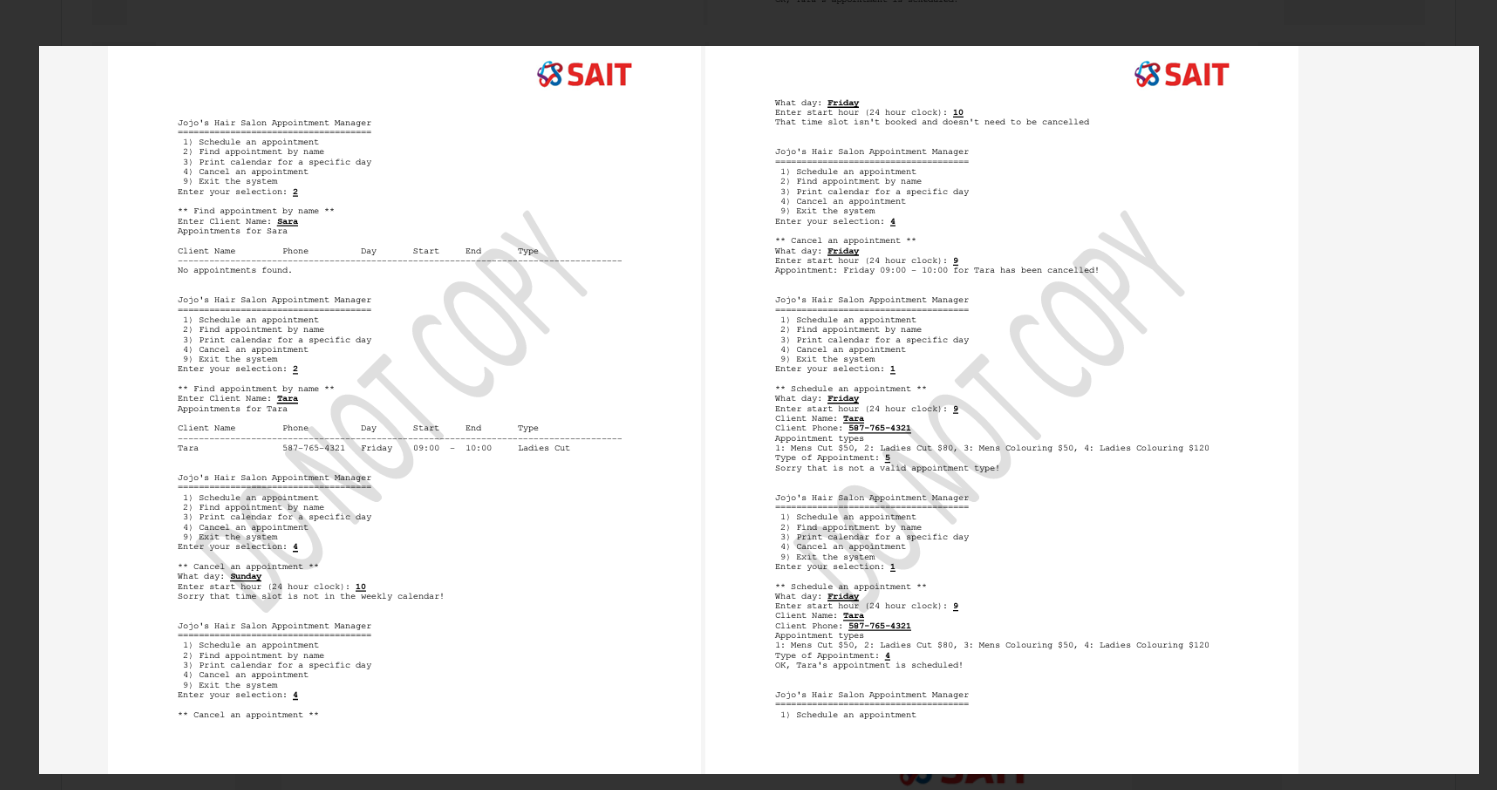
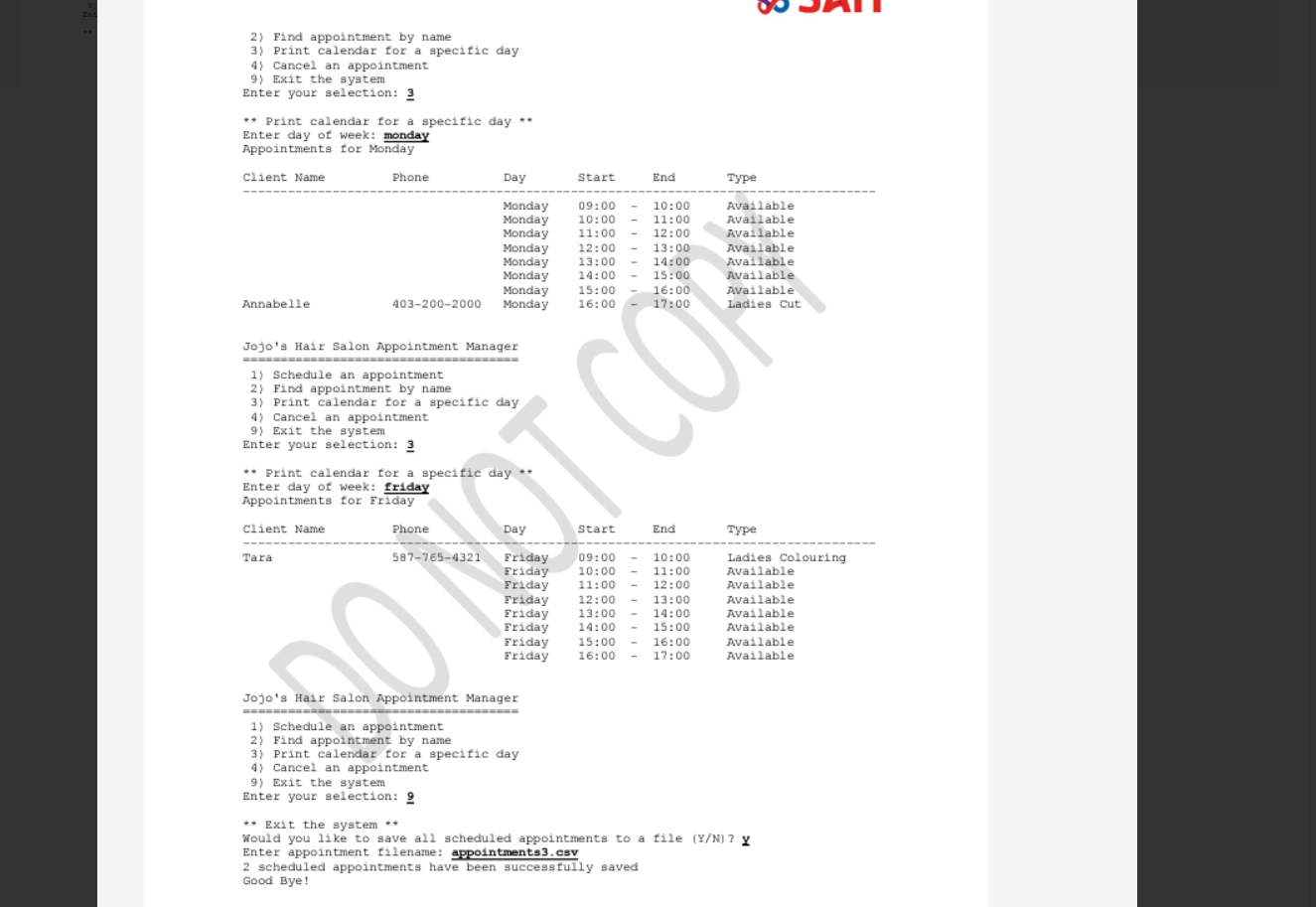
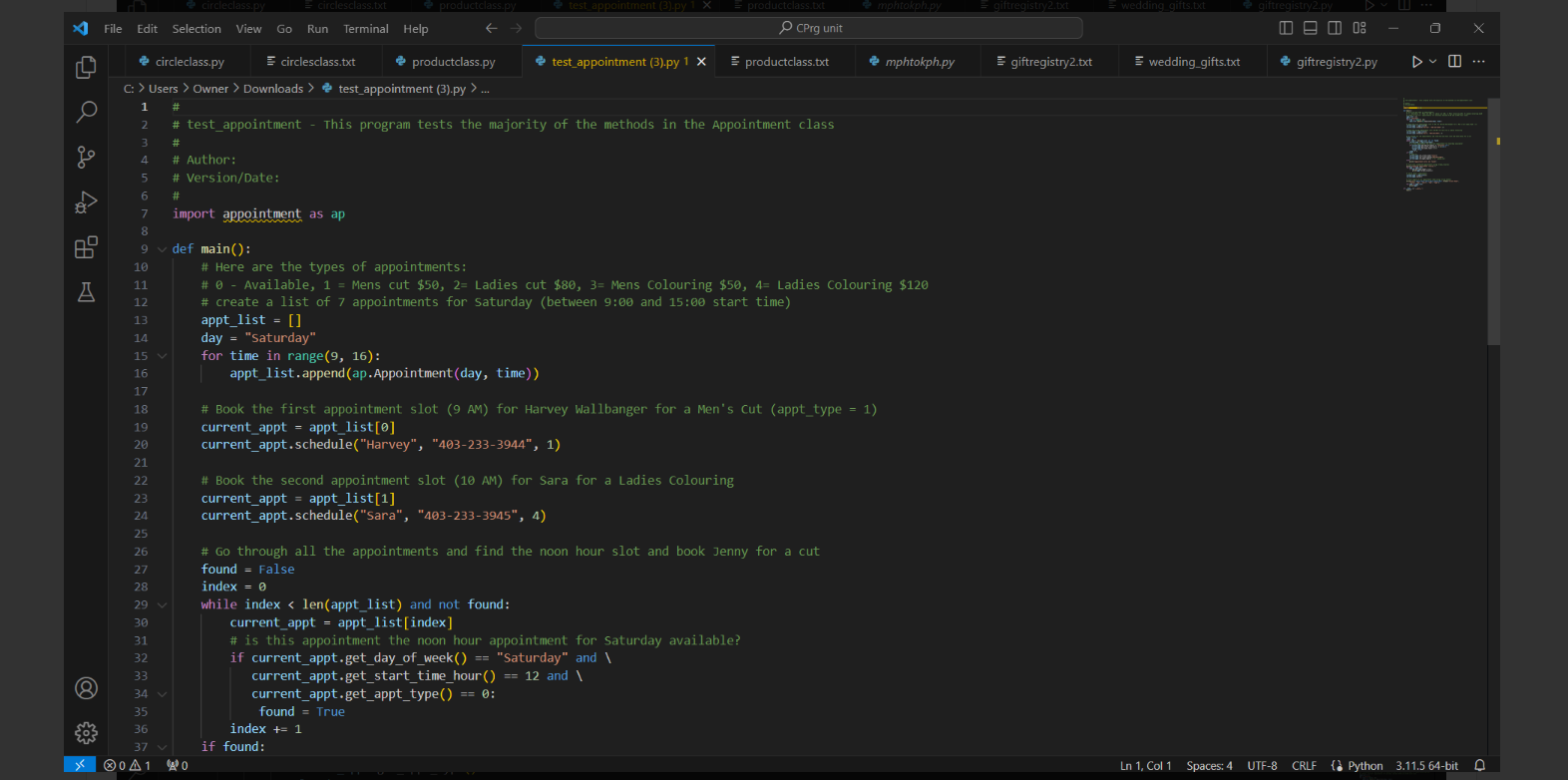
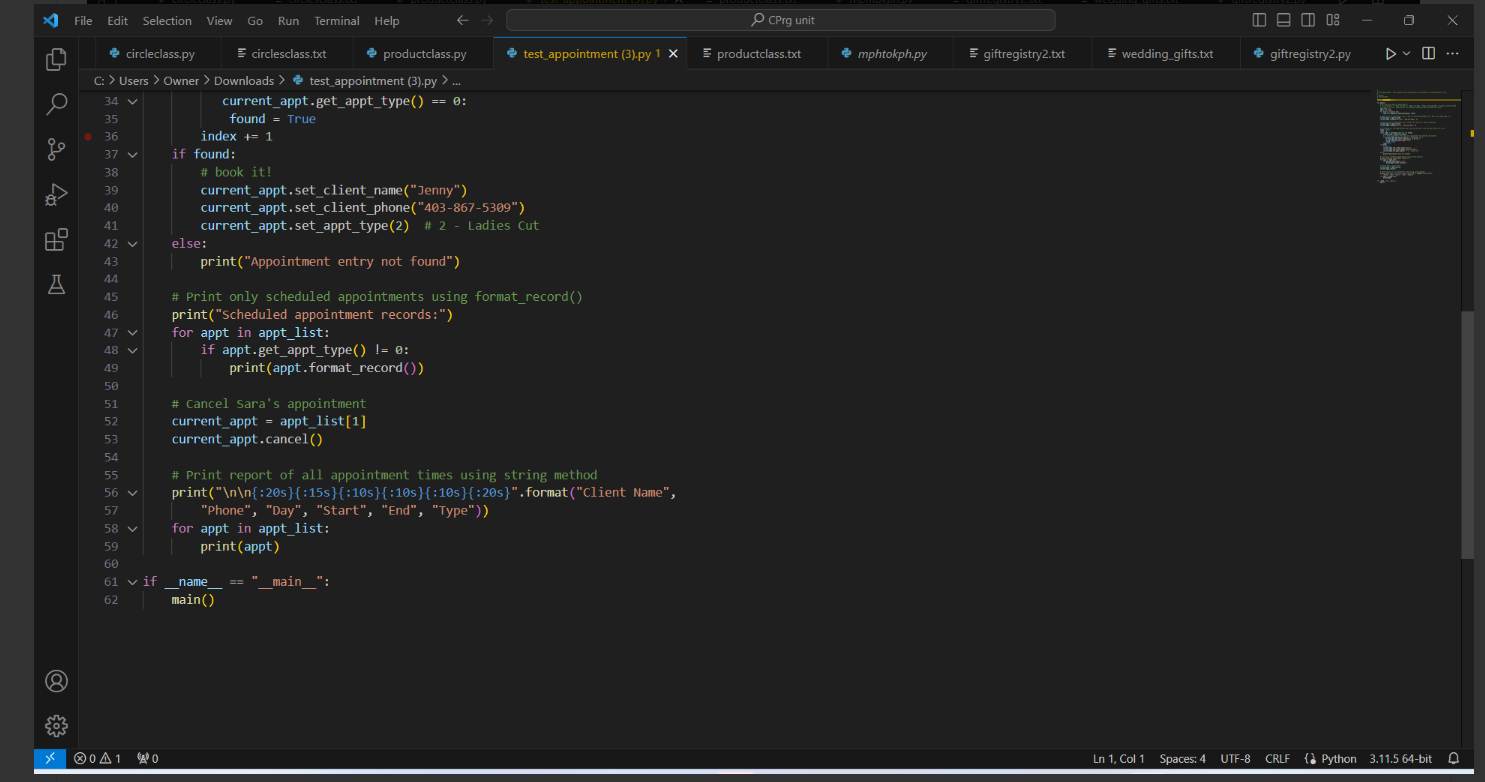
O k Student Name: Student ID: Project: Classes Type: Group Assignment Students should ONLY USE programming constructs covered in the course material. Submissions using programming concepts that are not covered in the course material will be penalized. Penalty with no limit could be applied Late submissions will not be accepted Equipment and Materials For this assignment, you will need: Python IDE Git software GitHub repository $8SAIT Scenario Jojo's Hair Salon needs a system to track customer appointments. Jojo has hired your team to create an appointment management system which is customized to her salon's business needs. . Weight: 25% Instructions This assignment consists of two parts, both completed outside of class time. See the course schedule and Brightspace for exact dates. Rubrics are available in Brightspace. Project Part 1: (No Submission) 1. Create the Appointment class as outlined in the document (recommended filename: appointment.py). 2. Test the Appointment class to verify its correct operation by running the supplied test_appointment.py program, which outputs a single line of data and reports errors in the implementation of the class. 2023, Southem Alberta Institute of Technology 3. Each student is encouraged to individually complete Part 1. At least, group members MUST equally participate in Part 1. Project Part 2: (Submission) 1. Have one member of your group set up a GitHub repository for Part 2 of this project. Make sure that this GitHub repository is private. Add group members and your instructor as collaborators. 2. Create a separate branch in GitHub for each group member, containing the part they will work on. The branch name should include the task name and the student name. 3. Develop the code for the Appointment Management Module as specified in this document. As a team, you will also need to assess each member's Appointment class and then finalize one version for your group's solution. 4. GitHub must be effectively used for group collaboration. 5. Ensure all group members update their parts to their respective GitHub branches. 6. The final project code must be pushed to master/main on GitHub. 7. Check your solution against the detailed marking criteria available in Brightspace. 8. Create a project video. Each group will submit a video to Brightspace or their GitHub Repository (either as a URL or an uploaded video file). In the video: $8SAIT EACH team member will introduce themselves - camera should be on. Please use laptop camera/screen share to create video (not a phone). Provide a demonstration of the application covering all the functionality CORRESPONDING TO THE SAMPLE RUN PROVIDED. Share the group's final solution (Python code) on screen and have EACH team member explain a portion of the code that they developed. A debugger may be used to step through sections of code (optional). 9. Submit the following files to Brightspace: A ZIP file for the whole project folder that includes: The code of the program that you implemented (.py files). A copy of your sample runs (.txt files) that correspond to the sample runs provided. All data files updated by program after the test runs have been completed. A link to your group's GitHub project repository. Video presentation. Peer assessment. 2023, Southem Alberta Institute of Technology Appointment Management System Details Jojo's Hair Salon requires that the appointment management system track the following information for each appointment: Client name Client phone number Type of appointment: 0. Available 1. Mens cut $50 2. Ladies cut $80 3. Mens Colouring $50 4. Ladies Colouring $120 $SAIT Day of week: Monday, Tuesday, Wednesday, Thursday, Friday, Saturday Start time of appointment (e.g. 9 AM) Note: all appointments are one hour long. The appointment management system needs to provide the following functionality: create a one-week calendar of available appointments for Monday - Saturday, 9 AM - 4 PM daily (last appointment is 4-5 PM) allow the user to optionally load previously booked appointments into the week's calendar . . CORY allow the user to select and perform any of the following menu functions (continuously until the user chooses to exit the system): o Schedule an appointment o Find appointment by name o Print calendar for a specific day o Cancel an appointment o Exit the system allow the user to optionally save all scheduled appointments to a file senior software developer has already done some design work on the Appointment class as well as the core functions required for the appointment management module. Please refer to the detailed information in the following two sections as you complete both programming parts of this project. Part 1 - Appointment Class Properties/Attributes (hidden) client_name, client_phone, appt_type, day_of_week, start_time_hour Methods Method Name Constructor Getters get_appt_type_desc() get_end_time_hour() Setters The schedule() cancel() format_record() _str_() Description _init__() initializes the Appointment object properties. The constructor should assign empty/zero for the first three properties (i.e. representing an available appointment) and accept two parameters for assigning to day_of_week and start_time_hour Implement one getter method for each Appointment property. The getter method should return the value of the property $SAIT An additional "getter" method that translates and returns the object's appt type as a text description, I.e.: appt type description Available Mens Cut Ladies Cut Mens Colouring Ladies Colouring 0 1 2 3 An additional "getter" method that returns the value of the object's start_time_hour + 1 Implement a setter method for the first three Appointment properties: client_name, client_phone, and appt_type. The setter method should set the property to the parameter value received Sets client_name. client_phone, and appt_type properties to the parameter values received Resets client_name. client_phone, and appt_type properties to empty/zero Returns a string representation of an Appointment object This representation should include all appointment properties separated by a comma Same format as in "appointments.csv" file, e.g.: Harvey, 403-233-3944,1, Saturday, 09 Returns a string representation of an Appointment object This representation is formatted for display, e.g.: Harvey 403-233-3944 Saturday 09:00 10:00 - Mens Cut Part 2 - Appointment Management Module Create a separate module (e.g. appt_manager.py) for your appointment management application code which will be comprised of various functions, including a main() entry function that controls the overall processing. The functions listed below are required but you may use additional functions too. Function Name create_weekly_calendar() print_menu() find_appointment_by_time() show_appointments_by_name() . load_scheduled_appointments() Inputs appointment filename from user show_appointments_by_day() . $8SAIT . . Description Iterates through each day of the week (Monday to Saturday) For each day, iterates through each available hour (9 to 16) For each hour, creates new Appointment object and adds it to the appointment list (i.e. calendar) Iterates over each line (i.e. appointment) in the file, parsing the attribute values into separate variables Calls find_appointment_by_time() to locate the corresponding appointment in the list and invoke the schedule() method to set the properties appropriately Returns the number of scheduled appointments loaded Displays the menu of application options Accepts menu selection from user until valid selection is entered Returns user's valid selection Receives the day and start hour of the appointment to find Searches the list of Appointments for corresponding day and start hour If the appointment is found, returns the Appointment object, otherwise returns nothing Receives the client name of the appointment(s) to show Searches the list of Appointments for corresponding client name, allowing for partial & non-case sensitive matches Displays all matching appointments in the format given in the Sample Run (hint: use the __str___() method implicitly) Receives the day of the appointments to show Searches the list of Appointments for the corresponding day $8SAIT Displays all matching appointments in the format given in the Sample Run (hint: use the_str__() method implicitly) save_scheduled_appointments(). Inputs appointment filename from user, checks if the file already exists and if so, allows user to proceed (i.e. overwrite the file) or repeat the filename input main() Iterates over each appointment in the list and if scheduled (i.e. appt_type != 0), writes the appointment to the file in the proper CSV format (hint: use the format_record() method) Returns the number of scheduled appointments saved Entry point for the Appointment Management System Coordinates the overall processing: o Set up a list of appointments for the week Optionally load a previous appointment schedule O O Present the menu, get and evaluate user's selection, repeating until user chooses to quit Get additional user inputs as needed Call appropriate functions and/or Appointment class methods to help perform the actions for each menu option Display relevant context/status messages Optionally save scheduled appointments to a file before ending program KI Tip: except for print_menu() and main(), the appointment management module functions listed above should all be defined to receive a list of Appointment objects as a parameter. Of course, the find_* and show_*functions may require an additional parameter or two as indicated in the table above. S Sample Run: see Project Sample Run F2023 document. Make sure your program output matches this sample run. Starting Data File: see appointments1.csv. Make sure that your application maintains the same comma-separated format established in this file. Peer Assessment Each member should assess the contribution/participation of all other group members. N - No significant contribution/participation M - Marginal contribution/participation E- Expected contribution/participation X - Exceptional contribution/participation Please use the following table to complete the peer assessment. Marks/tasks are just samples. Reviewer 1 Reviewer 2 Name Name M Reviewer 3 Name N/A Completed Tasks Task 1 description Task 2 description Task 3 description Task 4 description . Task 5 description Task 6 description A student can receive a deduction on their individual grade for the assignment if they do not meet the expected contribution/participation based on the feedback of other group members. Grading Member/Reviewer Member 1 Name Member 2 Name Member 3 Name E X Working Code Style DO Documentation Testing Item Video Version Control Peer Assessment Total N/A E 1 N N/A Percentage 60 10 5 10 5 5 5 100 . 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment Sample Run #1 (inputs in bold underline) starting the Appointment Manager System Weekly calendar created Would you like to load previously scheduled appointments from a file (Y/N)? Y Enter appointment filename: appointments.csv File not found. Re-enter appointment filename: appointments1.csv 3 previously scheduled appointments have been loaded Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 1 Schedule an appointment ** What day: Thursday Enter start hour (24 hour clock): 11 client Name: Steven client Phone: 403-600-6000 Jojo's Hair Salon Appointment Manager Appointment types. 1: Mens Cut $50, 2: Ladies Cut $80, 3: Mens Colouring $50, 4: Ladies Colouring $120 Type of Appointment: 1 OK, Steven's appointment is scheduled! 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 3 ** Print calendar for a specific day ** Enter day of week: Toosday Appointments for Toosday client Name Phone Jojo's Hair Salon Appointment Manager 1) Schedule an appointment. 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 3 client Name Day **Print calendar for a specific day ** Enter day of week: tuesday Appointments for Tuesday Phone Day Start Start $8SAIT End End OF COPY Type Type 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 3 Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system. Enter your selection: 3 Client Name ----- **Print calendar for a specific day ** Enter day of week: Thursday Appointments for Thursday Steven Gabriela Phone client Name 403-600-6000 368-111-9999 akm Steven Stephanie Tuesday Tuesday Tuesday Tuesday Tuesday Tuesday Tuesday Tuesday Jojo's Hair Salon Appointment Manager. 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 2 ** Find appointment by name ** Enter Client Name: ste Appointments for ste NOT Phone Day *** Schedule an appointment ** What day: fryday 09:00 - 10:00 10:00 11:00 11:00 - 12:00 12:00 13:00 13:00 - 14:00 14:00 - 15:00 15:00 - 16:00 16:00 17:00 Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 4 COPY Start 403-600-6000 Thursday 11:00 - 12:00 368-999-1111 Saturday 10:00 - 11:00 End $8 SAIT Available Available Available Available Available Available Available Available Type Available Available Mens Cut Available Ladies Colouring Available Available Available. Type Mens Cut Ladies Cut Cancel an appointment ** What day: saturday Enter start hour (24 hour clock): 10 Appointment: Saturday 10:00 - 11:00 for Stephanie has been cancelled! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name. 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 9 **Exit the system ** Would you like to save all scheduled appointments to a file (Y/N) ? Y Enter appointment filename: appointmentsi.csv File already exists. Do you want to overwrite it (Y/N)? N Enter appointment filename: appointments2.csv 3 scheduled appointments have been successfully saved Good Bye! 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day ** 4) Cancel an appointment 9) Exit the system Enter your selection: 1 Would you like to load previously scheduled appointments from a file (Y/N)? Schedule an appointment ** What day: Monday SAIT QT COPY Enter start hour (24 hour clock): 16 Client Name: Annabelle Client Phone: 403-200-2000 Appointment types 1: Mens Cut $50, 2: Ladies Cut $80, 3: Mens Colouring $50, 4: Ladies Colouring $120 Type of Appointment: 2 OK, Annabelle's appointment is scheduled! Jojo's Hair Sal Appointment Manager. Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 1 * Schedule an appointment ** What day: Monday Enter start hour (24 hour clock): 16 Sorry that time slot is booked already! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 1 ** Schedule an appointment ** What day: fryday Enter start hour (24 hour clock): 9 Sorry that time slot is not in the weekly calendar! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 4) Cancel an appointment 9) Exit the system Enter your selection: 1 Schedule an appointment What day: friday Enter start hour (24 hour clock): 8 Sorry that time slot is not in the weekly calendar! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day A 4) Cancel an appointment 9) Exit the system Enter your selection: 1 ** Schedule an appointment ** What day: friday Enter start hour (24 hour clock): 9 client Name: Tara Client Phone: 587-765-4321 $8 SAIT NOT COPY Appointment types 1: Mens Cut $50, 2: Ladies Cut $80, 3: Mens Colouring $50, 4: Ladies Colouring $120 Type of Appointment: 2 OK, Tara's appointment is scheduled! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 2 Find appointment by name ** Enter Client Name: Sara Appointments for Sara Client Name No appointments found. Phone Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 2 * Find appointment by name ** Enter Client Name: Tara Appointments for Tara Client Name Tara Day Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 4 587-765-4321 Friday 09:00 10:00 Cancel an appointment ** Start What day: Sunday Enter start hour (24 hour clock): 10 Sorry that time slot is not in the weekly calendar! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name. 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 4 **Cancel an appointment ** End SAIT COPY Type Ladies Cut What day: Friday Enter start hour (24 hour clock): 10 That time slot isn't booked and doesn't need to be cancelled Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 4 Cancel an appointment ** What day: Friday Enter start hour (24 hour clock): 9 Appointment: Friday 09:00 - 10:00 For Tara has been cancelled! Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 1 ** Schedule an appointment ** What day: Friday Enter start hour (24 hour clock): 2 client Name: Tara Client Phone: 587-765-4321 Jojo's Hair Salon Appointment Manager. Appointment types 1: Mens Cut $50, 2: Ladies Cut $80, 3: Mens Colouring $50, 4: Ladies Colouring $120 Type of Appointment: 5 Sorry that is not a valid appointment type! 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 1 Schedule an appointment ** What day: Friday Enter start hour (24 hour clock): 9 client Name: Tara Client Phone: 587-765-4321 $SAIT Type of Appointment: 4 OK, Tara's appointment is scheduled! COPY Appointment types 1: Mens Cut $50, 2: Ladies Cut $80, 3: Mens Colouring $50, 4: Ladies Colouring $120 Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 3 **Print calendar for a specific day ** Enter day of week: monday Appointments for Monday Client Name Annabelle ============ Phone client Name 403-200-2000 Tara Day Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 3 Monday Monday Monday 587-765-4321 09:00 10:00 11:00 Monday 12:00 Monday 13:00 Monday 14:00 ** Print calendar for a specific day Enter day of week: friday Appointments for Friday Monday Monday Start Friday Friday Friday Friday Jojo's Hair Salon Appointment Manager 1) Schedule an appointment 2) Find appointment by name 3) Print calendar for a specific day 4) Cancel an appointment 9) Exit the system Enter your selection: 9 NOT 09:00 10:00 11:00 12:00 13:00 14:00 End 10:00 2 scheduled appointments have been successfully saved Good Bye! End 10:00 11:00 12:00 13:00 14:00 15:00 15:00 - 16:00 16:00 17:00 Type Available Available Available Available Available Available Available Ladies Cut Type Ladies Colouring Available Available Available Available Available Available Available ** Exit the system ** Would you like to save all scheduled appointments to a file (Y/N) ? Y Enter appointment filename: appointments3.csv 8.9 A circleclass.py File Edit Selection View Go Run Terminal Help 3 4 5 6 7 8 9 def main(): 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 + circleclass.py circlesclass.txt productclass.py C: > Users > Owner > Downloads > test_appointment (3).py > ... 1 # 2 # test_appointment - This program tests the majority of the methods in the Appointment class # 31 32 33 34 35 36 # Author: # Version/Date: # import appointment as ap 37 V 1 0 A10 0 A = circlesclass.txt productclass.py # Here are the types of appointments: test_appointment (3).py 1 X index += 1 test_appointment (3).py 1 X if found: productclass.txt CPrg unit # Book the second appointment slot (10 AM) for Sara for a Ladies Colouring current_appt = appt_list[1] current_appt.schedule("Sara", "403-233-3945", 4) =productclass.txt #0 - Available, 1 = Mens cut $50, 2= Ladies cut $80, 3= Mens Colouring $50, 4= Ladies Colouring $120 # create a list of 7 appointments for Saturday (between 9:00 and 15:00 start time) appt_list = [] day = "Saturday" for time in range(9, 16): appt_list.append(ap.Appointment (day, time)) # Book the first appointment slot (9 AM) for Harvey Wallbanger for a Men's Cut (appt_type = 1) current_appt = appt_list[0] current_appt.schedule("Harvey", "403-233-3944", 1) # Go through all the appointments and find the noon hour slot and book Jenny for a cut found = False index=0 while index < len(appt_list) and not found: current_appt = appt_list[index] # is this appointment the noon hour appointment for Saturday available? if current_appt.get_day_of_week() == "Saturday" and \ current_appt.get_start_time_hour() == 12 and \ current_appt.get_appt_type() == 0: found = True mphtokph.py mphtokph.py giftregistry2.txt giftregistry2.txt wedding gifts.txt wedding_gifts.txt giftregistry2.py 08 giftregistry2.py ^ < T SENI BUALANUVEL Airsan www H www. H 20 Ln 1, Col 1 Spaces: 4 UTF-8 CRLF Python 3.11.5 64-bit x e a 9 4 A File Edit Selection View Go Run Terminal Help + circleclass.py circlesclass.txt productclass.py C: > Users > Owner > Downloads > test_appointment (3).py > ... 34 current_appt.get_appt_type() == 0: 35 36 37 V 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 vif 62 A1 O index += 1 found = True if found: # book it! current_appt.set_client_name("Jenny") current_appt.set_client_phone("403-867-5309") else: current_appt.set_appt_type(2) # 2 - Ladies Cut print("Appointment entry not found") # Print only scheduled appointments using format_record() print("Scheduled appointment records:") for appt in appt_list: if appt.get_appt_type() != 0: print (appt.format_record()) # Cancel Sara's appointment current_appt = appt_list[1] current_appt.cancel() name main() test_appointment (3).py 1 X # Print report of all appointment times using string method print(" {:20s}{:15s}{:10s}{:10s}{:10s}{:20s}".format("Client Name" "Phone", "Day", "Start", "End", "Type")) for appt in appt_list: print (appt) _main__": O CPrg unit =productclass.txt mphtokph.py giftregistry2.txt wedding_gifts.txt 908 giftregistry2.py Ln 1, Col 1 Spaces: 4 UTF-8 CRLF 0 POST SOON MA 20 We wwwww... www.w Bayman... K CRLFPython 3.11.5 64-bit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
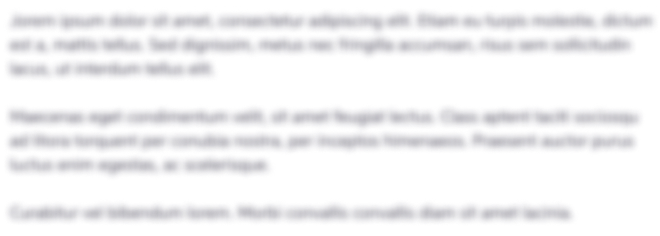
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started