Question
Object Oriented Paradigm Assignment-1 OBJECTIVES Create and submit a project report Trace Code to determine output. Explore simple programming mistakes and the related errors produced
Object Oriented Paradigm
Assignment-1 OBJECTIVES Create and submit a project report Trace Code to determine output. Explore simple programming mistakes and the related errors produced by the compiler. SUBMISSION Save all work on your flash drive before you submit to blackboard. Submit a copy of the report (Microsoft Word file or a pdf file). The report should have a title sheet that includes your name, date and the assignment number. The report should include a copy of source code and an output. PROGRAM 1 Create a version of the TempConverter application to convert temperature values from Fahrenheit to Celsius. Read the Fahrenheit temperature value from the user. ALGORITHM 1. Initialize a variable (base) and assign to 32. 2. Initialize another variable (conversion_factor) as double to store 5.0/9.0 3. Assign a variable to store Fahrenheit temperature value and Celsius value. To read the values from user, use the scanner object and print a statement to read the value from the user. 4. Use the formulae to convert the Fahrenheit temperature value to Celsius Temperature value. a. Celsius = (Fahrenheit-base) * conversion_factor 5. Then print both the temperature values in different lines. CODE TEMPLATE //Name: //Date: import java.util.Scanner; public class TempConverter { //----------------------------------------------------------------- // Reads Fahrenheit temperature values and converts the equivalent in Celsius temperature //----------------------------------------------------------------- public static void main(String[] args) { final int BASE=32; 2 final double CONVERSION_FACTOR = 5.0/9.0; double fahrenheitTemp, celsiusTemp; // use the scanner object to read the temperature value in Fahrenheit from the user // convert the temperature using the formulae CelsiusTemp = (fahrenheitTemp - BASE)* CONVERSION_FACTOR; // print the values of the Fahrenheit Temperature and Celsius Temperature } } 3 PROGRAM 2 Write an application that prompts for and reads a double value representing a monetary amount then determine the fewest number of each bill and coin needed to represent that amount. Starting with the highest (assume that the ten dollar bill is maximum needed). For example if the value entered is 47.63 then the program should print the output as 4 ten dollar bills 1 five dollar bill 2 one dollar bills 2 quarters 1 dime 0 nickels and 3 cents ALGORITHM: 1. Initialize a variable as double to read a value from the user the money to be converted into dollars, quarters, dimes and etc. 2. Use scanner object to read the value. 3. Multiply the value with 100 so as to convert the total value into cents. 4. Perform division operation so as to get the quotient which is number of ten dollar bills. 5. To get the remainder we now use modulo operator and get the remainder which is used in the next steps to determine the number of 5 dollar bills and so on. 6. If desired you can also print the remainder so as to keep the track of remainder. 7. The remainder thus obtained from the above step is used to get the number of 5 dollar bills and again modulo operation to get the remainder. 8. The remainder obtained in the above step is further used to get number of dollar bills by performing division and modulo operation to get the remainder. 9. Similarly, perform the same operations as above to get the number of quarters, dimes and cents by dividing the value obtained in the above respective steps. 4 CODE TEMPLATE //Name: //Date: import java.util.Scanner; public class MoneyConversion { //----------------------------------------------------------------- // Reads a monetary amount and computes the equivalent in bills // and coins. //----------------------------------------------------------------- public static void main (String[] args) { double total; int tens, fives, ones, quarters, dimes, nickels; int remainingCents; Scanner scan = new Scanner(System.in); System.out.print ("Enter monetary amount: "); total = scan.nextDouble(); remainingCents = (int) (total * 100); // perform divisions and perform modulo operations repetitively until you get the number ten dollar // bills, five dollar bills, one dollar bills, quarters, dimes and cents System.out.println ("That's equivalent to:"); System.out.println (tens + " ten dollar bills"); System.out.println (fives + " five dollar bills"); System.out.println (ones + " one dollar bills"); System.out.println (quarters + " quarters"); System.out.println (dimes + " dimes"); System.out.println (nickels + " nickels"); System.out.println (remainingCents + " pennies"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
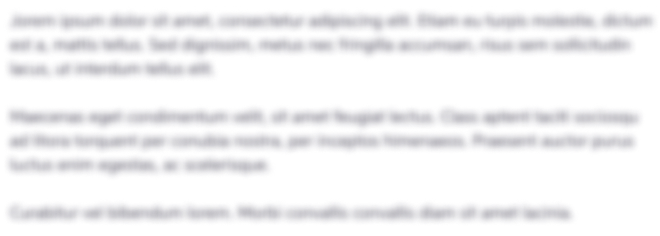
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started