Objective : Complete a Node.js app that makes use of JavaScript arrays to store and retrieve objects. Learn more about the use of node.js modules
Objective: Complete a Node.js app that makes use of JavaScript arrays to store and retrieve objects. Learn more about the use of node.js modules and JS classes to abstract code. Implement a testing strategy so that the app has functional unit tests.
Scenario: Your brother-in-law needs you to build an app to help him track inventory in his warehouse. The warehouse has rows (indicated by a numbers 0-24) and squares (indicated by a numbers 0-9). So a location is a row/square pairing, like 8,3. Inventory that is received is stored in "lots" at a certain location in the warehouse, and they are assigned a unique lot id upon receipt.
When the customer wants to ship an item out of the warehouse, a shipping lot is created and assigned its own id. Inventory is transferred from the receiving lot to the shipping lot, and the shipping lot is stored in a separate location of the warehouse for export.
Task: Build a terminal-based node app that will present the user with a menu that allows them to do one of three things:
Add inventory at a location
Ship inventory from a location
Quit the program
Much of the program has been implemented for you. The main thing you are required to do is implement the methods of the program and design suitable test cases to thoroughly test the program.
Warehouse Module
The warehouse module is a node.js module that abstracts warehouse storage for both warehouse and outbound inventory. The properties and methods have been documented in the starter package. The inventory arrays have been provided, but you need to implement the methods.
Warehouse Inventory
You have been provided with a two-dimensional array to store your inventory. This simply means that we're using one array to store the rows, and at each location in that array, you'll use another array to represent the squares. Each "square" will be a place that a lot can be stored.
Outbound Inventory
You have also been given an outbound inventory queue (as a simple array) that will contain lot objects waiting for shipment.
Lot Module
The lot module implements a class with these properties:
id -auto generated at creation
amount - amount of inventory in this lot
notes - Alphanumeric text about this lot
shipInventory(amountShipped) - a method that:
takes in an amount of inventory to ship
decrements the inventory amount
returns a new lot object containing the shipped inventory amount and a copy of the notes, with a brand new lot id
If an invalid ship action is attempted, the function should return false
--------------------------------------------------------------------------------------------------
// Gives the user a menu and gets their chosen action
function getActionChoice() {
// Represents the option chosen from this menu
action = readlineSync.question('What would you like to do? 1:Build lot 2:Find lot 3:Quit');
return action;
}
// Interacts with the user to build a new lot object
function buildLot() {
// make sure they enter a valid amount
// Get the notes as well
// Return a new lot object based on this input
}
// Interacts with the user to ask how much they want to ship
function getShipAmount(currentAmount) {
// Make sure the user enters a valid number that isn't greater than the amount that can be shipped
}
// Interacts with the user to ask where a lot should be, returns an object with row and location numbers
function getLocation() {
// Get a valid row number
// Get a valid square number
// Return an object containing both values
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
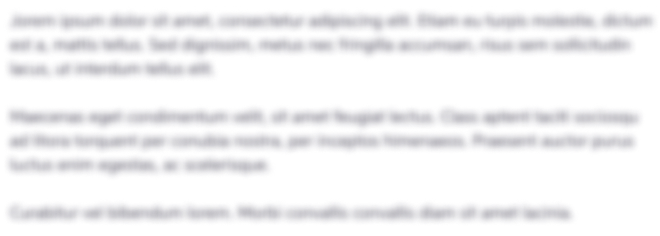
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started