Question
Objective: Develop class definitions and implementations to demonstrate C++ inheritance: Specifically, you are to: 1. Develop an abstract base class called Vehicle (in a header
Objective: Develop class definitions and implementations to demonstrate C++ inheritance: Specifically, you are to: 1. Develop an abstract base class called Vehicle (in a header file vehicle.h and implementation file vehicle.cpp) having data members: a. A name (type char*) which stores the name of the Vehicle object as a C-style character array and b. A static attribute out (an ostream*) which is the output object for the print function (see member functions below) initialized to reference standard output (i.e., &cout). Vehicle should use a protection scheme for its attributes which allows derived classes (but not general users of the class) the ability to inspect or modify the attributes. The member functions for Vehicle are: a. A default constructor initializing name to the empty string b. A constructor taking a single string parameter to initialize the name. Since name is stored internally as a char*, the constructor should handle the conversion internally. NOTE: you may combine this constructor with the default constructor via default parameters. c. A copy constructor which provide for a non-aliased (i.e., deep) copy of a Vehicle object. d. An assignment operator providing a deep copy on a Vehicle. e. A destructor which returns any storage allocated in the constructors (or assignment operator). The destructor should be specified so that it and its descendants are dynamically dispatched. f. A pure virtual function print having the signature: void print(). g. A pure virtual function read having the signature: void read(). 2. Develop another class called MotorVehicle, derived from Vehicle, within files motorVehicle.h and motorVehicle.cpp, having additional data members: a. make (type string) which stores the name of the motorVehicle manufacturer (e.g, Ford, Jeep, etc.), b. model (type string) which stores the nameplate (e.g., Focus or Wrangler), and c. mpg (type double) which stores the combined EPA fuel economy estimate in miles per gallon. In addition, the MotorVehicle class should have as member functions: a. A default constructor. Default values are empty strings for name, make and model, and zero for the mpg. b. A constructor taking initial values for all four non-static attributes (or you may combine these constructors if you choose). Again use the string type as a parameter for the name. c. Accessor and Mutator functions for all five attributes (i.e., out, name, mpg, model, and make). Accessors and mutators should be named in the standard fashion (e.g., getName(), setMpg(), etc.). d. The accessor and mutator for name should use string as the return type and parameter, respectively, and handle the conversion to char* internally. Additionally, the accessor for out should return an ostream& and the mutator for out should have a single ostream& parameter. e. A print function which overrides its base class and prints each non-static attribute of MotorVehicle to the out attribute. NOTE: because the out attribute is an ostream*, the syntax for printing to out becomes: *out << valueToPrint; f. A read function which overrides its base class and, for each non-static attribute, writes a descriptive prompt to stdout to request a value and then reads that value from stdin. For example, for the mpg attribute MotorVehicle::read() could output a prompt such as, Please enter the miles per gallon for this vehicle: to stdout and then read from stdin a mpg value. NOTE: To keep the read function simple, read all input as a string without white space and then (if necessary) convert the value to the proper type. Input validation on the values you read is not required. 3. Develop two derived classes called Car (in files car.h and car.cpp) and Truck (files truck.h and truck.cpp) each inherited from MotorVehicle. Each derived class should support similar kinds of constructors, accessors, mutators, and I/O functions as MotorVehicle (e.g., there should be a default constructor and a constructor initializing all non-static attributes, and there should be accessors and mutators for the new attributes, the read function should operate similarly as before, etc.). In addition: a. Car should have an attribute trim (type string). b. Truck should have an attribute cargoCapacity (type double). 4. Write the overridden functions for read and print to use the capabilities of their parent class versions. For example, since the MotorVehicle version of print writes the values of name, mpg, model and make to out, the derived version of print should NOT re-create this capability. 5. Test your classes using the program vehicleTest.cpp and the test file vehicleTestCases.txt. If your classes are properly written, you will be able to exercise the test program without modifications. 6. NOTE: The test program and test cases I have provided does not test all capabilities of your implementation (e.g., such as self-assignment from one Vehicle to another) so you may want to modify one (or both) of the test cases or program in order to fully exercises the code you have written (I know your instructor will :-).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
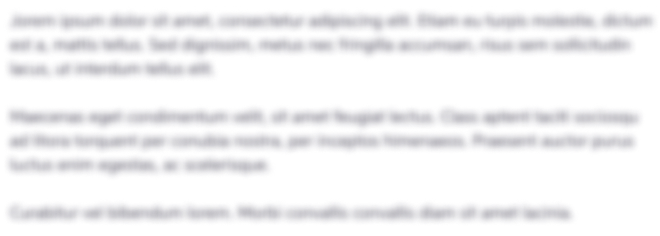
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started