Question
Objective: Learn basic C# Programming Language A certain automobile dealership sells fifteen different models of automobiles and employs ten salesmen. A record of sales for
Objective: Learn basic C# Programming Language
A certain automobile dealership sells fifteen different models of automobiles and employs ten salesmen.
A record of sales for each month can be represented by a table, the first row of which contains the
number of sales of each model by salesman 1, the second row contains the number of sales of each
model by salesman 2, and so on. For example, suppose that the sales table for a certain month is the
following:
0 0 2 0 5 6 3 1 3 4 3 3 1 0 2
5 1 9 0 0 2 3 2 2 3 1 4 6 2 1
0 0 0 1 0 0 0 3 1 6 2 1 7 3 2
1 1 1 0 2 2 2 5 2 7 4 2 8 2 1
5 3 2 0 0 0 5 2 1 2 6 6 6 1 4
4 2 1 0 1 1 0 4 4 1 5 5 1 2 1
3 2 5 0 1 2 0 1 3 5 4 3 2 1 2
3 0 7 1 3 5 1 0 6 8 2 2 3 2 1
0 2 6 1 0 2 2 1 1 2 1 5 4 3 0
4 0 2 3 2 1 3 2 3 1 5 4 0 1 0
Figure 1
The automobile models are defined as follows.
Camry, Corolla, Tercel, Protege, Mazda3, Mazda6,Altima, Maxima,
Sentra, Diamante, colt, Mirage, Legacy, Stylus, Impulse.
A Console Based C# program to be written to accomplish the following requirements:
1.The C# program must manage data using a 2-Dimensional array of integers (table in figure 1).
2.The program must be menu driven (See Figure 2)
Your system should be menu driven with the following options.
General Auto Sales
==================
1.Add a new sale
2.Sales Per Salesman by model
3.All Sales Per Salesman
4.All Sales For The Company
5.Exit
Figure 2
1.Add a new sale. When this option is selected a screen is popped up requesting the following
information.
Enter Transaction Code :
A transaction code is a 4 character string. For example 0912 is a valid transaction code. The first two
characters represent the salesman code , and the last two characters represent the automobile model
code. Once the transaction code is entered at the above prompt, a verification is required check if the
entered code is a valid one. If the code is invalid then an error message is displayed. If the
entered code is a valid one, the value of the (9,12) location of the table must be incremented by 1.
[Note: In C#, array indices start from 0. Therefore 0, 0 location is a valid location for a 2-D array.
Therefore 0000 is a valid code.
2.Sales per salesman by model. If this option is selected, again, similar transaction code (as described in
1)is entered first. (The verification MUST be done for all options)
Suppose the code 0111 was entered. This is a valid code. This means salesman 01, 11th model. Read
the (01,11) location of the 2-D array and display the following information
Sales Representative Code : 01
Automobile Model : Mirage
Total Sold By This Representative : 4
3. All Sales Per Salesman. This is similar to option 2 except for that all models sold by that representative
is displayed.
As an example , say the code 0809 was entered. This is also a valid code. The following information is
displayed on the screen.
Sales Representative Code : 08
Automobile Model Number Sold
Camry 0
Corolla 2
Tercel 6
Mazda3 1
Marda6 0
Protg 2
Altima 2
Maxima 1
Sentra 1
Diamante 2
Colt 1
Mirage 5
Legacy 4
Stylus 3
Impulse 0
Total 30
==========
4.Total Sales for the company : A monthly sales report must be produced in the following form:
MODEL
Salesman Ca Cr Te M3 M6 Pro Alt Max Sen Dia Col Mir Leg Sty Imp Total
0 0 0 2 0 5 6 3 1 3 4 3 4 3 1 0 35
1 5 1 9 0 0 2 3 2 2 3 1 3 6 2 1 40
2 0 0 0 1 0 0 0 3 1 6 2 1 7 3 2 26
3 1 1 1 0 2 2 2 5 2 7 4 2 8 2 1 40
4 5 3 2 0 0 0 5 2 1 2 6 6 6 1 4 43
5 4 2 1 0 1 1 0 4 4 1 5 5 1 2 1 32
6 3 2 5 0 1 2 0 1 3 5 4 3 2 1 2 34
7 3 0 7 1 3 5 1 0 6 8 2 2 3 2 1 44
8 0 2 6 1 0 2 2 1 1 2 1 5 4 3 0 30
9 4 0 2 3 2 1 3 2 3 1 5 4 0 1 0 33
-------------------
-----
-----
-----
-----
-----
-----
----------
----
------
-----
------
25
11 35 6 13 21 19 21 27 39 33 35 40 18 12 357
In the actual print out, all the above abbreviated names should be printed as full names as shown in
the type declaration above.
You can hardcode the initial 2-D array to the following. You can hardcode the initial 2-D array to the following.
0 1 0 0 0 0 0 1 0 0 0 0 0 0 0
0 0 0 1 0 0 0 0 0 0 0 0 0 1 0
0 0 0 0 0 0 1 0 0 0 0 0 0 0 0
0 2 0 0 0 0 0 0 0 0 0 0 1 0 0
0 0 0 1 0 0 0 0 0 0 1 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
1 0 0 0 0 0 0 0 1 0 0 0 0 0 0
0 1 0 0 0 0 0 0 0 0 0 0 0 0 0
2 0 0 0 0 0 0 0 0 0 0 0 0 0 1
0 0 0 0 0 0 1 0 0 1 0 0 0 0 0
Figure 2
Initial 2-D Array
(Note : 0000 is a valid code)
In your program code, you MUST make sure (verification & validation is required) that the correct code is
used to access the table. For example, the user enters the code 1512. This is an invalid code. Your
program must be intelligent enough to detect this and display a proper error message.
For testing purposes you may build your own set of data. You must test your application using the given
scenario.
All output files must have appropriate headings and self documenting information. Identifiers must be
meaningful. The program should be laid out neatly. Block structure should be used to localize
declarations (Avoid Global Variables as much as possible). Whenever possible a piece of information
should be written once; thus numbers and constant declarations should be used rather than explicit
literals. Make all subroutines shorter as possible.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
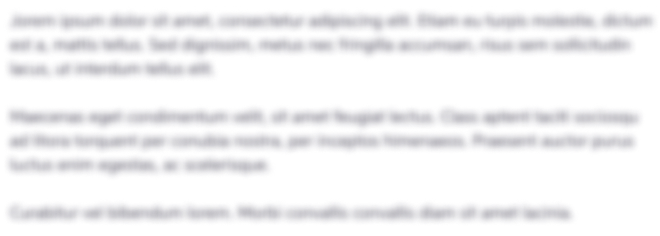
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started