Question
Objective Practice writing and understand custom functions in C. *Create a flash card program for multiplying single digit integers. *A flash card is conventionally an
Objective
Practice writing and understand custom functions in C.
*Create a flash card program for multiplying single digit integers.
*A flash card is conventionally an index card where one side has a question and the other side contains the answer to the question.
1.
You are required to create at least 3 custom functions that provide some functionality of the flash card program.
*At least 1 function needs to return a value.
*At least 1 function needs to have parameters.
*The main() does NOT count towards the 3 requirement.
2.
Do NOT create any global or static variables in your assignment. All variable declarations must be inside main, or in your custom functions.
In general, you should only use global or static variables when needed. For this program, neither are not needed.
Global variables can cause unintended side-effects and potentially security issues because it's value may be accessed and modified at any point in the code.
An objective of the assignment is to understand and implement custom functions that have parameters and return a value.
3.
Declare all function prototypes above the main function and define all functions below main.
Function prototypes and function definitions are different.
A function prototype is a declaration of a function, a function definition is the actual function that contains the function body.
4.
Create a program named numberCalculator.c, that does the following:
Setup your program to use the custom getdouble() function. Ensure the necessary files in the directory and include in the program.
Setup your program to generate a random number.
* Include the necessary header files at the top of your program. There are 2 includes that are associated with random numbers. In total, you should have 4 includes after setting up your program to generate random numbers.
* Seed the random generator using UNIX time.
Declare and initialize an integer variable that will be used to keep track of how many questions the user answered correctly.
Declare and initialize another integer variable that will be used to keep track of how many questions the user answered incorrectly.
When the program starts, output a message telling the user the purpose of the program.
Ask the user how many questions do they wish to answer.
Use the custom getdouble() function to get the number and store it in an integer variable. Typecast the return value when assigning to the variable. For example: int numQuestions = (int) getdouble();
Perform an error check on the input. If the number is less than 1, output a message to the user to enter an integer 1 or more, and the program should end.
If the number is 1 or more, proceed with the rest of the program.
The program will need to generate two random integers in the range 0 to 9, inclusive.
The program will also need to calculate the answer to determine if the user was correct.
Have the program display the question in the following format: QX: What is A times B?
1. Where X is the question number
2. A is the first random integer
3. B is the second random integer
Use the custom getdouble() function to get the user's answer.
If the user answers correctly, tell the user they got it correct, record the score.
If the user answers incorrectly, tell the user they got it incorrect, display the correct answer, and record the score.
The program should repeat the process (bullets #10 - 15) for as many times the user wanted.
After the last question has been answered, output a message showing how many questions were correct and how many were incorrect.
Additionally, print a percentage breakdown of correct and incorrect.
*Display the percentages to 2 decimal places.
*To print a single percent sign using printf, use the format specifier %%
*Be careful of integer division. When you calculate the percentage, you may end up with an integer. To remedy this issue, multiply by 100.0 instead of 100, this will force floating-point arithmetic. For example: double percentCorrect = 100.0 * numberCorrect / (correctNumber + incorrectNumber);
Double-check that you met the additional requirements of the assignment outlined in (1) and (2).
Be sure to have a program description at the top and in-line comments.
Each custom function needs to have a description at the top as well as comment inside the function.
NOTE:
Try to keep your custom functions simple. You don't want to do too much in a function because (1) variable scope, and (2) if you need to return a value, you can only return one value.
Here are some suggestions for functions:
A function that simply prints the introduction when called.
Asks the user how many questions they want to do, gets the number, and returns the number.
Generates a random integer between 0 and 9 and returns it.
Prints the question given the two random integers as arguments.
Determines if the user's answer was correct or incorrect. Return 1 meaning correct and -1 if incorrect.
Prints the breakdown of correct and incorrect.
Because the variables that record the amount of correct and incorrect are declared and initialized in main, do not make a function that attempts to increment those variables. Those variables have variable scope in main so the incrementing of the correct and incorrect needs to happen in main. Example outputs:
% make % ./program Multiplication flash card program! This program is designed for single-digit multiplication problems. How many questions would you like to answer? 0 Error: Please enter an integer greater than or equal to 1.
% ./program Multiplication flash card program! This program is designed for single-digit multiplication problems. How many questions would you like to answer? 7 Q1: What is 9 times 6? 54 Correct! Q2: What is 9 times 7? 61 Sorry, incorrect. The answer was 63. Q3: What is 9 times 9? 0 Sorry, incorrect. The answer was 81. Q4: What is 6 times 3? 9 Sorry, incorrect. The answer was 18. Q5: What is 4 times 3? 12 Correct! Q6: What is 7 times 8? 56 Correct! Q7: What is 6 times 3? 1 Sorry, incorrect. The answer was 18. ~~~~~~~~~~~~~~~~~~~~~~~~ All questions answered, here are your results. Number correct: 3 Number incorrect: 4 Percentage of correct: 42.86% Percentage of incorrect: 57.14%
Step by Step Solution
There are 3 Steps involved in it
Step: 1
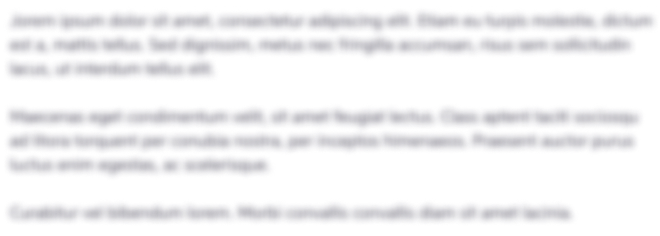
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started