Question
OBJECTIVE The objective of this assignment is to give you practice with the basic shell commands in Unix, with constructing a program in C that
OBJECTIVE
The objective of this assignment is to give you practice with the basic shell commands in Unix, with constructing a program in C that reads and writes basic data types, and with binary operations and representations of those basic data types.
C PROGRAM
Introduction
The Internet Protocol (IP) is the principal communications protocol for all devices connected to the Internet. Each device that participates in a computer network that uses IP for communication is identified by its IP address, a numerical label consisting of 32 bits. These 32-bit addresses are canonically represented in dot-decimal notation, which consists of four integer numbers, each ranging from 0 to 255, separated by dots, e.g., 128.208.252.2 (copy and paste this address into the Web browsers address field, if you are curious what this particular location is).
For routing purposes, when information is sent over the Internet, it is divided into packets and each packet is first and foremost identified by the network it belongs to (similar to a zip code of the mailing address) and only then by the specific location on that network (similar to the street address). To convey both the network IP (also called network prefix) and the specific location IP (also called host IP), instead of encoding both IP addresses separately, the notation is constructed that reads as follows:
one IP address
a slash character
an integer that denotes the routing mask
In order to figure out where to send the packet, the routers have to decode this notation to find the network IP and the specific location IP. In order to do so, the routers use the provided routing mask (also called a subnet mask) in
conjunction with the provided IP address. The routing mask integer is simply the count of the leading 1s in the IP address and the notation could be decomposed by using the bitwise AND operation of that IP address and the subnet mask.
The following example shows how the routers figure out the separation of the network IP and the host IP using the notation described above for the message address: 192.168.5.130/24.
Binary form | Dot-decimal notation | |
IP address | 11000000.10101000.00000101.10000010 | 192.168.5.130 |
Subnet mask | 11111111.11111111.11111111.00000000 | 255.255.255.0 |
Network prefix (IP address AND subnet mask) | 11000000.10101000.00000101.00000000 | 192.168.5.0 |
Host part (IP address AND ones complement of subnet mask) | 00000000.00000000.00000000.10000010 | 0.0.0.130 |
Problem Statement
Create a program that converts IP addresses to/from their dot-decimal notation using primitive data types and bitwise operators (i.e. arrays and strings are NOT allowed). The program should start by giving the user a choice as to which conversion they want to perform.
If a user selects 1, then the program prompts for the message address, as described above (dot-decimal notation representing the packets IP address followed by a forward slash and a subnet mask) and:
a) verifies the 4 int values that were entered with dots are within the appropriate range (0-255); otherwise, it keeps on prompting until the correct values were entered
b) verifies that the value entered for a subnet mask is within 0 32 range; otherwise, it keeps on prompting until the correct value is entered
c) displays the 32-bit unsigned int equivalent of the IP address in both unsigned decimal and binary
d) displays the subnet mask in unsigned decimal, binary, and in dot-decimal notation,
e) displays the network prefix (binary AND of IP and mask) in unsigned decimal, binary, and in dot-decimal
f) displays the host id (binary AND of IP and ones complement of the mask) in unsigned decimal, binary, and
dot-decimal
If a user selects 2, then the program prompts for the dot-decimal notation of the network prefix and the host and calculates the message address consisting of IP/subnet and displays them. Note that the IP component is the result of OR-ing the network prefix with the host. However, the calculation of the subnet mask is not straight forward since a subnet is not necessarily unique. Lets use the following algorithm: find the last 1 in the network prefix and use this location to calculate the number of leading 1s in your subnet.
Then the programs prompts the user, if they want to repeat the program (use lowercase r as the indicator) and repeats the process as required. This is a sample run of the program (bold and italics indicate user entries). You are to follow the format shown in this sample run:
What type of conversion do you want? Enter 1 for IP-address and subnet, 2 for the host and network prefix: 1
Enter the message address: 192.168.5.130/24 The IP-address is 3232236930 in decimal and 11000000 10101000 00000101 10000010 in binary
The subnet mask is 4294967040 in decimal and 11111111 11111111 11111111 00000000 in binary The subnet mask in dot-decimal is: 255.255.255.0
The network prefix is: 3232236800 in decimal and
11000000 10101000 00000101 00000000 in binary The network prefix in dot-decimal is 192.168.5.0
The host id is: 130 in decimal and 00000000 00000000 00000000 10000010 in binary The host id in dot-decimal is 0.0.0.130
Enter r to repeat, q to quit: r
-------------------------------------------------------------------------
What type of conversion do you want? Enter 1 for IP-address and subnet, 2 for the host and network prefix: 1
Enter the message address: 192.-168.5.130/24 Wrong address, try again: 256.168.5.130/24 Wrong address, try again: 255.168.5.130/33 Wrong address, try again: 255.168.257.130/24 Wrong address, try again: 255.168.257.130/24 Wrong address, try again: 73.150.2.210/9
The IP-address is 1234567890 in decimal and 01001001 10010110 00000010 11010010 in binary
The subnet mask is 4286578688 in decimal and 11111111 10000000 00000000 00000000 in binary The subnet mask in dot-decimal is: 255.128.0.0
The network prefix is: 1233125376 in decimal and 01001001 10000000 00000000 00000000 in binary The network prefix in dot-decimal is 73.128.0.0
The host id is: 1442514 in decimal and 00000000 00010110 00000010 11010010 in binary The host id in dot-decimal is 0.22.2.210
Enter r to repeat, q to quit: r
-------------------------------------------------------------------------
What type of conversion do you want? Enter 1 for IP-address and subnet, 2 for the host and network prefix: 2 Enter the host: 0.0.0.130 Enter the network prefix: 192.168.5.0
The message is: 192.168.5.130/24
Enter r to repeat, q to quit: q
Specs
Your program has to use the same choice values (1, 2, r, q ) and follow the same order of operations as shown in the sample run above, although your actual prompt wording could be different
You have to use bitwise operators in your program
You are only allowed to use basic primitive types, i.e. arrays and strings are NOT allowed
Your program is to be written as a single c file named pr1.c
Your program must compile in gcc gnu 90 programs that do not compile will receive a grade of 0
If you use a math library, then you will need to compile your program with the following command:
gcc -std=gnu90 pr1.c -lm
Your program has to follow basic stylistic features, such as proper indentation (use whitespaces, not tabs), whitespaces, meaningful variable names, etc.
Your program should include the following comments:
o Your name at the top
Test Plan
o Whether you tested your code on the cssgate server or Ubuntu 16.04 LTS Desktop 32-bit o Comments explaining your logic o If your program does not run exactly as shown above, explain at the top how to run your program
you will not receive full credit but at least you may receive some credit rather than none
A test plan in this class is a document that shows the test cases you performed on the final version of your program (values entered to test the outcomes). A test plan in this case should include full code coverage, should list the actual values you entered to test your program, and should be submitted as the following table, in a pdf document named test.pdf:
Extra Credit (15%)
Open-ended: extend the program by using additional IP conversions, for example given 32-bit decimal numbers for the IP and subnet calculate dot-decimal and binary notation explain in comments at the top of your code. Make sure your extra credit does not modify the original processing but rather adds to it
Step by Step Solution
There are 3 Steps involved in it
Step: 1
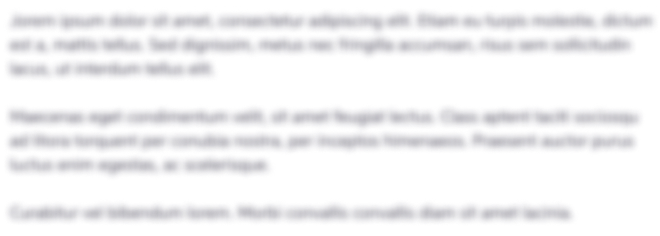
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started