Question
Objective This assessment item relates to the unit learning outcomes as in the unit descriptor. This assessment is designed to give students experience in analyzing
Objective This assessment item relates to the unit learning outcomes as in the unit descriptor. This assessment is designed to give students experience in analyzing a suitable dataset and creating different visualizations in dashboard and to improve student presentation skills relevant to the Unit of Study subject matter. Case Study: You are a data scientist hired by a retail company, "SmartMart," which operates a chain of grocery stores. SmartMart has been in the market for several years and has a significant customer base. However, the company is facing challenges in optimizing its operations and maximizing profits. As a data scientist, your task is to analyze the provided dataset and identify areas where data science techniques can be applied to create business value for SmartMart. Dataset: The dataset provided contains information on SmartMart's sales transactions over the past year. It includes data such as: Date and time of each transaction Customer ID Product ID Quantity sold Unit price Total transaction amount
Store ID Tasks: Apply appropriate statistical analysis techniques to extract valuable information from the dataset. This may include but is not limited to: a. Descriptive statistics b. Correlation analysis c. Hypothesis testing d. Time-series analysis Identify key findings and insights from your analysis that can help SmartMart make data-driven decisions to optimize its operations and increase profitability. Present your analysis results in a clear and concise manner, including visualizations and explanations where necessary. Provide recommendations on specific strategies or actions that SmartMart can take based on your analysis. Deliverables: 1. Written report documenting your analysis process, findings, and recommendations containing Python code/scripts used for data analysis, along with comments explaining the code logic and methodology and also Visualizations (e.g., plots, charts) supporting your analysis and findings.
Note: Please provide a single report that includes screenshots of Python code along with corresponding results, as well as screenshots of visualizations that supports your analysis.
Dataset: Use the below program to generate dataset with 1000 rows and following 7 columns. Customer ID Product ID Quantity sold Unit price Total transaction amount Store ID import pandas as pd import numpy as np import random
from datetime import datetime, timedelta # Generate 1000 random dates and times within a specific range start_date = datetime(2023, 1, 1) end_date = datetime(2023, 12, 31) date_times = [start_date + timedelta(seconds=random.randint(0, int((end_date - start_date).total_seconds()))) for _ in range(1000)] # Generate random customer IDs customer_ids = ['C' + str(i).zfill(4) for i in range(1, 1001)] # Generate random product IDs product_ids = ['P' + str(i).zfill(3) for i in range(1, 101)] # Generate random quantities sold quantities_sold = np.random.randint(1, 10, size=1000) # Generate random unit prices unit_prices = np.random.uniform(1, 100, size=1000) # Calculate total transaction amounts total_transaction_amounts = quantities_sold * unit_prices # Generate random store IDs store_ids = ['S' + str(i).zfill(3) for i in range(1, 11)] # Randomly assign store IDs to transactions store_ids = [random.choice(store_ids) for _ in range(1000)] # Create DataFrame data = { 'Date & Time': date_times, 'Customer ID': random.choices(customer_ids, k=1000), 'Product ID': random.choices(product_ids, k=1000), 'Quantity Sold': quantities_sold, 'Unit Price': unit_prices, 'Total Transaction Amount': total_transaction_amounts,
'Store ID': store_ids } df = pd.DataFrame(data) # Convert Date & Time column to datetime format df['Date & Time'] = pd.to_datetime(df['Date & Time']) # Sort DataFrame by Date & Time df = df.sort_values(by='Date & Time') # Reset index df.reset_index(drop=True, inplace=True) # Print DataFrame print(df)
Assessment criteria
1. Analysis Process and Methodology
2. Findings and Insights
3. Presentation and Clarity
4. Python Code/Script
5. Recommendations
Step by Step Solution
There are 3 Steps involved in it
Step: 1
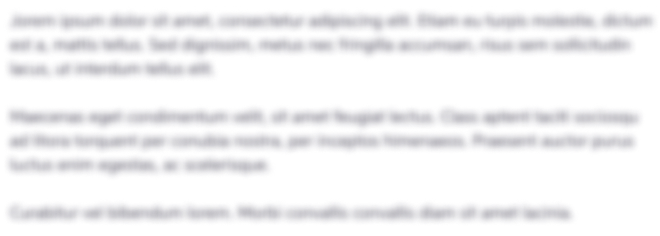
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started